Android TextView
Widgets are user interface (UI) elements that help users to interact with Android applications. It is one of many equipments that can be used to improvise the user interface of your application. TextView refers to a widget that displays text on the screen according to the layout, size, color, etc. set in a specific TextView. You can modify or edit the options themselves.
Structure:
public class TextView
extends View
implements ViewTreeObserver.OnPreDrawListener
Class Hierarchy:
java.lang.Object
android.view.View
android.widget.TextView
Syntax:
<Any_Layout>
.
.
<TextView>
android:Attribute1 = " attribute1 value"
android:Attribute2 = "attribute2 value"
.
android:AttributeN = "attributeN value"
</TextView>
.
.
</Any_Layout>
The layout here can be any layout such as Constraint, Relative, Linear, etc. (For more information on layout, see the article on Basic Layouts). Additionally, this documentcontainsinformation about a number of attributes in the table.
Example:
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".ExampleActivity3">
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Text View Example"
android:textColor="#151515"
android:textSize="30sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.482"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
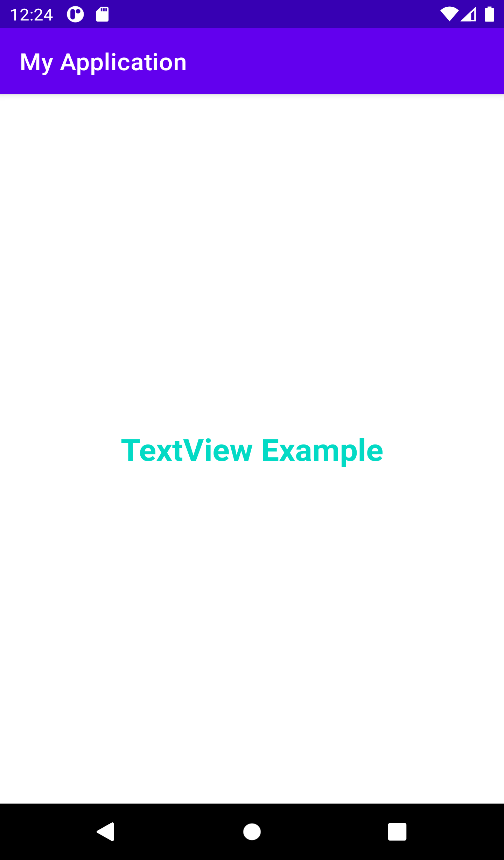
Steps for including a TextView in an Android Application:
1. The first will be to create a new Android project or open an existing project to edit it in the Android Studio. Under any scenario, there must be an activity java class file and an XML layout activity file linked to the class.
2. Open the activity layout file and inserta textview in the layout.
3. Now in the SecondActivity.java file, connect theXML layout file as follows:
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
//activity_second is the name of the XML layout file to be displayed.
}
4. Let’s change the Text displayed in the TextView and show a toast when clicked.
5. The XML layout file and the Java file codesare as follows:
activity_second.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".SecondActivity">
<TextView
android:id ="@+id/id1"
android:layout_width ="wrap_content"
android:layout_height ="wrap_content"
android:text ="Welcome To"
android:textSize ="26sp"
android:textStyle ="bold"
android:textColor ="@color/teal_200"
app:layout_constraintBottom_toBottomOf ="parent"
app:layout_constraintLeft_toLeftOf ="parent"
app:layout_constraintRight_toRightOf ="parent"
app:layout_constraintTop_toTopOf ="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
SecondActivity.java
import androidx.appcompat.app.AppCompatActivity;
import android.widget.TextView;
import android.widget.Toast;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class SecondActivity extends AppCompatActivity {
// Creating the object
publicTextView txt;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
txt= findViewById(R.id.id1);
// Changing the text of the Textview upon click
// and also show a Toast message
txt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view)
{
// Setting new text
txt.setText("Paradise");
// Showing Toast
Toast
.makeText(SecondActivity.this,
"Welcome to Paradise",
Toast.LENGTH_SHORT)
.show();
}
});
}
}
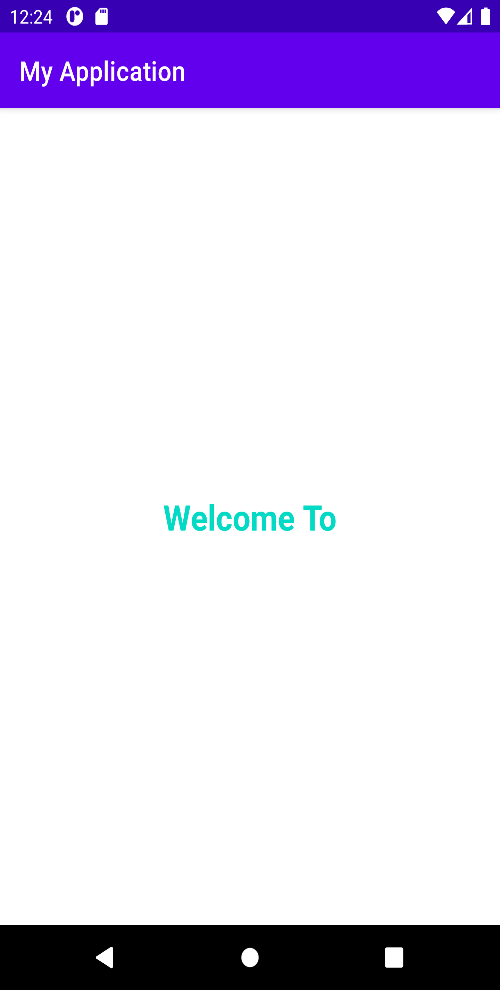
On Click |
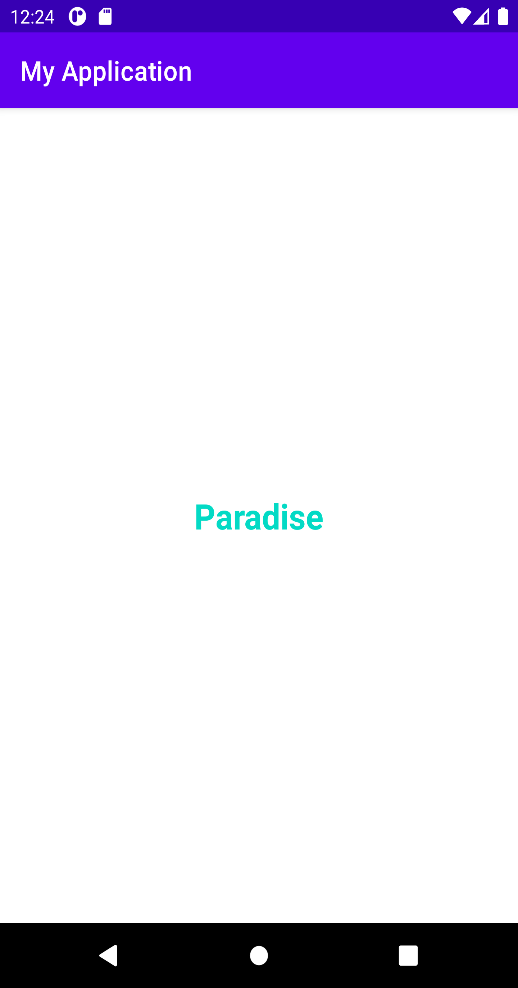
Attributes of TextView
Attributes | Description |
android:text | Sets the text of the Textview |
android:id | Assigns a distinct ID to the Textview |
android:cursorVisible | Use this property to show or hide the cursor. Default values are displayed. |
android:drawableBottom | Place an image or other graphic asset below the Textview. |
android:drawableEnd | Place an image or other graphic asset to the Textview’s end. |
android:drawableLeft | Place an image or other graphic asset to the Textview’s left. |
android:drawablePadding | Utilized to place padding to the in the Textview. |
android:autoText | Spelling errors are corrected automatically in the text of the Textview. |
android:capitalize | It automatically capitalizesthe text that the user enters in the Textview. |
android:drawableRight | Places drawable to the text’s right in a Textview. |
android:drawableStart | Places drawable to text’s start in a Textview. |
android:drawableTop | Places drawable to text’s top in a Textview. |
android:ellipsize | Utilize this property when you want the text to be ellipsiswhen the text’s length is longer than the width of Textview. |
android:ems | Changing the Textview’s width in ems. |
android:gravity | Text of the Textview can be aligned either vertically or horizontally or both. |
android:height | Utilize to change theTextview’s height. |
android:lines | Utilize to changethe height of the Textview by the number of lines. |
android:maxHeight | Change the Textview’s maximum height. |
android:minHeight | Change the Textview’s minimum height. |
android:maxLength | Change the Textview’s maximum character length. |
android:maxLines | Defines maximum lines that aTextview can contain. |
android:minLines | Defines minimum lines that a Textview can contain. |
android:maxWidth | Change the maximum width that the Textview can have. |
android:minWidth | Sets minimum width that the Textview can have. |
android:textAllCaps | Capitalize all text in Textview. |
android:textColor | Changethe text’s color. |
android:textSize | Changethe text’sfont size. |
android:textStyle | Changethe text’s style. For example, bold, italic. |
android:typeface | Changethe text typeface or font. For example,Arial, normal, sans, serif, etc |
android:width | Changes width of the TextView. |