MATLAB Strings
You can address text in MATLAB utilizing string exhibits. Every component of a string exhibit stores a grouping of characters. The arrangements can have various lengths without cushioning, for example, "yes" and "no". A string exhibit that has just a single component is likewise called a string scalar.
You can list into, reshape, and connect string exhibits utilizing standard cluster tasks, and you can add text to them utilizing the + administrator. Assuming a string exhibit addresses numbers, you can change it's anything but a numeric cluster utilizing the twofold capacity.
To Create a String in Matlab
You can create a string placed a text in double quotes as shown below:
Str1 = "world, people"
Str1=
"world, people"
String array
We are creating a string array, we are concatenating string scalars like numbers can be concatenated with a numeric array, using square brackets.
Str1 = ["M" "Gem" "Apo";
"Sky" "Sky B" "IyS"]
Output:
Str1 =
2×3 string array
"M" "Gem" "Apo"
"Sky" "Sky B" "IyS"
We have used MATLAB online to execute this code and obtain the result as we can in this screenshot.
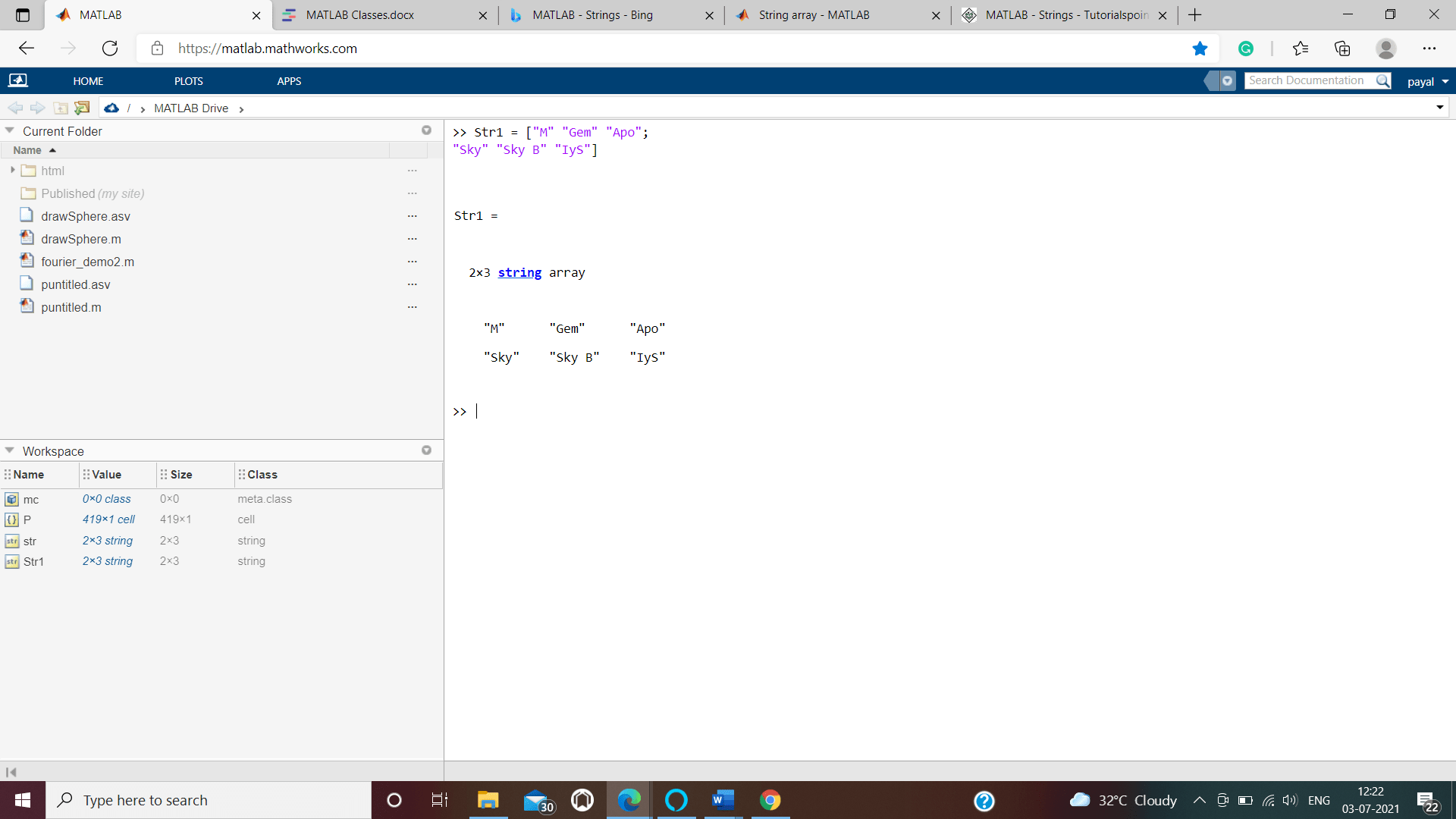
String Functions in MATLAB
MATLAB gives flexibility by providing many string functions for manipulating strings, creating, combining strings, modify strings, to blank, to copy, comparing and parsing strings.
String functions in MATLAB -
Function name | Description |
isspace | For Array elements that are space characters |
isstrprop | To Determine string category |
sscanf | To Read from string formatted data |
deblank | To Strip from end of string to trailing blanks |
strtrim | To Remove white space from string i.e leading and trailing |
char | To Represents character array |
iscellstr | To convert cell array of strings |
isletter | To check alphabetical letters |
isspace | To check space characters |
isstrprop | To Determine category of string |
strcat | To horizontally Concatenate the strings |
strjoin | For Joining strings into single string in cell array |
ischar | To check whether item is character array |
isletter | To Array elements that are alphabetic letters |
strfind | To Find one string within another |
strrep | To replace substring |
strsplit | To Split string at delimiter |
strtok | To Selected parts of string |
ischar | To check item is character array |
sprintf | To Format data into string |
lower | To Convert string to lowercase |
blanks | To represents blank characters |
cellstr | To change strings from character array |
validatestring | To Check validity of text string |
symvar | To Determine symbolic variables in expression |
regexp | To Match the regular expression |
regexpi | To match the regular expression |
regexprep | To Replace the given string |
regexptranslate | To translate the string to reg expression |
strncmpi | To Compare two characters of strings |
upper | To Convert into uppercase |
strjust | To Justify the character array |
Examples
To illustrate few string functions mentioned above -
Formatting the Strings
We are creating a script file and type the following code-
N = pi*100*ones(1,8);
sprintf(' %f \n %.2f \n %+.2f \n %12.2f \n %012.2f \n', N)
we are kind of multiplying the pi value with 100 and creating a matrix od ones then try to print the N variable by formatting the strings as follows.
Output:
ans =
' 314.159265
314.16
+314.16
314.16
000000314.16
314.159265
314.16
+314.16
let’s now execute this piece of code on MATLAB online to obtain the respective result as shown in the below screenshot.
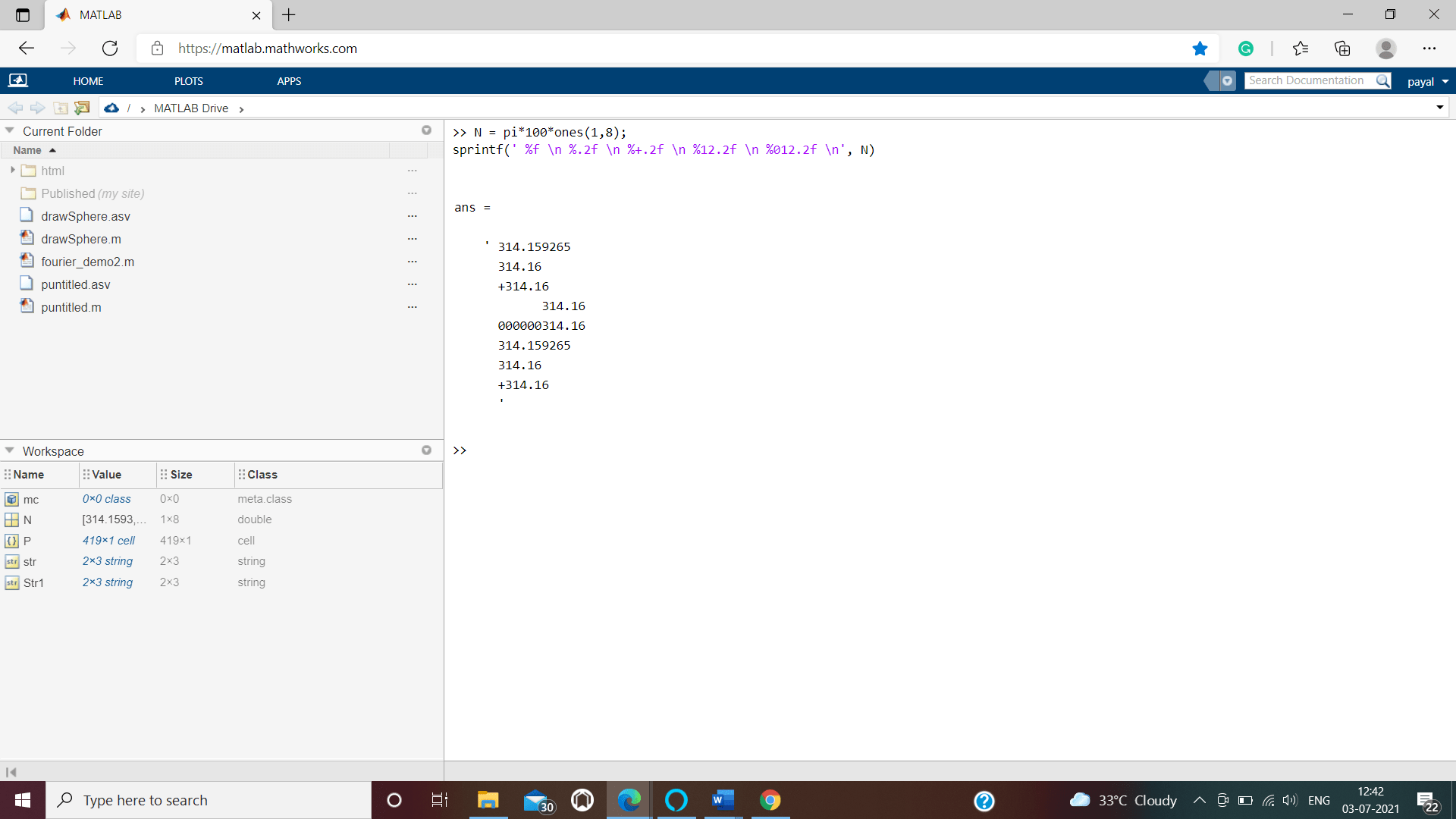
Joining the Strings
We are Creating a script file and type the following code-
We are creating cell array of strings and then joining two strings by using symbols “^” and “$” and storing the value in variables st1 and st2 as shown in the code below:
%cell array of strings
strmyarray = {'r','b','g', 'y', 'o'};
% cell array into single string
st1 = strjoin(str_array, "^")
st2 = strjoin(str_array, "$")
Output:
st1 =
'r^b^g^y^o'
st2 =
'r$b$g$y$o'
let’s now execute this piece of code on MATLAB online to obtain the respective result as shown in the below screenshot.
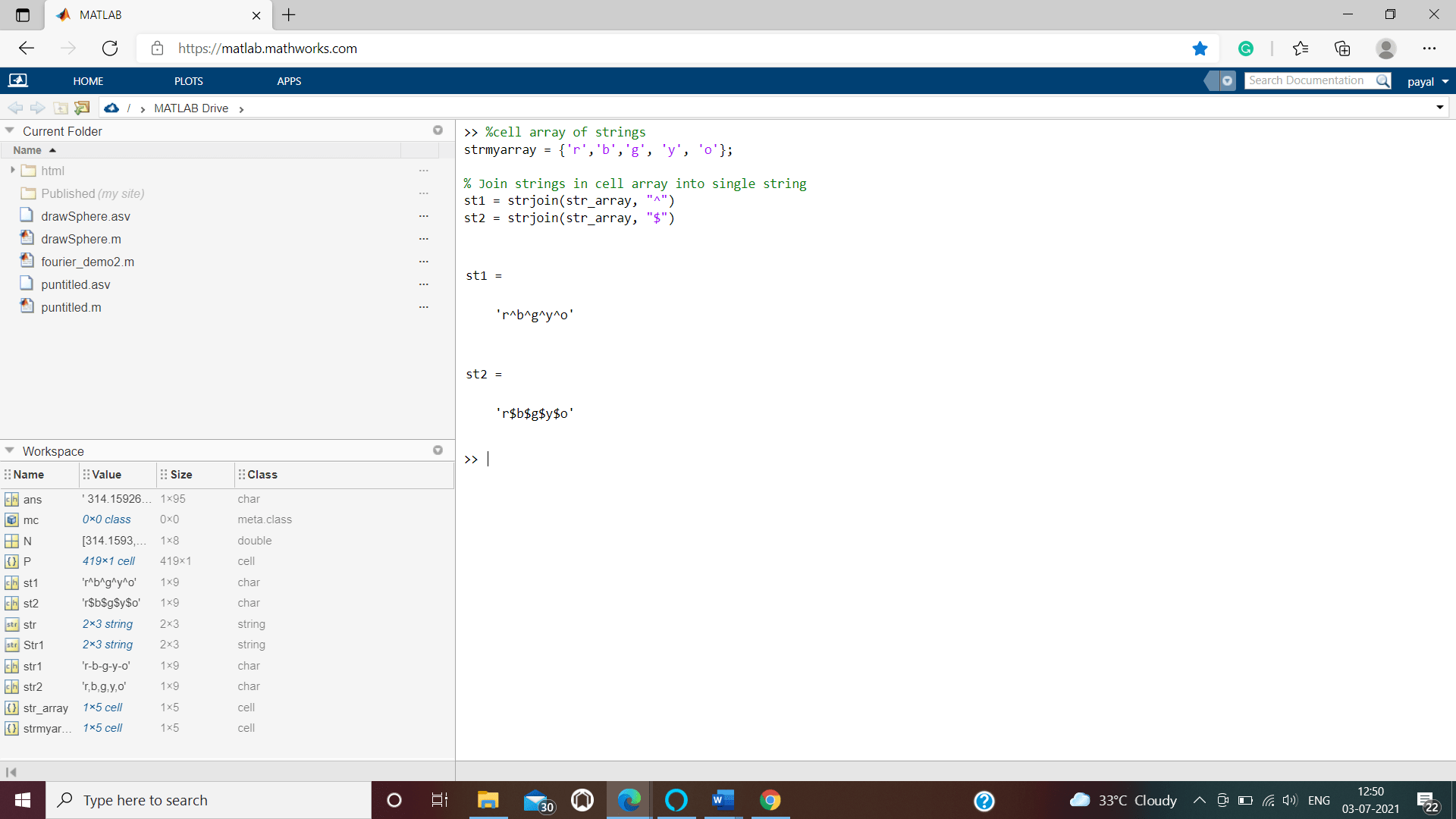
Replacing the Strings
We are Creating a script file and type the following code-
We are creating a dictionary type object studentsNAME and then applying methods like strrep to replace the content of the created object. Next we are displaying the first student names.
studentsNAME = {'Zali', 'ieha Bhatt', ...
'Mon Maik', 'padhu tam', ...
'Madhu Sharma', 'phawna Sharma',...
'yuu Ali', 'teva Dutta', ...
'Sun Ali', 'pofia bir'};
new_studentname = strrep(studentsNAME(8), 'Reva', 'Poulomi')
% Displaying the first student names
first_names_OF_STUDENT = strtok(studentsNAME)
OUTPUT:
new_studentname =
1×1 cell array
{'teva Dutta'}
first_names_OF_STUDENT =
1×10 cell array
{'Zali'} {'ieha'} {'Mon'} {'padhu'} {'Madhu'} {'phawna'} {'yuu'} {'teva'} {'Sun'} {'pofia'}
let’s now execute this piece of code on MATLAB online to obtain the respective result as shown in the below screenshot.
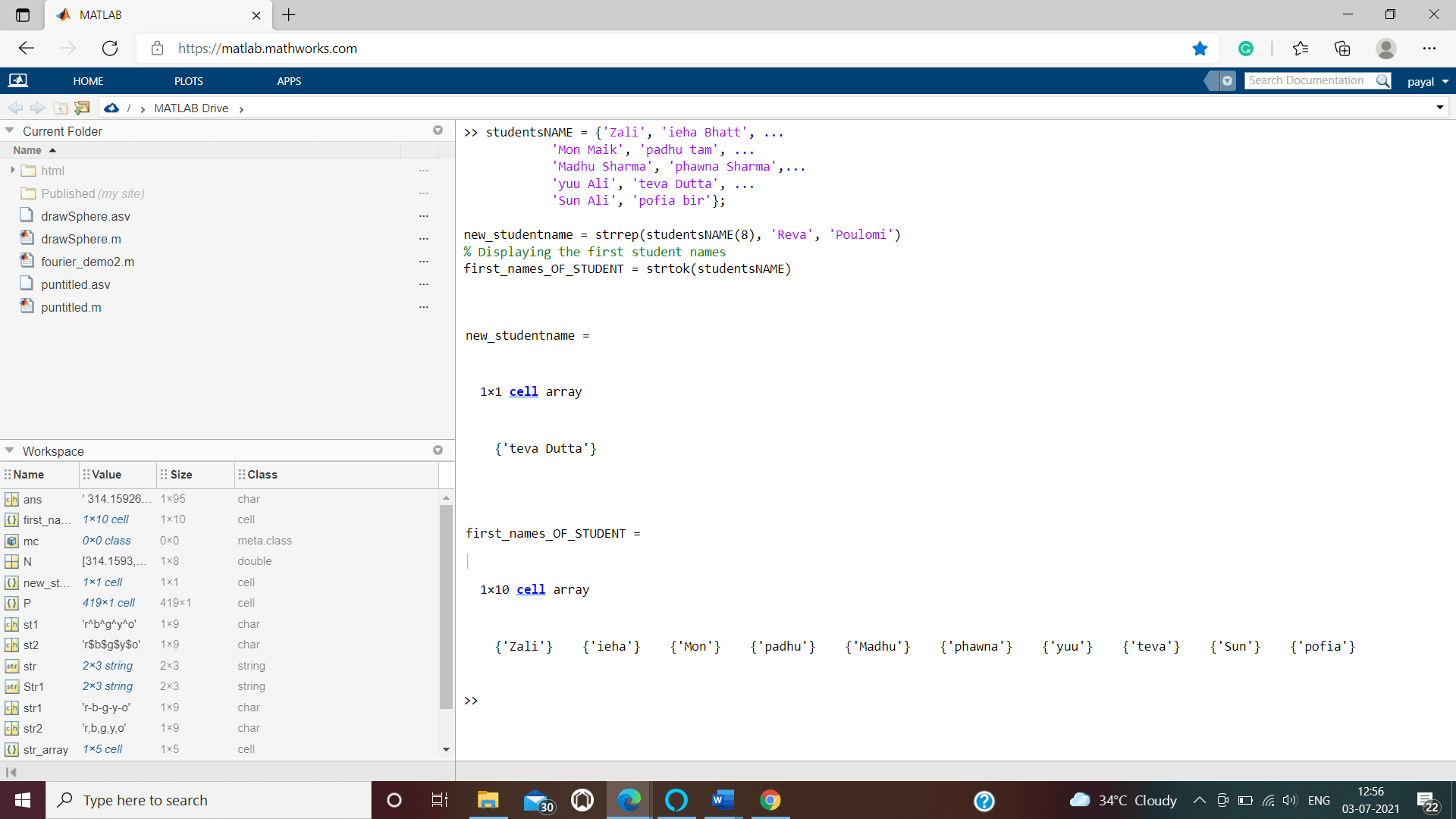
Multidimensional matrix as input
In this instance let us walk through an input which is a multidimensional matrix 3x3 dimensions (three rows and three columns). input is taken as a cell-matrix in addition to input data in alphabets format like { ' j ' , ' t ' , ' s ' ; ' a ' , ' a ' , ' p ' ; ' b ' , ' q ' , ' d ' } as shown below code.
Code:
clc ;
clear all ;
myinput = { ' j ' , ' t ' , ' s ' ; ' a ' , ' a ' , ' p ' ; ' b ' , ' q ' , ' d ' }
out1 = char ( myinput )
Output:
myinput =
3×3 cell array
{' j '} {' t '} {' s '}
{' a '} {' a '} {' p '}
{' b '} {' q '} {' d '}
out1 =
9×3 char array
' j '
' a '
' b '
' t '
' a '
' q '
' s '
' p '
' d '
myinput = { ' j ' , ' t ' , ' s ' ; ' a ' , ' a ' , ' p ' ; ' b ' , ' q ' , ' d ' }
let’s now execute this piece of code on MATLAB online to obtain the respective result as shown in the below screenshot.
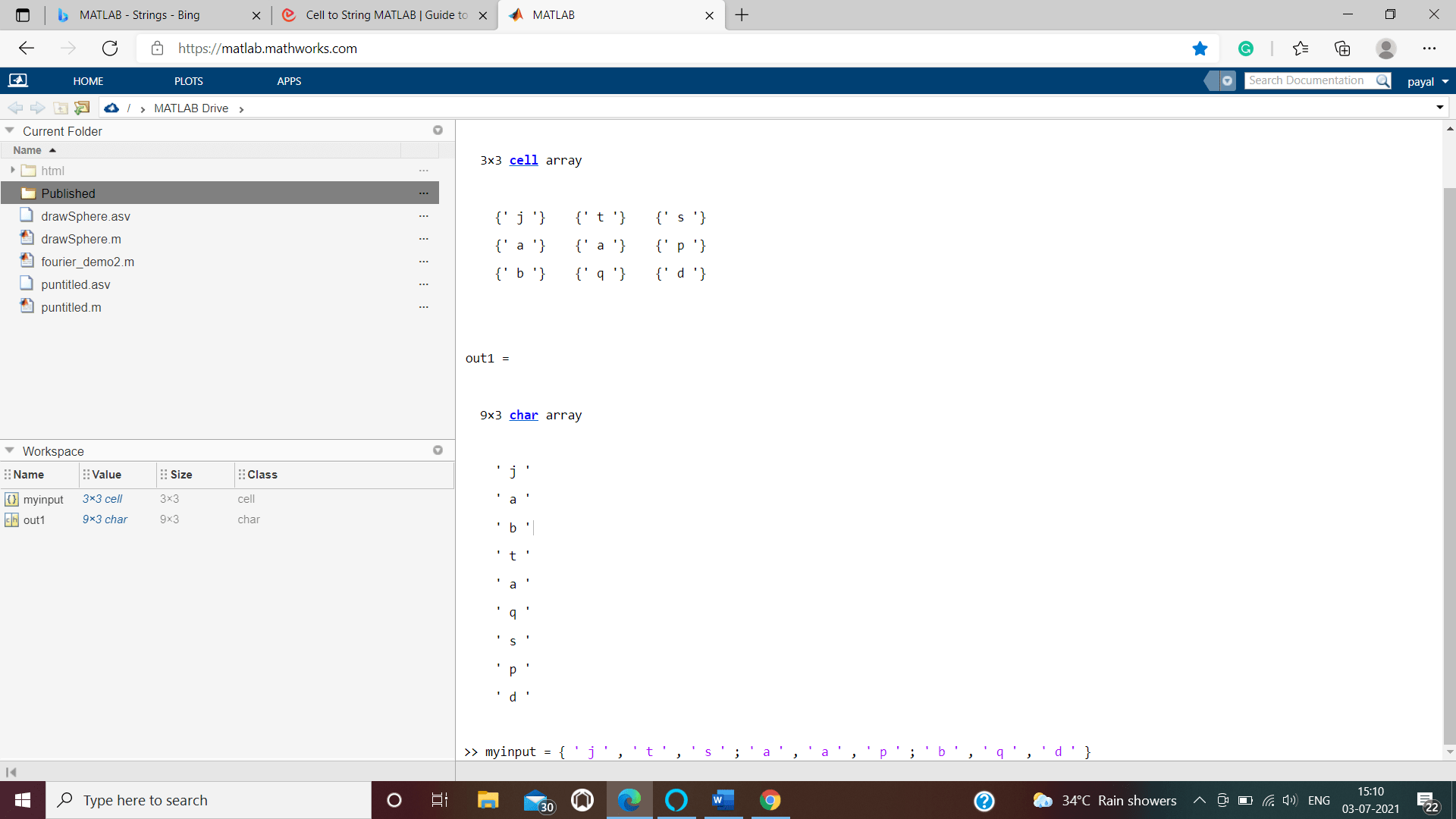
Declaring and initializing data types into one object
Now let us take input in form of various types like as integer, character, string, and float. In our code, input is assigned with a one-dimensional vector, data as follows ‘world HELLO ‘, ‘ 756 ‘, ‘ BYE ‘, ‘ 22.98 ‘,’ H E Y ‘, ‘ 190 ‘ }. in this code world HELLO, BYE, HEY are strings. 365 and 190 are integers and 22.98 is different datatype i.e float.
Example:
clc ;
clear all ;
myinput = {'world HELLO ' , ' 756 ' , ' BYE ' , ' 22.98 ' ,' H E Y ' , ' 190 ' }
myout = char( myinput )
myout ( 3 )
myout(5)
myout(1)
Output:
myinput =
1×6 cell array
{'world HELLO '} {' 756 '} {' BYE '} {' 22.98 '} {' H E Y '} {' 190 '}
myout =
6×12 char array
'world HELLO '
' 756 '
' BYE '
' 22.98 '
' H E Y '
' 190 '
ans =
' '
ans =
' '
ans =
'w'
Conclusion
In this tutorial, we have learnt how to change cell array into data type string by utilizing char function or also string function. We can transform all, character, types of data integer, types of float, etc. into the prescribed string format. We can transform formats like float, one-dimensional array, and single element and so on into the required format of data.