Android Studio Resources
To build an outstanding Android application there are many items that you need to use. Aside from the coding of your application, you need to handle various resources such as bitmaps, menus, colors, layout definitions, animations, etc. static content used by your code.
These resources are managed in various subdirectories under the project's res/ directory.
Organizing Resources in Android Studio
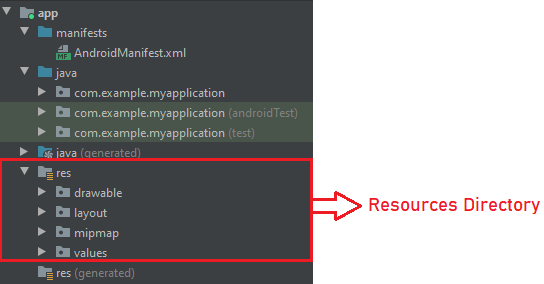
1) drawable/
Image files like .png, .jpg, .gif or bitmaps, shapes, animation drawable derived from XML files. These are storedin res/drawable/ and accessed from the R.drawable class.
2) layout/
XML files that define the layout of the activities. These are stored in res/layout/ and accessed from the R.layout class.
3) values/
XML files containing values like strings, integers, colors, etc.The following table shows the file naming conventions for resources that can be stored inthe values directory:
Resource | File Name |
arrays | arrays.xml |
color values | colors.xml |
styles | styles.xml |
String values | strings.xml |
4) mipmap/
Drawable files for various launcher icon densities are stored in res/mipmap.
5) anim/
XML files defining property animations. These are stored in the res/anim/ folder and can be accessed using R.anim class.
6) color/
XML files that define a list of color states. These are stored in res/color/ andcan be accessed using R.color class.
7) menu/
XML files that define application menusincluding options menus, context menus, and submenus. These are stored in res/menu/ and can be accessed using R.menu class.
8) raw/
Any file you save in raw format. To open these raw files, you need to call Resources.openRawResource() with the resource ID (R.raw.filename).
Alternative Resources
Applications you develop must provide alternative resources to support different types of device configurations. To make your application work properly on any configuration you should include alternative drawable resources and string resources. At run time, Android detects the configuration of the device and loads the resources with respect to the device’s configuration for the application.
Follow these steps to assign configuration-specific resources:
- Create a new sub-directory in res/ with name as <resources_name><config_qualifier>. In this, resources_name will be either of the resources such as values, layouts, mipmap, etc. Qualifiers specify the individual configurations that use these resources. A list of full modifiers for various types of resources can be found in the official documentation.
- Save each alternate resource in this new directory. The name of the resource filesmust be the same as the basicresource files, but these files would requireconfiguration-specific alternative content. For example, an image file with the same name but a higher resolution screen will have a higher resolution.
Below is an example of specifying a default language layout and an alternative Korean language layout.
MyFirstProject/
app/
manifest/
AndroidManifest.xml
java/
MyActivity.java
res/
drawable/
icon.png
background.png
layout/
activity_main.xml
info.xml
layout-kr/
main.xml
values/
strings.xml
Accessing Resources
You need access to the resources defined in your code or layout XML file during application development. The following describes how to access resources in both scenarios:
A. In Code
When an Android application is compiled, an R class is created. This contains the res IDs of all resources present in the res/ directory. You can use R classes to access resources using subdirectories and resource names, or directly using resource IDs.
Example 1
To access res/drawable/icon.jpg and set an ImageView with image resource you will use the following code:
In this, an object of Image View has been created using R.id.img1 to get ImageView definedwith idimg1 in a layout file. Then to set the image resource in Image View, R.drawable.icon is used to get the icon image available in the drawable sub-directory.
ImageViewimageView= (ImageView) findViewById(R.id.img1);
imageView.setImageResource (R.drawable.icon);
Example 2
Let’s take an example where res/values/strings.xml has following string resources:
<resources>
<string name="app_name">My Application</string>
<string name="main_activity">Main Activity</string>
</resources>
Now to set the text on a TextView object with ID name using a resource ID you will use the following code:
TextViewmessageText= (TextView) findViewById(R.id.text1);
messageText.setText(R.string.app_name);
Example 3
Let’s take a layout res/layout/activity_main.xml as follows:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayoutxmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="match_content"
android:layout_height="match_content"
android:text="@string/main_activity"
android:textSize="18sp"
android:textStyle="bold"/>
<ImageView
android:id="@+id/img1"
android:layout_width="150dp"
android:layout_height="175dp"
android:layout_gravity="top|center_horizontal"
android:layout_margin="10dp"
android:paddingBottom="30dp"
android:src="@mipmap/ic_launcher_profile" />
</RelativeLayout>
This following java code will be usedto load the above layout for an Activity:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
B. In XML
Let’s take the following resource XML res/values/colors.xml file that includes following color resource:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="purple_200">#FFBB86FC</color>
</resources>
Now to use this resource in the layout file to set the text color you will use “@color/purple_200”:
<?xml version="1.0" encoding="utf-8"?>
<EditTextxmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:textColor="@color/purple_200"/>