Dart - extends, with and implements Keywords
The application development in Dart programming language using the Flutter framework, regularly experience different usage of the implements, extends and with keywords. Dart has full support for inheritance that is one class can inherit another class. Dart can create a new class from an existing class. To implement such features in Dart we make use of keywords to do so. In this article, we will look at 3 keywords used for the same purpose and compare them, namely:
- extends
- with
- implements
Let’s look into them one at a time.
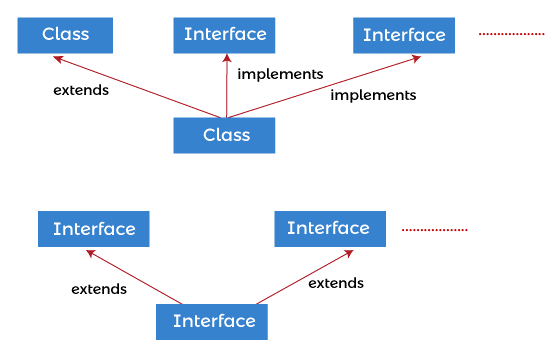
extends
In Dart, an extended keyword is often used to change class behaviour using Inheritance. The ability of a class to acquire properties and features in another category is called Inheritance. It is the ability of a program to create a new class from an existing class. In simple terms, we can say that we use extends to form a subclass, and super referring to the superclass. A class whose properties the child class inherits is called a Parent Class. The parent class is also known as the base class or super class.
The class that inherits properties from another class is called child class. Child class is also known as derived class, heir class, or subclass.
The extends keyword is the typical OOP class inheritance. If a class ‘A’ extends another class ‘ B ’ then all the properties, variables, and methods implemented in the parent class ‘ A ’ are also available in the child class ‘ B ’. Moreover, we can also override the functions of the base class in the derived class.
We use the keyword ‘extends’ if you want to create a more specific version of a class. For instance, if a child class ‘Car’ extends from the base class ‘ Vehicle ’, then all the properties, variables and functions defined in the ‘ Car ’ class will be available in the ‘ Vehicle ’ class.
Example :
Let us consider an example of implementation of the ‘extends’ keyword. There is no need to override the definition of the inherited class and can use the existing definition in the child class.
// This is the definition of the parent class ' teacher’
class teacher
{
// function member output( )
void output( )
{
print( " All classes have a teacher ! " ) ;
}
}
/* This is the definition of the base class ' student ' that inherits
* the base class ' teacher ' */
class student extends teacher
{
// function member output( )
void display( )
{
print( " Every student is taught by some teacher ! " ) ;
}
}
void main( )
{
/* creating an object of the derived class ' student '. This object
* can access the mmebers of both the classes ' student ' and ' teacher '
* because ' student ' class inherits ' teacher ' class. */
var s1 = new student( ) ;
// accessing member function output( ) of ' teacher ' class
s1.output( ) ;
// accessing member function display( ) of ' student ' class
s1.display( ) ;
}
Output :
All classes have a teacher!
Every student is taught by some teacher!
implements
An interface in Dart refers to the syntax or blueprint that any class must adhere to. It basically defines the array of methods available on the object. It provides the user with the blueprint of the class that any class should follow if it interfaces with that class. If a class inherits another class, it should override ( re-define ) the functions present inside that interfaced class in its own way as per the subject. In Dart, there isn't a specific or direct way of creating the interfaces. To implement them, we use the ' implement ' keyword. By default, every class is an interface in itself containing all the interface members and the members of any other interfaces that it implements.
Every class implicitly defines an interface containing all the instance members of the class and of any interfaces it implements. If you want to create a class A that supports class B’s API without inheriting B’s implementation, class A should implement the B interface. A class implements one or more interfaces by declaring them in an implements clause and then providing the APIs required by the interfaces.
Example 2:
// definition of the interface class ' Interface1'
class Interface1
{
// definition of the display1( ) function of the ' Interface1 ' class
void display1 ( )
{
print( " This is the function of the interface class ' Interface1 '. " ) ; }
}
// definition of the interface class ' Interface2 '
class Interface2
{
// definition of the display2( ) function of the ' Interface2 ' class
void display2( )
{
print( " This is the function of the interface class ' Interface2 '. " ) ; }
}
// definition of the interface class ' Interface3’
class Interface3
{
// definition of the display3( ) function of the ' Interface2 ' class
void display3( )
{
print( " This is the function of the interface class ' Interface3 '. " ) ;
}
}
// definition of the subclass implementing the above three interface classes
class subclass implements Interface1, Interface2, Interface3
{
void display1( )
{
print(" Overriding the display1( ) function in the subclass. " ) ;
}
void display2( )
{
print( " Overriding the display2( ) function in the subclass. " ) ;
}
void display3( )
{
print ( " Overriding the display3( ) function in the subclass. " ) ;
}
}
void main( )
{
// creating the object of the subclass
subclass s1 = new subclass( ) ;
// calling overridden methods of the interface classes in the subclass
s1.display1( ) ;
s1.display2( ) ;
s1.display3( ) ;
}
Output :
Overriding the display1( ) function in the subclass.
Overriding the display2( ) function in the subclass.
Overriding the display3( ) function in the subclass.
with
Mixins are a way to reuse class methods in multiple class hierarchies. Mixins can be understood as abstract classes that are used to reuse methods in different classes with the same functions / attributes. Mixins are a way of thinking and reusing the working the family of operations and state. It is similar to the reuse you get from extending a class, but not multiple inheritances. There is still only one superclass.
The ' with ' keyword is used to install Mixins. Mixin is a different type of structure, which can only be used with a keyword ' with '.
Mixin is a different type of structure, which can only be used with a keyword ' with '. In Dart, the class can play the role of mixin if the class does not have a constructor. It is also important to note that mixin does not impose a limit type and imposes restrictions on use in class methods.
Example 3:
// mixin with name ' teacher '
mixin teacher {
void func( ) {
print( ' This is the function of the mixin teacher ' ) ;
}
}
// mixin with name ' student '
mixin student {
void func2( ){
print( 125 ) ;
}
}
// mixin type used with keyword
class Principal with teacher, student{
@override
void func( ) {
print( ' We can override function inside this class if needed ' ) ;
}
}
void main( ) {
var princi = Principal( ) ;
princi.func( ) ;
princi.func2( ) ;
}
Output :
We can override function inside this class if needed
125