Dart Variables
Variables are used to store the value of a particular data type referred to in the whole program by a name or identifier. They refer to a memory location as allocated by the compiler and act as a container of values in a program.
The variable declaration refers to declaring a variable of a certain data type to hold the value of that type. On compilation, when the compiler comes across a variable declaration statement, it allocates some memory space to that variable depending upon the data type, compiler, and processor.
Variable is declared as follows:
data type variable_name
and initialized as
data type variable_name = value;
For example,
int a = 5;
In Dart, variables store references to the data type, with the value assigned to it.
Rules of declaring a Variable:
There are a few rules to write identifiers in a Dart code which are as follows:
- The variables cannot begin with digits. However, they may contain a digit at the end or in-between the letters of an identifier.
- Dart is a case-sensitive language, and so are the variables.
For example, Area and area are two different identifiers. - Blank spaces, mathematical symbols, runes, and Unicode characters are not allowed in the variable declaration.
- Keywords cannot be used as the variables name, which are the reserved words of a programming language, and they must be unique.
- Variables can not include any special character except underscore ‘_’ or dollar ‘$’.
- The first character can only be an alphabet (lower or upper case) or permissible special characters, dollar ‘$’ and underscore ‘_’. But two successive underscores ‘__’ are not allowed.
Variable Declaration
In Dart programming language, the ‘var’ keyword is used to declare a variable when we do not explicitly mention the variable's data type.
In Dart, it is optional to mention the data type of the variable. The compiler automatically understands the data type of the variable by scanning the value assigned to the variable. Due to this reason, Dart is also known as an infer type language.
Syntax:
var variable_name;
Example:
var remainder;
Type System
In Dart, we can explicitly mention the data type of the variable while declaring them. In such a case, the 'var 'keyword is not required.
Syntax:
data type variable_name;
This statement clearly defines the type of value a variable can hold.
Example:
double remainder;
Here, double is a data type to store floating-point numbers.
Multiple Variables Declaration
Dart provides the feature of declaring multiple variables with values of the same type in a single line by separating each variable by a comma.
Syntax:
data_type var1, var2, var3;
data_type var1 = val1, var2 = val3, var3 = val9;
Here var refers to the variable name, and val refers to the value.
Example:
int a, b, c;
int a = 5, b = 0, c = 9;
In the first example, no value is initialized to any variable. In such a case Dart compiler assigns a default value to the variables, which is null.
Note that the default value of numeric variables is also null.
For example
Consider the following code where only the c variable is initialized with value 5.
void main( )
{
var a, b, c = 5;
print( ' \nValue of a is : $a ' ) ;
print( ' \nValue of b is : $b ' ) ;
print( ' \nValue of c is : $c ' ) ;
}
Output :
Value of a is : null
Value of b is : null
Value of c is : 5
Since only c was initialized with 5, the same value got printed, while for a and b, the compiler initializes them with a default value, null.
final and const Keywords
‘final’ and ‘const’ keywords are used to initialize the variables with a constant value that cannot be changed throughout the program.
‘final’ can be set only once as they are assigned the value at the compile-time debarring any change in the future. At the same time, ‘const’ is a compile-time constant, and it is implicitly equivalent to final.
Syntax:
If no type is mentioned then, they are used in place of var as follows,
final var_name = value;
In the case of type annotation, they are used before the data type as follows,
final data_type var_name = value;
For example
Consider the following code
void main( )
{
var a = 5; // normal variable declaration
final b = 6; // variable declaration using final keyword
print( 'Value of a is : $a and Value of b is $b' );
a = 6; // changing the value of normal variable
b = 7; // changing the value of variable with final keyword
print( 'Value of a is : $a and Value of b is $b' );
}
The following code gives an error at line number 9, stating, "The final variable 'b' can only be set once."
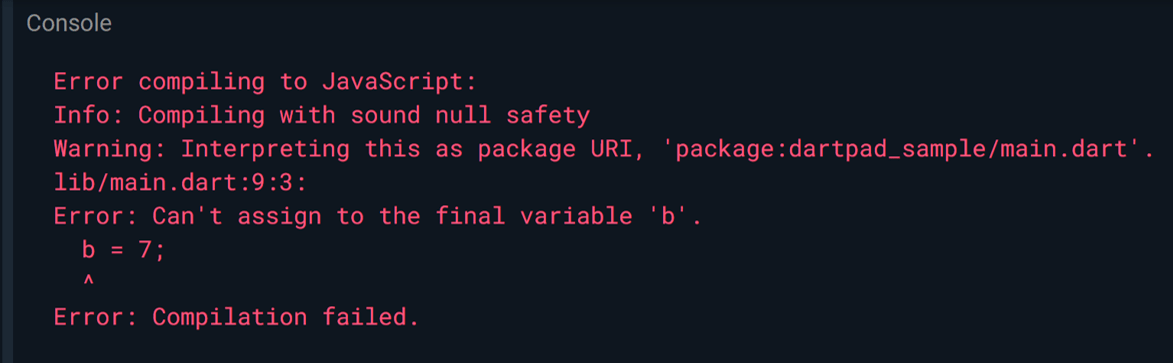
The ‘const’ keyword is used to create compile-time constants. If the ‘const’ variable is to be declared in a class, mark it static const. The ‘const’ keyword is used not only to declare the variables but also to create constant values and constructors that create constant values.
Syntax:
const var_name = value;
Example:
const rem = 198; // rem variable assigned with constant value 198
var a = const [ ]; // creates a constant value
const a = [ ]; // equivalent to the above declaration
The value of the const variable cannot be changed throughout the program.
Example,
Consider the following code:
void main( )
{
const rem = 6;
rem = 5;
}
This code gives an error on line number 4, stating, "Constant variables can't be assigned a value."
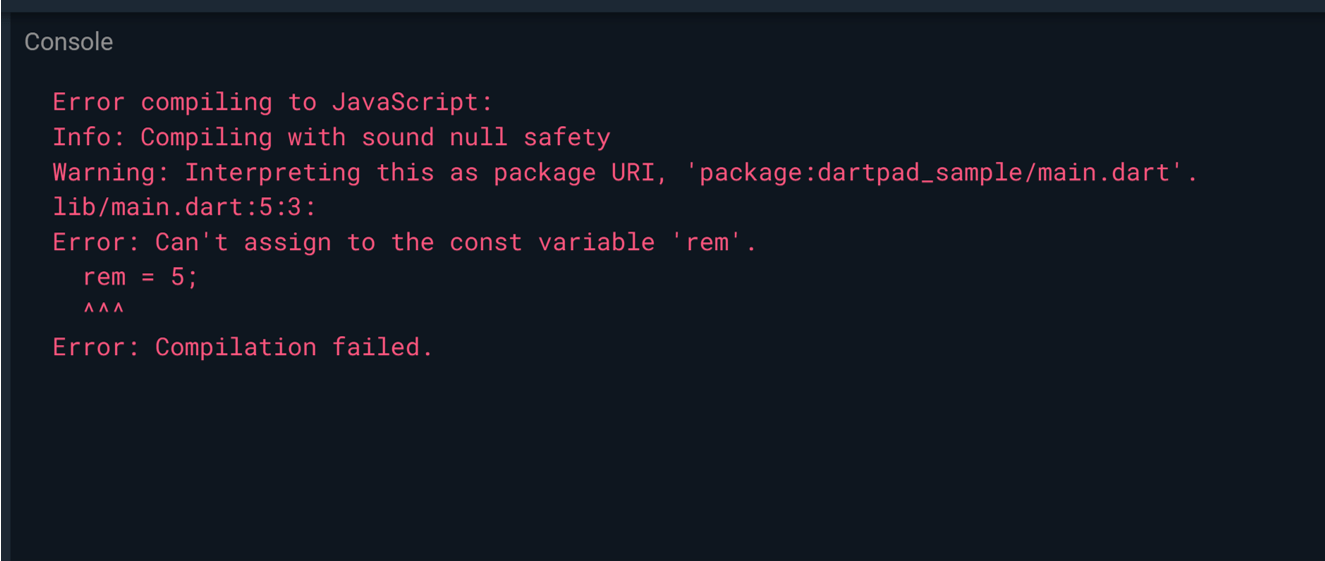
Late Variables
The newer version of Dart, Dart 2.12, gave the concept of late modifiers, which can be used in the following cases:
- Declaring a variable that can't be null and is initialized after the declaration.
- Initializing a variable lazily.
Dart's control flow analysis detects if a non-nullable variable is set to a non-null value before using it. But at times, this analysis fails. If we know that a variable is set before it is used in the program, but Dart doesn’t agree. This can be resolved by making the variable as late. If we fail to initialize a late variable, a runtime error occurs when the variable is used.