MATLAB Control Statements
What are control statements?
The name itself suggests that something is being controlled, these control statements decide or alter the flow of execution ina program. In program, we often need to modify or alter few lines of code, these control statement helps to perform this type of task.
Four categories of control statements are sequence control statements, decision control statements, repetition control statements (looping statements), and case control statements.
MATLAB control statements.
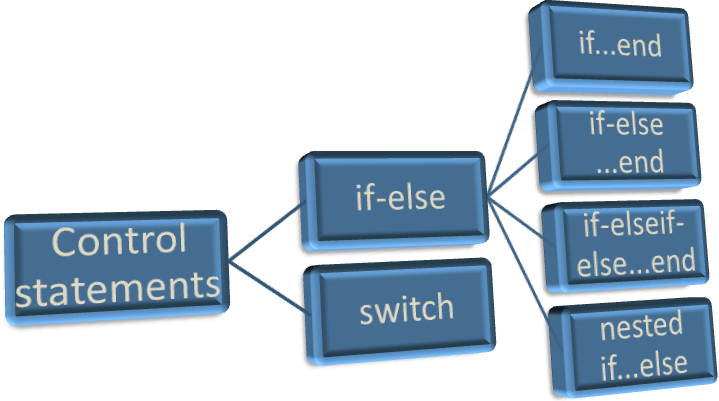
If…end
If…end statement helps to execute a block of code by satisfying a condition. The condition is defined by user at the time of coding. This if… block of code is closed with the keyword “end”. We can also use elseif, else if further conditioning is required.
Let us look at the syntax and few examples below.
Syntax:
if exp1 set of statements end
Flowchart:
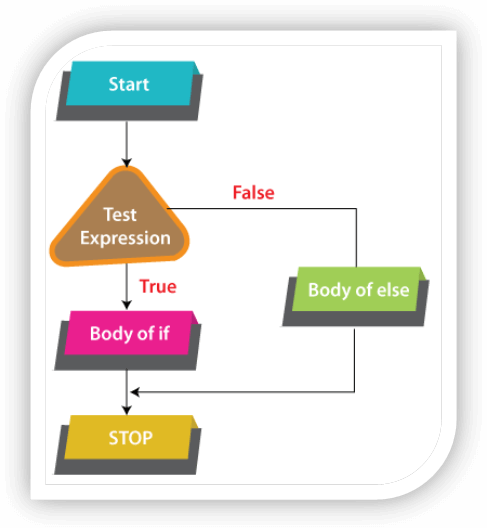
Example:
j = 10; if j ~= 0 disp('Nonzero value') end
Output:
Nonzero value
If-else…end statement
This control statement executes at most one no. of commands on which condition evaluates true, and a default block is evaluated if conditions are not met.
The control flows in this direction à if clauseà1stelseif clause à 2ndelseif clause àelse clause.
Let us look at the syntax and few examples below.
Syntax:
if exp1 statements block else Statements block end
Flowchart:
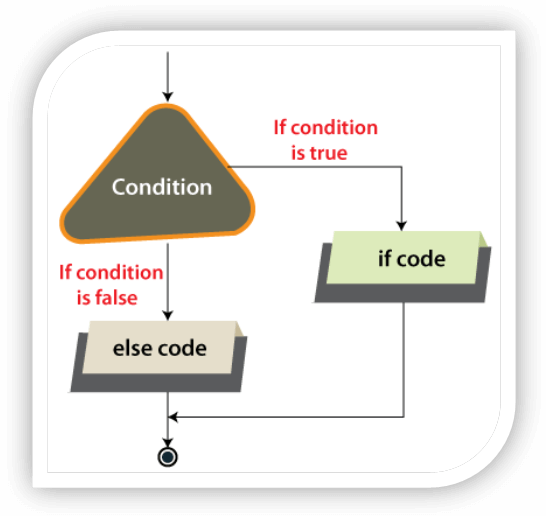
Example:
Ifsize(d)
is equal tosize(f)
, arrays are concatenated otherwise an empty array is returned as output. d = ones(2,3); f = rand(3,4,5); ifisequal(size(d),size(f)) k = [d; f]; else disp('d and f are unequal.') k = []; end
Output:
d and f are not the same size.
If-elseif-else….end
This control statement is the extended version of previous control statement, here we keep on adding elseif clause according to our requirement.
Let us look at the syntax and few examples below.
Syntax:
If exp1 Statements block elseif exp2 Statements block else Statements block End
Flowchart:
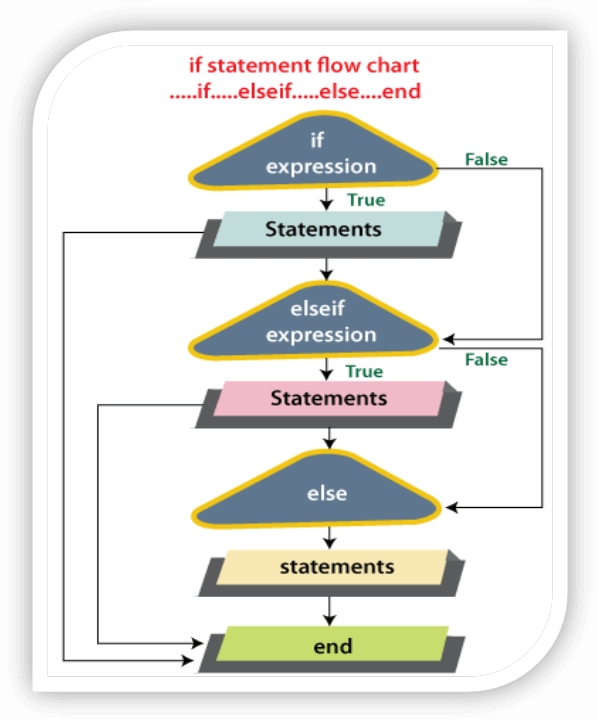
Example:
x = 20; min = 2; max = 6; if (x >= min) && (x <= max) disp('within specified range') elseif (x > max) disp('exceeds maximum ') else disp(' is below minimum') end
output:
exceeds maximum
Nested if-else
Nested if-else is used when we decide to condition set of statements inside statements, but each if clause requires the end keyword. Let us look at the syntax and few examples below.
Syntax:
if exp1 Statements block if expression Statements block else Statements block end elseif exp2 Statements block if expression Statements block end else Statements block end
At first expression-1 is checked, the following set of statements are executed then control flows to if-else block. Elseif expression2 is further checked weather the condition gets satisfied or not. If none of the above condition is satisfied then at last else-block is executed with the end keyword.
Flowchart:
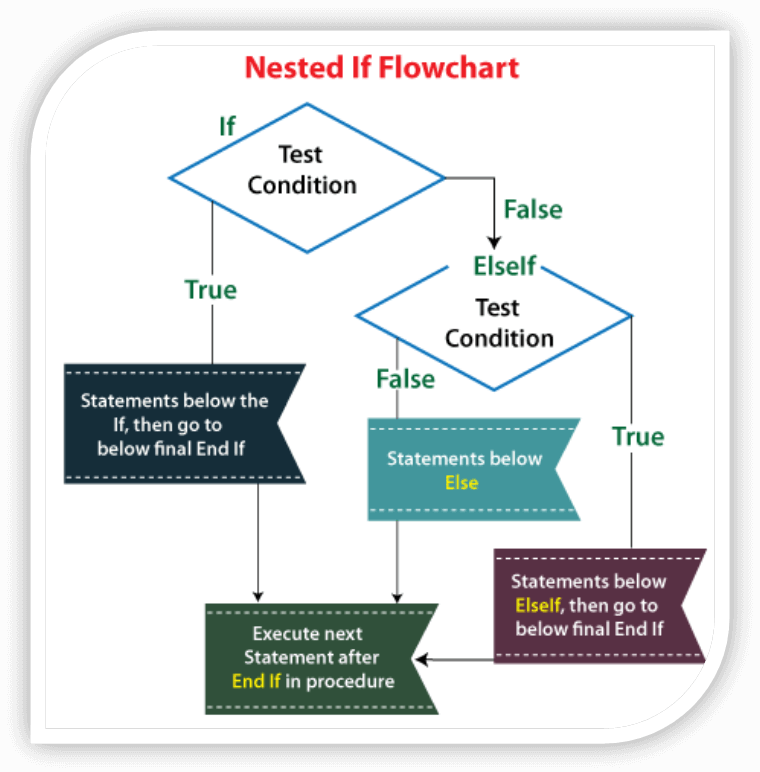
Example:
k =900; i=800; if( k ==900) if(i==800) fprintf('Value of k is 900 and i is 800\n'); end end fprintf('value of k is : %d\n', k ); fprintf('value of i is : %d\n',i);
output:
Value of a is 900 and b is 800 value of a is : 900 value of b is : 800
Switch Statement
Switch statement can be an alternative of if-else statements, it is used to test a input against set of rules already defined. This control statement executes one of the several group of statements.
Let us look at the syntax and few examples below.
Syntax:
switch switch_expr case case_exp1 set of Statements case case_exp2 set of Statements case case_exp..N set of Statements otherwise set of Statements end
Flowchart:
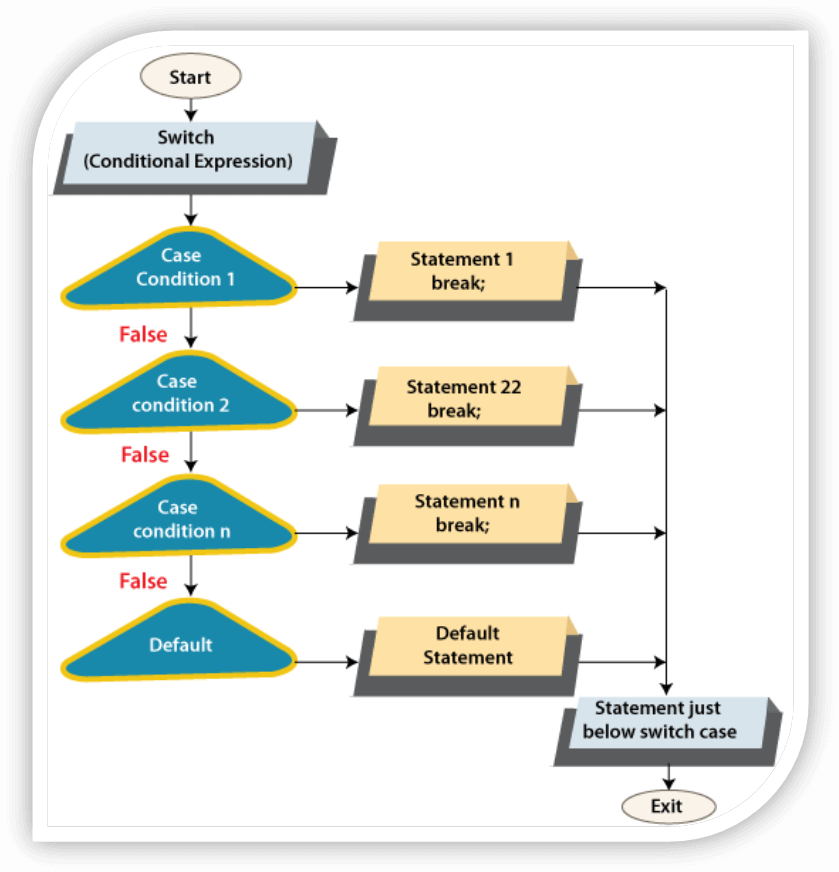
Example:
% program to convert number to string a = input('enter a number from 1 to 7 : ') switch a case 1 disp('number in wordsàone') case 2 disp(' number in wordsàtwo') case 3 disp(' number in wordsàthree') case 4 disp(' number in wordsàfour') case 5 disp(' number in wordsàfive') case 6 disp(' number in wordsàsix') case 7 disp(' number in wordsàseven') otherwise disp('not a valid number') end
Output:
enter a number: 4 number in wordsàfour