TD in HTML
In HTML, a table cell is defined by the td element. The letter "td" in HTML stands for "table data." It is an HTML element utilized to specify specific HTML table cells. "td" elements represent the actual information within each column of HTML tables, which are used to organize and display information in an organized tabular way.
Here's the basic syntax of a "td" element:
<td>Table Data</td>
Here's an example of how "td" elements are utilized within an HTML table:
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
}
</style>
</head>
<body>
<h1>The td element</h1>
<p>The td element defines a cell in a table:</p>
<table>
<tr>
<td>Cell A</td>
<td>Cell B</td>
</tr>
<tr>
<td>Cell C</td>
<td>Cell D</td>
</tr>
</table>
</body>
</html>
Output:
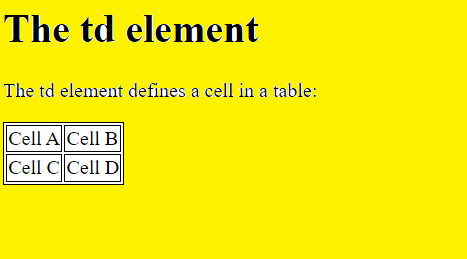
In this example:
- `<table>` is the container for the entire table.
- `<tr>` defines table rows.
- `<th>` defines table headers (usually displayed in bold and centred by default).
- `<td>` defines table data cells (contains the actual data in each cell).
At last, to organize and show tabular data on web pages, "td" elements are a critical component of HTML tables. To achieve the required design and functionality, they describe the specific data cells inside the table and may be customized using attributes and CSS.
Fixed Column Width using <td> Tag in a Table
The browser automatically adjusts the size of the rows and columns in an HTML table so that table contents fit in the row. The width of the columns may, however, occasionally need to be set or uniform in size. Therefore, there are several ways to fix the column's width.
The width of a specific column may be fixed using the <td> tag width property. We may give this property a numerical number or a percentage.
Example:"
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
}
</style>
</head>
<body>
<h1> Width using <td> tag in a table</h1>
<table border="1">
<tr>
<td width="100">Column 1</td>
<td width="200">Column 2</td>
<td width="30%">Column 3 (30%)</td>
</tr>
<tr>
<td>Row 2, Column 1</td>
<td>Row 2, Column 2</td>
<td>Row 2, Column 3</td>
</tr>
</table>
</body>
</html>
Output:
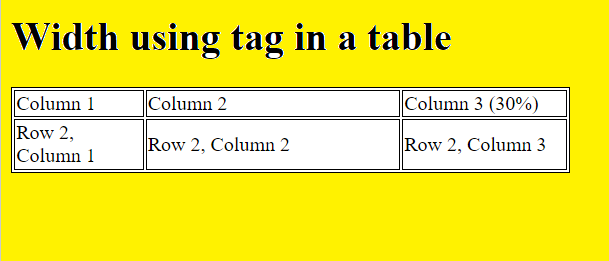
How to Add Background Color to Table Cell (with CSS)
A table cell (or <td> element) in an HTML table can have a background color added to it via CSS. Here is how to go about it:
Adding the style property straight to the <td> element within your HTML code is possible using inline CSS. Here's an illustration:
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
}
</style>
</head>
<body>
<h1>Add Background Color to td</h1>
<table>
<tr>
<th>Month</th>
<th>Savings</th>
</tr>
<tr>
<td style="background-color:#FF0000">January</td>
<td style="background-color:#00FF00">$100</td>
</tr>
</table>
</body>
</html>
Output:
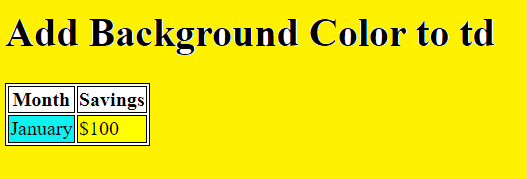
The background-colour property is explicitly specified using the style attribute for each <td> element in the above instance. Any valid CSS colour representation, including a colour name, hexadecimal colour code, RGB value, and more, may be used to set the background colour.