Android Content Providers
In Android, Content Providers are a very important component, which serves the purpose of relational databases and is used to store application data. The purpose of the content provider in the Android OS is like a central repository, where the application data is stored, allowing other applications to easily retrieve and safely modify data according to the user's needs.
The Android system allows content providers to store application data in various ways. Users can manage app data such as images, audios, personal contact information, andvideos in SQLite databases, files, and even the network.
nmContent providers have certain permissions to grant or revoke the right of other apps to utilize or interfere with the data.
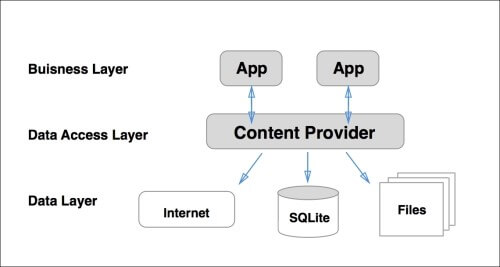
Content URI
The main concept of a content provider is a content Uniform Resource Identifier (URI). The URI is used as a query string to yield data from the content provider.
The structure of the content URI: content://authority/Path_optional/ID_optional
Details of the other parts of the content URI:
- content://: Required part of the URI indicating that the specified URI is a content URI.
- Permissions: Indicates content provider’s name, such asbrowser or messenger. This part must be unrepeated across all content providers.
- Path_optional: Specifies the data type provided by the content provider. This part is important because it is useful for content providers to aid various types of data that are not interrelated, such as video and audio files.
- ID_optional: The number to use when you need to retrieve a particular tuple. When an ID is mentioned in a URI, it is an ID-based URI, otherwise, it is a directory-based URI.
Content Provider Operations
Content providers have four basic operations: read, create, update, and delete. These tasks are often referred to as CRUD operations.
- Create: Used for creating data in the content provider.
- Read: Requesting data from the content provider.
- Update: Modify existing data.
- Delete: Deletes the existing data from the store.
Content Provider Working
UI components in Android applications, such as Activities and Fragments, use the object CursorLoader to send query requests to the ContentResolver. Operations requests (create, read, update, delete, etc) are sent as clients to the ContentProvider by theContentResolver object. The ContentProvider processes the request after receiving it from ContentResolver and returns the desired outcome.
Below is animage representing the working of the content provider.
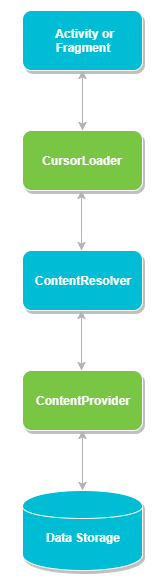
Creation of Content Provider
To create a content provider, the important steps to follow are:
- In the MainActivity file’s directory create a new java class andthis class should inherit the ContentProviderparent class.
- To access content, define the URI address of the content provider.
- For storing application data, make a Database.
- Override the 6 abstract methods of the ContentProviderparent class.
- The content provider should be registered in the AndroidManifest.xml file using the tag.
Below are the methods that the content provider child class must override for the content provider to work.
- onCreate() When the provider is created, this method is called.
- query() It receives the client’s request and returns a Cursor object when it is called.
- insert() For inserting a new tuple into the content provider, this method is called.
- delete() For deleting an existing tuple from the content provider, this method is called.
- update() For updating an existing tuple from the content provider, this method is called.
- getType() For getting the data MIME type at the given URI, this method is called.
Content Provider Example
Below is an example of using a content provider in an Android application. Here you create your own content provider to insert and retrieve data into your Android application.
Create an Android application project using the Android Studio IDE. Specify a name for the project as contentprovider or whatever you like and the package name should be com.example.contentprovider as described in the Android Studio tutorial.
Now the next step is to create your own content provider file DetailsProvider.javain \src\main\java\com.myapplication.Javatpoint.contentprovider package.To define our actualprovider and associated methods right-click on your application folder and Go to New and select Java Class and give name as DetailsProvider.java.
Once the new file DetailsProvider.javahas been created, open it, and implement the code as follows:
package com.example.myapplication.Javatpoint;
import java.util.*;
import android.content.Context;
import android.content.UriMatcher;
import android.database.Cursor;
import android.content.ContentProvider;
import android.content.ContentUris;
import android.content.ContentValues;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteException;
import android.database.sqlite.SQLiteOpenHelper;
import android.database.sqlite.SQLiteQueryBuilder;
import android.net.Uri;
public class DetailsProviderextends ContentProvider{
static final String id = "id";
static final String name = "name";
static final String DETAILS_PROVIDER = "com.example.myapplication.Javatpoint.DetailsProvider";
static final String URL = "content://" + DETAILS_PROVIDER + "/users";
static final Uri URI= Uri.parse(URL);
static final int URI_CODE = 1;
static final UriMatchermatcher;
static {
matcher = new UriMatcher(UriMatcher.NO_MATCH);
matcher.addURI(DETAILS_PROVIDER, "users", URI_CODE);
matcher.addURI(DETAILS_PROVIDER, "users/*", URI_CODE);
}
private SQLiteDatabaseliteDatabase;
static final String NAME_OF_DATABASE = "StudDB";
static final String NAME_OF_TABLE = "StudentName";
static final int DB_VERSION = 1;
static final String TABLE_CREATION = " CREATE TABLE " + NAME_OF_TABLE+ " (id INTEGER PRIMARY KEY AUTOINCREMENT, "
+ " name TEXT NOT NULL);";
@Override
public String getType(Uri uri) {
switch (matcher.match(uri)) {
case URI_CODE:
return "vnd.android.cursor.dir/users";
default:
throw new IllegalArgumentException("Not supported URI: " + uri);
}
}
@Override
public booleanonCreate() {
Context context= getContext();
DatabaseHelper helper = new DatabaseHelper(context);
liteDatabase= helper.getWritableDatabase();
if (liteDatabase!= null) {
return true;
}
return false;
}
private static HashMap<String, String>val;
@Override
public Cursor query(Uri uri, String[] projection, String select,
String[] selectArgs, String sortOrder) {
SQLiteQueryBuilderqueryb= new SQLiteQueryBuilder();
queryb.setTables(NAME_OF_TABLE);
switch (matcher.match(uri)) {
case URI_CODE:
queryb.setProjectionMap(val);
break;
default:
throw new IllegalArgumentException("URI Not Known: " + uri);
}
if (sortOrder == null || sortOrder == "") {
sortOrder = id;
}
Cursor cur = queryb.query(liteDatabase, projection, select, selectArgs, null,
null, sortOrder);
cur.setNotificationUri(getContext().getContentResolver(), uri);
return cur;
}
@Override
public Uri insert(Uri uri, ContentValuesvalu) {
long row_ID= liteDatabase.insert(NAME_OF_TABLE, "", valu);
if (row_ID>0) {
Uri _uri= ContentUris.withAppendedId(URI, row_ID);
getContext().getContentResolver().notifyChange(_uri, null);
return _uri;
}
throw new SQLiteException("Adding a record failed" + uri);
}
@Override
public int update(Uri uri, ContentValuesval, String select,
String[] selectArgs) {
int ct = 0;
switch (matcher.match(uri)) {
case URI_CODE:
ct = liteDatabase.update(NAME_OF_TABLE, val, select, selectArgs);
break;
default:
throw new IllegalArgumentException("URI Not Known: " + uri);
}
getContext().getContentResolver().notifyChange(uri, null);
return ct;
}
@Override
public int delete(Uri uri, String select, String[] selectArgs) {
int ct = 0;
switch (matcher.match(uri)) {
case URI_CODE:
ct = liteDatabase.delete(NAME_OF_TABLE, select, selectArgs);
break;
default:
throw new IllegalArgumentException("URI Not Known: " + uri);
}
getContext().getContentResolver().notifyChange(uri, null);
return ct;
}
private static class DatabaseHelperextends SQLiteOpenHelper{
DatabaseHelper(Context context) {
super(context, NAME_OF_DATABASE , null, DB_VERSION);
}
@Override
public void onCreate(SQLiteDatabaseliteDatabase1) {
liteDatabase1.execSQL(TABLE_CREATION);
}
@Override
public void onUpgrade(SQLiteDatabasesqLiteDatabase, int oldV, int newV) {
sqLiteDatabase.execSQL("DROP TABLE IF EXISTS " + NAME_OF_TABLE);
onCreate(sqLiteDatabase);
}
}
}
Now open the activity_main4.xml layout file from layout directory in resources package and implement or modify the code as follows:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id= "@+id/display"
android:layout_width= "wrap_content"
android:layout_height= "wrap_content"
android:layout_marginStart= "112dp"
android:layout_marginTop= "128dp"
android:text= "Name"
app:layout_constraintStart_toStartOf= "parent"
app:layout_constraintTop_toTopOf= "parent" />
<EditText
android:id="@+id/enterName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="10dp"
android:ems="10"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/display" />
<Button
android:id="@+id/btnAdd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="112dp"
android:layout_marginTop="12dp"
android:onClick="onClickAddData"
android:text="Add User"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/enterName" />
<Button
android:id= "@+id/btnGET"
android:layout_width= "wrap_content"
android:layout_height= "wrap_content"
android:layout_marginStart= "112dp"
android:layout_marginTop= "8dp"
android:onClick= "onClickShowData"
android:text= "Show Users"
app:layout_constraintStart_toStartOf= "parent"
app:layout_constraintTop_toBottomOf= "@+id/btnAdd" />
<TextView
android:id="@+id/data"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:clickable="false"
android:ems="10"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/btnGET" />
</androidx.constraintlayout.widget.ConstraintLayout>
Now open MainActivity.java file and implement the code like as follows:
package com.example.myapplication.Javatpoint;
import android.os.Bundle;
import android.view.MotionEvent;
import android.view.View;
import android.content.ContentValues;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.database.Cursor;
import android.net.Uri;
import android.view.inputmethod.InputMethodManager;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import com.example.myapplication.R;
public class MainActivity4 extends AppCompatActivity{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main4);
}
@Override
public booleanonTouchEvent(MotionEventev) {
InputMethodManageri=(InputMethodManager)getSystemService(Context.INPUT_METHOD_SERVICE);
i.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
return true;
}
public void onClickAddData(View v) {
ContentValuesval= new ContentValues();
val.put(DetailsProvider.name, ((EditText) findViewById(R.id.enterName)).getText().toString());
getContentResolver().insert(DetailsProvider.URI, val);
Toast.makeText(getBaseContext(), "New Record Inserted", Toast.LENGTH_LONG).show();
}
public void onClickShowData(View v) {
// Retrieve student records
TextViewresultView= (TextView) findViewById(R.id.data);
Cursor cur = getContentResolver().query(Uri.parse("content://com.example.myapplication.Javatpoint.DetailsProvider/users"), null, null, null, null);
if(cur.moveToFirst()) {
StringBuilder st= new StringBuilder();
while (!cur.isAfterLast()) {
st.append("\n" + cur.getString(cur.getColumnIndex("id"))+ "-" + cur.getString(cur.getColumnIndex("name")));
cur.moveToNext();
}
resultView.setText(st);
}
else {
resultView.setText("No Records Found");
}
}
}
Run the application to launch the Android virtual device and make sure that the content provider is working properly.
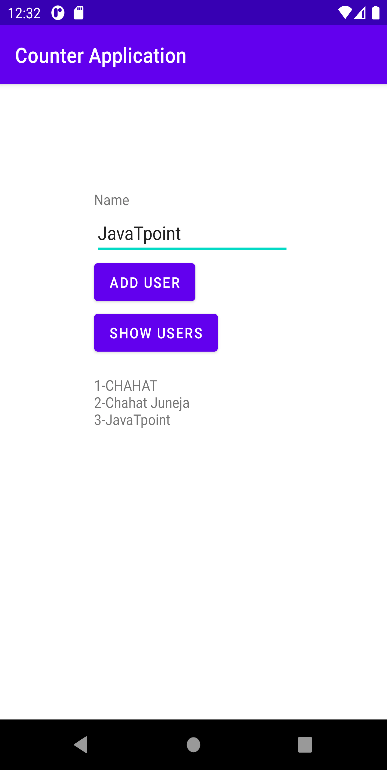
From above demonstration we learnt how to use content providers in our Android applications to store data in a centralized repo to allow other applications to access it according to the requirements.