Dart Switch Case Statement
In the case of if-else statements, we prefer not to use the if-else ladder when there are many test conditions to be evaluated. In such a situation, it is preferable to use the Dart switch case statement, which is the simplified form of the nested if-else statement.
Here, the single value of the variable is compared with the multiple cases. If a matching case is found, it executes a block of statements associated with that particular case, and the flow breaks out of the switch loop with the help of the break statement.
The break statement is very important in the dart switch case loop. If we somehow forget to write the break statement, the output corresponding to each statement after the case matches the value is printed to the console.
Let us understand its syntax :
switch( expression )
{
case value - 1 : //statement( s )
Block - 1 ;
break ;
case value - 2 : //statement( s )
Block - 1 ;
break ;
case value - 3 : //statement( s )
Block - 1 ;
break ;
case value - N : //statement( s )
Block - 1 ;
break ;
default : // statement( s ) ;
}
In this switch case statement syntax, the expression can be an integer or character. The values 1, 2, n is called the case labels. They identify each case of the string, and the value of the expression is checked against these case labels only. They must always be terminated by a colon ':'. It is mandatory to have unique case labels; redundant labels cause ambiguity for the compiler. It creates confusion that a particular value should be matched against one of the cases.
A block is multiple lines of code associated with a particular case label. After evaluating the test expression, the resultant value is compared with all the cases defined in the switch case. Whichever case label matches with the resultant value. The compiler executes the corresponding block. The break statement is used to truncate the flow out of the switch statement as soon as the value is matched with the case label. If we do not write the break statement after each case, it will execute all the cases until the program end is reached.
At times, the resultant value does not match with any of the case labels; the statement corresponding to the default case is executed in such a situation. However, writing the default statement is entirely optional in Dart.
Consider the following example to gain a better perspective on switch-case statements
Program
import 'dart:io' ;
void main( )
{
// accept the marks from the user
print( ' Enter the Marks ( 100 / 80 / 60 / 40 / 20 / 0 ) : ' ) ;
int? n = int.parse(stdin.readLineSync( ) ! ) ;
// switch case statement
switch( n )
{
case 100 : print( ' Grades are : A+ ' ) ;
break ;
case 80 : print( ' Grades are : B+ ' ) ;
break ;
case 60 : print( ' Grades are : C+ ' ) ;
break ;
case 40 : print( ' Grades are : D+ ' ) ;
break ;
case 20 : print( ' Grades are : E+ ' ) ;
break ;
case 0 : print( ' Fail ' ) ;
break ;
}
}
Output :
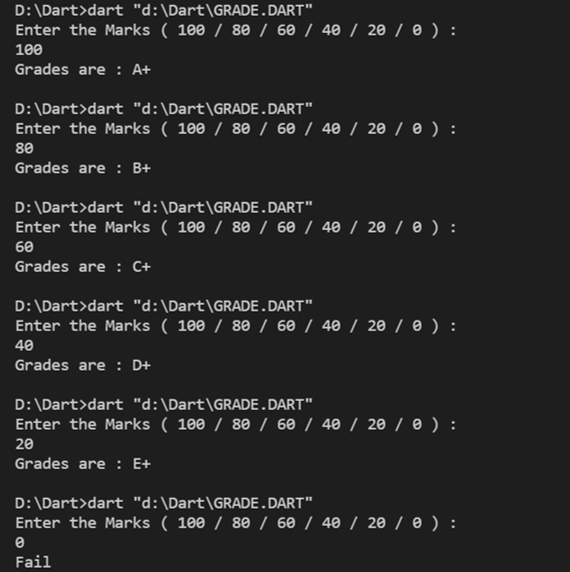
Explanation -
The marks are taken from the user in the above program and compared with different case labels. Whichever case matches, the output corresponding to that case is executed.
Consider the following code in Dart that is a simple calculator simulation.
Program
import 'dart:io';
void main( ) {
// printing the menu for the menu driven program
print( " \n MENU \n Select the choice you want to perform : \n 1. ADD \n 2. SUBTRACT \n 3. MULTIPLY \n 4. DIVIDE \n 5. EXIT \n Choice you want to enter : " ) ;
int? n = int.parse( stdin.readLineSync( ) ! ) ;
// accept the value of the first variable from the user
print( " \n Enter the value for x : " ) ;
int? x = int.parse( stdin.readLineSync( ) ! ) ;
// accept the value of the second variable from the user
print( " \n Enter the value for y : " ) ;
int? y = int.parse( stdin.readLineSync( ) ! ) ;
// switch case
switch ( n ) {
// case 1 for addition of two numbers
case 1 :
int s = x + y ;
print( ' \n Sum of the two numbers is : ' ) ;
print( s ) ;
break ;
// case 2 for difference of two numbers
case 2 :
int d = x – y ;
print( ' \n Difference of the two numbers is : ' ) ;
print( d ) ;
break ;
// case 3 for the multiplication of two numbers
case 3 :
int m = x * y ;
print( ' \n Product of the two numbers is : ' ) ;
print( m ) ;
break ;
// case 4 for the division of two numbers
case 4 :
int div = x ~/ y ;
print( ' \n Quotient of the two numbers is : ' ) ;
print( div ) ;
break ;
// default case if the value of the expression matches no case
default :
print( " Wrong choice " ) ;
}
}
Output :
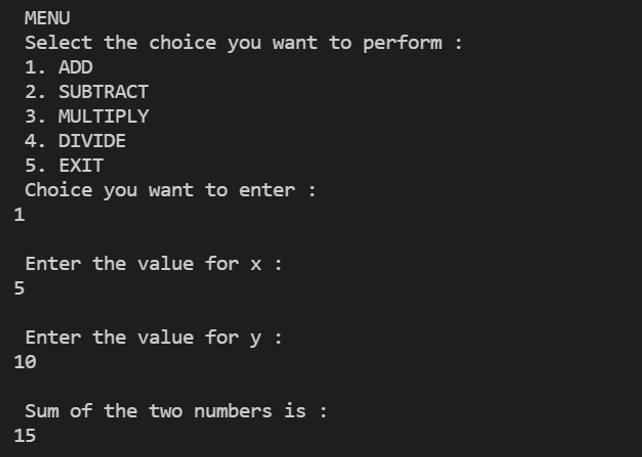
Explanation :
In the above code, we accept the value of the variable for the test expression from the user, and the compiler checks it with all the case labels. Case Label 1 corresponds to addition operation, Label 2 to subtraction operation, Label 3 to multiplication operation, and Label 4 to division operation. When the value of the test expression matches with the case label, the values of x and y are taken from the user, and the corresponding operation is performed.
Advantages of Switch case
The switch case statement is a simplified version of the nested if-else statement. The if-else statement unnecessarily increases the lines of code in the program and creates confusion for the reader to understand the program. Moreover, redundancy of cases occurs at times in the nested if-else statement. The switch case reduces the ambiguity of the program and enhances its readability and understandability.