HTML Code
Before jumping into the topic, let’s understand a common scenario where we have a code snippet in our HTML file. By code snippet, we mean computer code, which is written in some computer programming language like JavaScript.
In order to reflect this computer code uniquely among the regular HTML script/code, we use the code tag in HTML.
<code> in HTML
The <code> tag in HTML is a phrase tag, which has no attribute of its own. Do you have a computer code (computer programming code) in your HTML text content? No worries, the <code> tag comes into play in such scenarios. It is used to display a piece of computer code.
The output generated after the introduction of this tag is displayed in the monospace font. The font is generally the browser’s default monospace font.
Syntax:
<code> “computer code in some programming language” </code>
Attribute support
The code tag does not have attributes of it’ s own, but there are some global attributes in HTML which are supported by the code tag. These are as follows:
- Global attributes: attributes that can be used with all HTML elements.
- Event attributes
Types:
- Window events
- Form events
- Keyboard events
- Mouse events
- Media events
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<p>Let's consider this basic code for creating a table in HTML:</p>
<pre>
<code>
<table>
<tr>
<td></td>
<td></td>
</tr>
</table>
</code>
</pre>
</body>
</html>
Output:
Let's consider this basic code for creating a table in HTML:
<table>
<tr>
<td></td>
<td></td>
</tr>
</table>
Code 1: HTML + program code (in JS) without code tag
<!DOCTYPE html>
<html>
<head>
<title>Revision 1</title>
</head>
<body style="background-color: rgb(252, 245, 230)">
<h1 style="text-align: center; color: rgb(32, 116, 243)">Hello! World</h1>
<h2 style="text-align: left; color: saddlebrown">Recipe for Chai (Tea):</h2>
<ol type="1">
<li>Add 1 cup of water to a container</li>
<li>Boil the water</li>
<li>
Add the following contents in it:
<ol type="a">
<li>Ginger and cardamom</li>
<li>Two tsp sugar</li>
<li>1 tsp of tea leaves</li>
<li>1 cup of milk</li>
</ol>
</li>
<li>Let the mixture come to a boil</li>
<li>The tea is ready</li>
</ol>
<hr />
<p style="text-align: center">* * * * * * * * * * * * * *</p>
<hr />
<ul>
<li>
<b style="color: rgb(193, 63, 253); font-style: italic">
Let's suppose we need to make 'n' number of cups of tea using the same
recipe, but since the quantity is not 1 cup, hence we need to
calculate the ratio of ingredients depending on the number of cups.
</b>
<br />
<b
>Let's make this activity fun by adding a demo function to try to
calculate the ratio using a function in JavaScript</b
>
</li>
<br />
<li>The following is the JavaScript code :</li>
<p style="color: rgb(253, 25, 120)">
const chai_maker = function (cups) { const liquids = cups / 2;
console.log(`The quantity of milk and water should be ${liquids} cups
each`); const masala = cups / 1.6; console.log( masala <= 3 ? "Add 1
piece of each ingredient stated above" : `Add ${Math.floor(masala)}
piece of each ingredient stated above` ); }; chai_maker(3);
chai_maker(5); chai_maker(9);
</p>
</ul>
<hr />
<hr />
</body>
</html>
Output:

Example 2: formatting the code using <code>
<!DOCTYPE html>
<html>
<head>
<title>Revision 1</title>
</head>
<body style="background-color: rgb(252, 245, 230)">
<h1 style="text-align: center; color: rgb(32, 116, 243)">Hello! World</h1>
<h2 style="text-align: left; color: saddlebrown">Recipe for Chai (Tea):</h2>
<ol type="1">
<li>Add 1 cup of water to a container</li>
<li>Boil the water</li>
<li>
Add the following contents in it:
<ol type="a">
<li>Ginger and cardamom</li>
<li>Two tsp sugar</li>
<li>1 tsp of tea leaves</li>
<li>1 cup of milk</li>
</ol>
</li>
<li>Let the mixture come to a boil</li>
<li>The tea is ready</li>
</ol>
<hr />
<p style="text-align: center">* * * * * * * * * * * * * *</p>
<hr />
<ul>
<li>
<b style="color: rgb(193, 63, 253); font-style: italic">
Let's suppose we need to make 'n' number of cups of tea using the same
recipe, but since the quantity is not 1 cup, hence we need to
calculate the ratio of ingredients depending on the number of cups.
</b>
<br />
<b
>Let's make this activity fun by adding a demo function to try to
calculate the ratio using a function in JavaScript</b
>
</li>
<br />
<li>The following is the JavaScript code :</li>
<code style="color: rgb(253, 25, 120)">
const chai_maker = function (cups) { const liquids = cups / 2;
<p>
console.log(`The quantity of milk and water should be ${liquids} cups
each`);
</p>
<p>const masala = cups / 1.6;</p>
console.log( masala <= 3 ? "Add 1 piece of each ingredient stated above"
: `Add ${Math.floor(masala)} piece of each ingredient stated above` );
};
<p>chai_maker(3); chai_maker(5); chai_maker(9);</p>
</code>
</ul>
<hr />
<hr />
</body>
</html>
Output:

//Formatted text by <code>
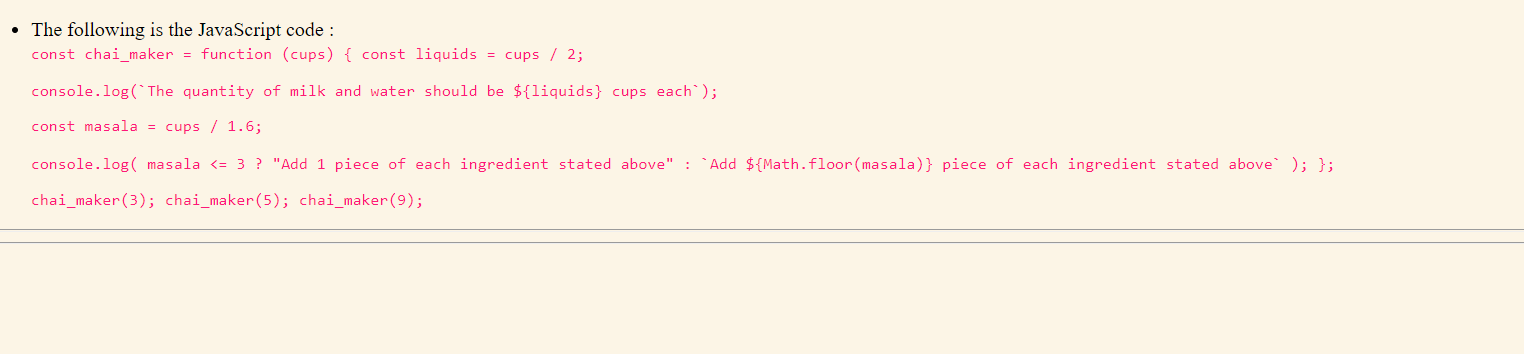
Some important attributes/tags related to code
Name | Description |
<kbd> | It defines keyboard input. |
<samp> | It specifies a sample output from a computer program. |
<pre> | Defines preformatted text. |
<dfn> | It defines a definition term. |
<var> | It defines a variable. |
<strong> | It defines an important text. |