Dart Miscellaneous types
Some Other Data types in Dart
Apart from the data types studied so far, we have three other data types also which are as follows:
- Never
- Dynamic
- Void
Let us understand each one in detail,
Never data type in Dart
Dart 2.9 offers a new bottom data type known as ‘Never’. Its declaration is there in the core libraries and can be used as a type annotation. It can act as a return type of functions. It has no values and using Never means that the expression can never successfully finish evaluating. It will either throw an exception or abort the execution of the code.
Remember that the static type of a throw expression is Never.
Example : Never fun( ) { }
Dynamic data type in Dart
This data type enables us to create variables that are dynamic in nature. By dynamic means their data type can be changed at the runtime.
For example,
dynamic msg = ‘ Hello World ! ’ ;
If instead of this, we have an initialization like this :
string msg = ‘ Hello World ! ’ ;
The compiler interprets that the variable ‘ msg ’ is of string type and will only and always hold string values. Even if we ever try to change its data type or to make it hold the integer value for example, we can’t do that.
Program
void main( )
{
// initializing the string name
String name = " This is dynamic data type tutorial ! " ;
// printing the string name
print( name ) ;
// initializing the string type name with integer
name = 8 ;
}
Output
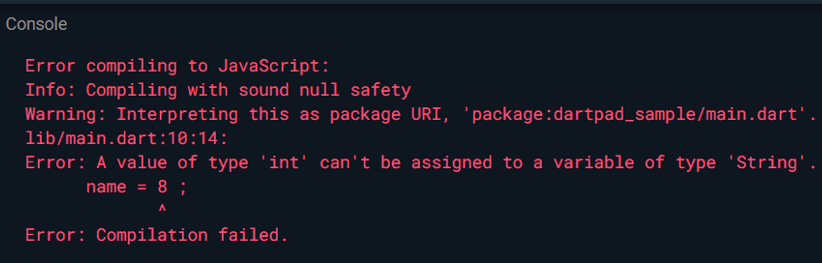
As you can see, the compiler threw a compilation error when we tried to assign the string variable ‘name’ with the integer value.
Consider another example where we use dynamic data type and tries to change the value of the variable:
Program
void main( )
{
// initializing the string name
dynamic name = " This is dynamic data type tutorial ! " ;
// printing the string name
print( name ) ;
// initializing the string type name with integer
name = 55 ;
// printing the integer value of the dynamic variable name
print( name ) ;
}
Output
This is dynamic data type tutorial !
55
void data type in Dart
void data type in programming languages usually means that they do not return anything. The return type in the Dart language only specifies what the DartAnlyzer warns us about.
If the return type of the function is void, it can return the following three
things :
1 ) Return null
2 ) Return dynamic variable
3 ) Return void function
Remember that we cannot use these returned values.
Constructors
- void ( )
Properties
1. hashCode
Returns the hash code for a numerical value, and it returns the same value for int and doubles when the value provided is the same.
Implementation = external int get hashchode ;
2. runtimeType
Represents the type of runtime an object has.
Implementation = Type get runtimeType ;
Methods
1. noSuchmethod ( )
This method is called when the non-existing method is accessed.
Implementation =
dynamic noSuchMethod (
Invocation invocation
)
2. toString ( )
Converts a given number into an equivalent shortest string and returns the same.
Implementation = external String toString( ) ;