Dart this Keyword
The ' this ' keyword, similar to that in Java and C#, refers to an object of the class using it. It points to the current object of the class, methods, or constructor.
To understand the use case of the ' this ' keyword, let us assume if the class attributes and parameter names in a program are the same. This arises ambiguity, which can be resolved using this keyword. The ' this ' keyword prefixes the class attributes. It can also be passed as a parameter in the member function or constructors or can be used to call them ( of the current class ).
Example 1 :
Consider the following examples in Dart that explain the concept of the 'this' keyword.
// Definition of the class ' employee '
class employee
{
// declaration of data members ‘ emp_id ’ and ‘ emp_name ’
var emp_id ;
var emp_name ;
// definition of constructor employee( )
employee( var emp_id, var emp_name )
{
/* accessing the data members of the class
using ' this ' keyword */
this.emp_id = emp_id ;
this.emp_name = emp_name ;
print( " This is an example of ' this ' keyword : " ) ;
// printing the value of emp_id
print( " The employee id is : " ) ;
print( emp_id ) ;
// printing the value of emp_name
print( " The name of the employee is : " ) ;
print( emp_name ) ;
}
}
void main( )
{
// passing arguments to the constructor
employee e1 = new employee( 1 , ' Yukta ' ) ;
}
Output :
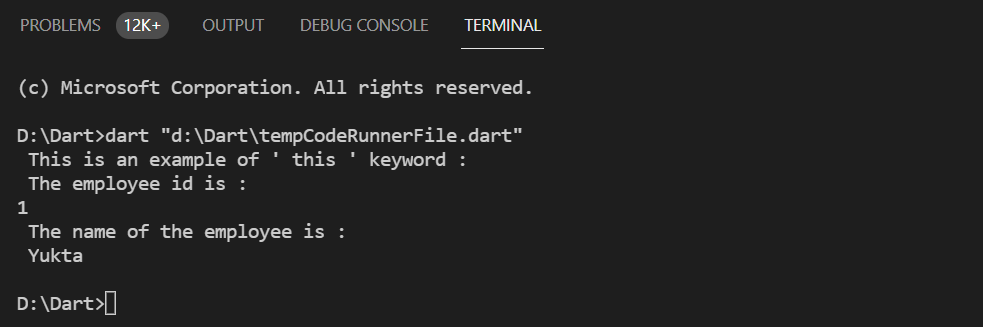
Explanation :
In the above program, we created a class called ‘ employee ’ that has two attributes, ' emp_id ' and ' emp_name '. We assigned the values to these attributes by creating a constructor and passing parameters the same as the class attributes name. The ' this ' pointer refers to the attributes and assigning the value.
The Dart compiler gets confused if there are a number of the parameters with the same name. This is the case of ambiguity. The ' this ' keyword is used to refer to the current class object to resolve this ambiguity.
Points to Remember
- The ' this ' keyword is used to point to the object of the class invoking it.
- It can be used to refer to the present class variables.
- We can instantiate or invoke the current class constructor using this keyword.
- We can pass the ' this ' keyword as a parameter in the constructor call.
- We can pass the ' this ' keyword as a parameter in the method call.
- It removes the ambiguity or naming conflict in the constructor or method of our instance/object.
- It can be used to return the current class instance.
Example 2 :
Consider another example to have a better understanding of ' this ' keyword :
Case 1 : When the name of the local variable is the same as that of the name of the member
// definition of the class called ‘ Test ’
class Test
{
// data member ' x ' declaration
var x ;
// defintion of member function setX( ) to set the value of x
void setX ( var x )
{
// The ' this ' pointer is used to retrieve the object's x
// hidden by the local variable ' x '
this.x = x ;
}
/* definition of member function display( ) to display the value
of variable x */
void display( )
{
print( " The value of x is : " ) ;
print( x ) ;
}
}
int main( )
{
// declaring an object named ' t '
var t = new Test( ) ;
// local variable ' x ' ( same name as that of data member ' x ' of
// class ' Test ')
int x = 20 ;
// accessing member functions of the class using object
t.setX( x ) ;
t.display( ) ;
return 0 ;
}
Output :
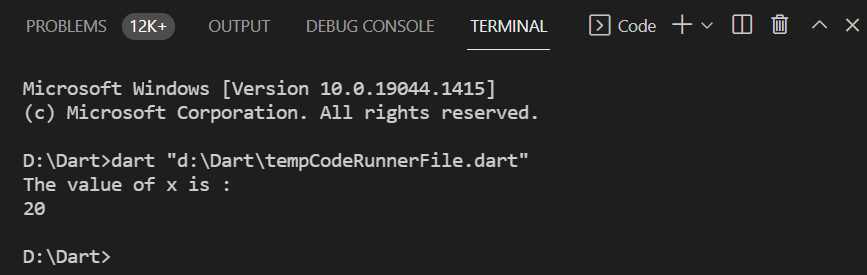
Case 2: To return a reference to the calling object
// definition of class ' Test '
class Test
{
// data member declaration
var x ;
var y ;
// definition of member function setX( ) with a return
// type of class and a single argument a of integer type
Test setX( int a )
{
x = a ;
return this ;
}
// definition of member function setY( ) with a return
// type of class and a single argument a of integer type
Test setY( int b )
{
y = b ;
return this ;
}
// definition of member function display( ) to display the values of x and y
void display( )
{
print( " The value of x is : " ) ;
print( x ) ;
print( " The value of y is : " ) ;
print( y ) ;
}
}
int main( )
{
// creating an object of the class ‘ t ’
var t = new Test( ) ;
// accessing the member functions of the class using
// the object of the class
t.setX( 10 ).setY( 20 ) ;
t.display( ) ;
return 0 ;
}
Output :
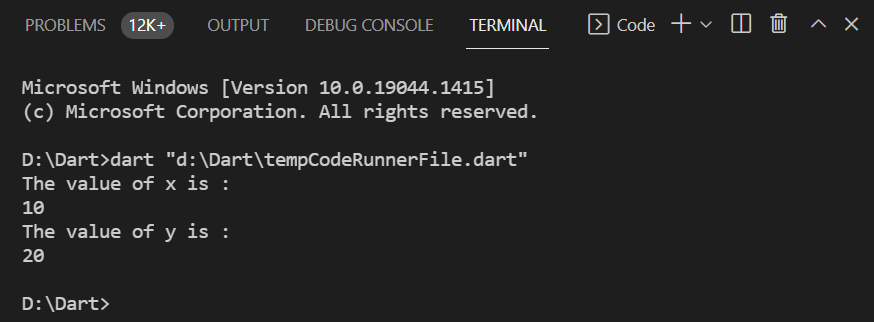
Local Variables :
Local variables are the variables that are local to the method, constructor, or block in which they are defined. The scope of this variable is only inside these identifiers. These local variables can not be used outside the function, constructor, or block in which they are defined.
Class Variables :
Class variables are the variables that are declared inside the class but outside the constructor, method, or block. These are also known as the static data members that are declared using the 'static' keyword. Some points to remember here are that :
Only a single copy of the static class variable is declared.
All the objects share this single copy of the variable only.
Instance Variables :
All the variables are other than class variables are known as is instance variables. It can be said that the variables declared without the 'static' keyword are instance variables. These variables are object-specific, which means for each object, they have a separate value, and they can be accessed using the instance of that class.
Difference Between Class Variable and Instance Variable :
Here are a few points of difference between class variable and instance variable
Sr. | Class Variables | Instance Variables |
1. | The class variables are declared using the ' static ' keyword in a class. They are not declared in the function or constructor. | The instance variables are the variables other than the static variables. These variables are declared in a class without using the ' static ' keyword. |
2. | The class variables can be accessed using the class name. Syntax : ClassName.variableName | The instance variables can be accessed using the object of that class. Syntax : ObjectRefernce.variableName |
3 | All the class variables are common to all the objects of that class as they share only one copy of this static variable. | The instance variables are not common to all instances of the class. Each object will preserve its own copy of the instance variables. |
4 | These are created when the program is started and destroyed when the program is terminated. The scope of the static variables is throughout the lifetime of the program. | The instance variables are created when an object of the particular class is created using the new( ) keyword and destroyed when the object is destroyed. |