Android Activity
What is an Activity?
Android Activity is a screen in the user interface of an Android application. In this way, Android activity is very similar to the desktop application window. An Android application can unlimitedactivities,which means there is no restriction on the number of screens. An app launches by displaying the launcher activity, and then the application can allow an opening for further activities.
Activity Lifecycle
When the Android app is launched for the first time, basic activity is generated. The activity then undergoes three states, Create, start, and resume, which were generated before they were ready to serve the user.
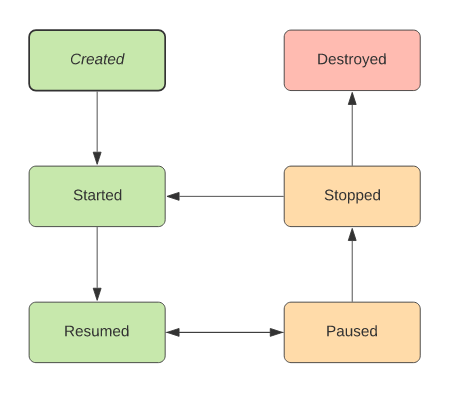
Android Activity Lifecycle
When the current activity opens another activity (screen), these activities are undergoing the same three states when opened.
For example, if activity A opens another activity B, activity A gets paused and gets pushed into the stack. This means that activity A will be suspended. When the user clicks back to return to A, activity A returns to the resume state. When the user returns to the home screen of the Android device, all activity is paused and then stopped. After that, when the user returns to the app, the activity goes through the start state and resume state. If your Android device requires memory that Android applications occupy the device's memory, your app can be destroyed. In other words, the Android pushes the activity in a destroyed state.
The activity state always follows the arrows in the figure above. You cannot move an activity directly from creation to resume. Activities are always created, started, and restarted in that order. Resuming an activity can vary from suspend and resume from suspend to suspend, starting from the back, but cannot directly stop and resume.
An activity class represents all the activities in an Android application. These activity classes are subclasses of android.app.Activity. The Activity class contains a set of methods that correspond to the life cycle states that an activity can be in. The methods are as follows:
- onCreate():
This is the first method, which is called on the creation of activity for the first time.
- onStart():
When the application launches and theusercan see activity, this method is called.
- onRestart():
When the activity is restarted after it has been stopped, onRestart() method is called.
- onResume():
When the user makes interaction with the app, this method is called.
- onPause():
When the current activity is minimized or a new activity is started, and the previous getspaused, onPause() method is called.
- onStop():
When the activity is minimized or no longer visible, onStop() is called.
- onDestroy():
When the android OS destroys the activity, onDestoyed()method is called.
Create Activity
The Activity class inherits from the "AppCompatActivity" base class. The name "Compat" indicates Compatibility, which means that even if the class is the latest version, it will support the previous features. The activity class does not have a self-generated init () block, so you cannot instantiate the activity class yourself. This is handled by the system itself and the programmer can change the content of the activity. The instance Activity class can be created using the following steps with the help of callbacks.
Follow these steps to create an activity:
1. First, right-click on Apps. Then select New -> Activity and select the activity according to your requirements.
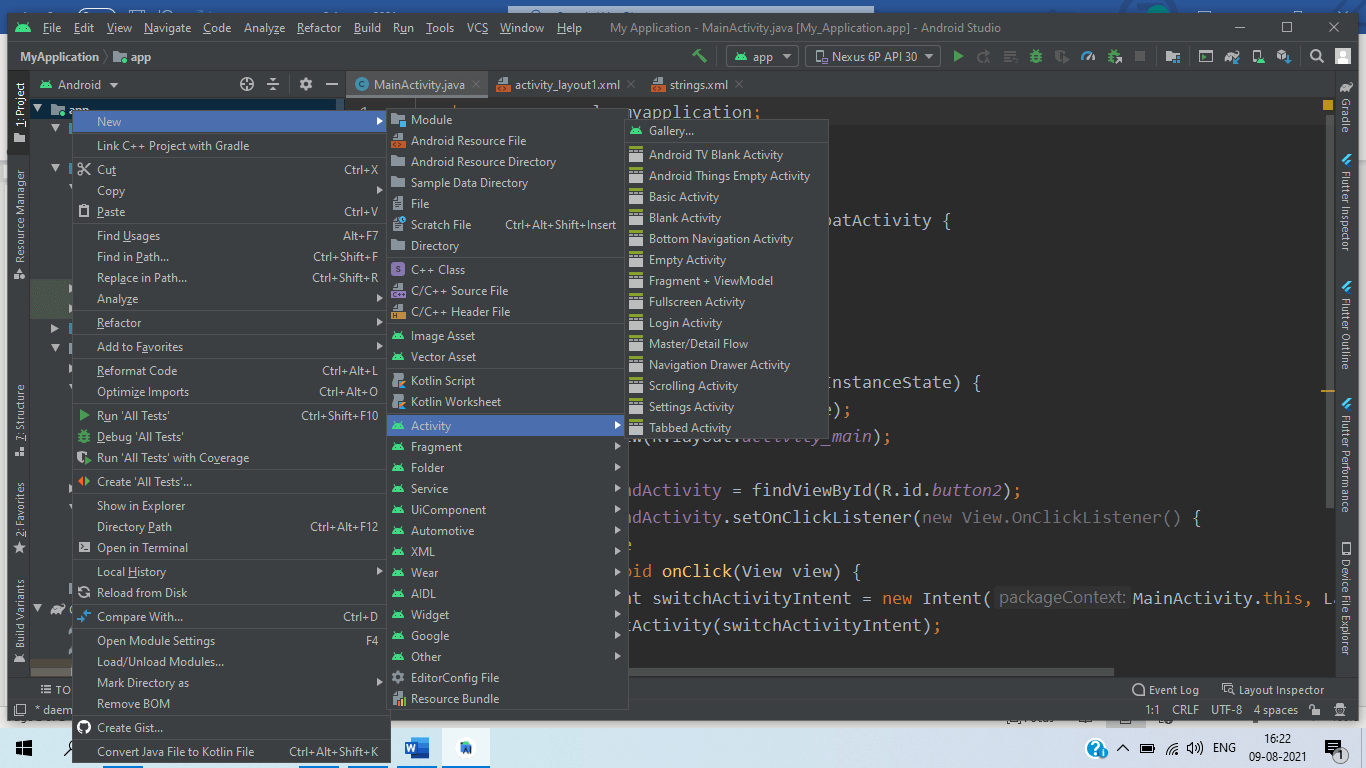
2. Then define the activity in Configure Activity window in AndroidStudio. Enter "Activity Name" and "Package Name" in the text boxes and check the checkboxes to create the layout of the activity and click Finish.
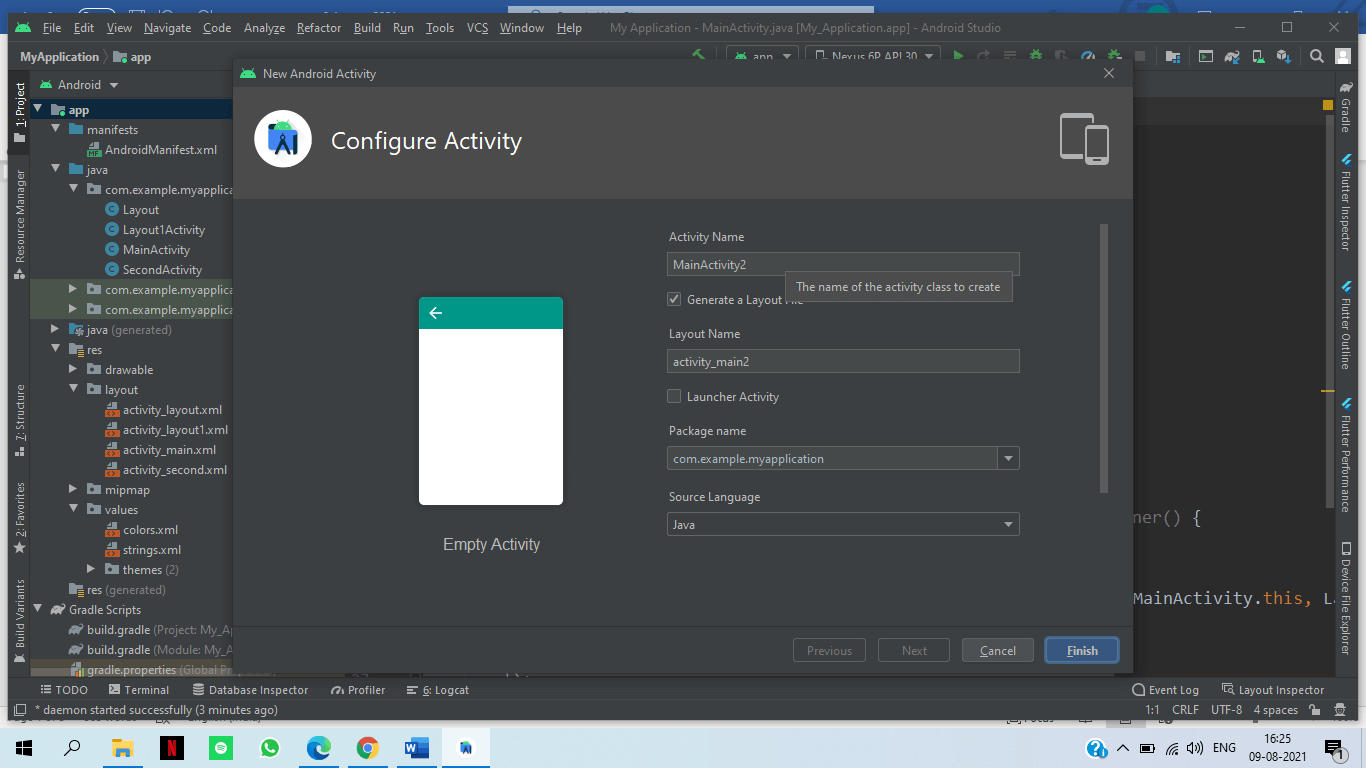
A new activity with the name you entered will be created with the following code:
public class MainActivity2extends AppCompatActivity{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);//OnCreate() method
setContentView(R.layout.activity_main2);
}
}
The function onCreate() is called when the Activity is created. setContentView() is used to set the layout that we declared in the XML file to the Activity.
R is the resource class in Android which is used to access the resources within the code segment.
An Android application can have an unlimited number of activities. Every activity you create for the application must be declared in the XML manifest file, and the launcher activity of the application must be declared with an intent filter, which comprises the action, and the category attributes, as shown below:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.MyApplication">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>