main function in Dart
The main( ) function is a predefined method in Dart that is also known as the entry-point of the program. The compiler begins the execution only when it comes across the main( ) function. It is the most important function of the Dart programming language. This function is responsible for the execution of all the library functions, user-defined statements and user-defined methods.
All the data variables and functions for the execution are defined inside the main( ) function only. An important point to remember here is that the main( ) function can only be used once in the program. The main( ) function may or may not return any value. Similarly, it may or may not have arguments as the parameters.
Given below is the syntax of the main( ) function :
void main( )
{
// Body of the main( ) function which can contain variable declaration and method definition
}
Also, the main( ) function can have optional parameters as List< String > that act as arguments to the function. These are also known as command-line arguments.
Consider an example that explains the concept of main( ) function :
Example 1:
The following example is a basic example of how the main function is the entry point of a Dart program.
main( )
{
print( " This is the main( ) function ! " ) ;
}
Output :
This is the main( ) function !
main( ) function with return value
We can make the main( ) function return some value also. At times the function returns some value based on the computation inside the function. The return statement comprises of the ‘ return ’ keyword and the variable that holds the value to be returned to the function call statement.
In case there is no return statement, then the function returns null. It is completely optional and as per the programmer whether to add the return statement or not. An important point to remember is that a function can only have one return statement.
Given below is the syntax :
return_type main( )
{
// body of the function
return value ;
}
In the above syntax, the function contains a return type as well which can be any valid data type of Dart language. The value to be returned must match with the return type of the function. The “ return value ; ” statement refers to the returning of the value held by the variable ‘ value ’.
Consider the example that explains the concept of return value in main( ) function :
Example 2 -
int main( )
{
print( " This is the function returning an integer value ! " ) ;
return 0 ;
}
Output :
This is the function returning an integer value !
Example 3 :
Consider another example wherein a function returning some value is contained inside the main( ) function :
void main( )
{
int add( int a, int b )
{
int sum = a + b ;
return sum ;
}
print( " The sum of these two numbers is : ${ add ( 40,50 ) } " ) ;
}
Output :
The sum of these two numbers is : 90
main( ) function with arguments
The main( ) function can accept arguments also as its parameters but they have to be of type List < String >. In this case, the program must be executed through command prompt to pass arguments to the main( ).
Given below is the syntax :
main( List < String > arguments )
{
// printing the arguments along with length
print( arguments.length ) ;
print( arguments ) ;
}
Consider another example that explains the above concept :
Example 4 :
void main( List< String > arguments )
{
print( " The length of arguments is : " ) ;
print( arguments.length ) ;
print( " List of arguments is : " ) ;
print( arguments ) ;
}
Output without using command prompt :
The length of arguments is :
0
List of arguments is :
[ ]
Output using command prompt :
To pass arguments to the main function, use the following command :
dart program_name.dart [ Argument 1 ], [ Argument 2 ], . . . . . . . . . . [ Argument n ]
Write this command in the command prompt as follows :
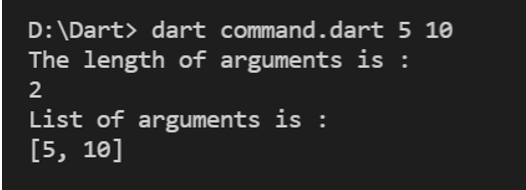
The length of arguments is :
2
List of arguments is :
[ 5, 10 ]