Android ScrollView
A ScrollView is a view group that is used to create vertically scrollable views in an android application. Only a single direct child can be contained by ScrollView. For placing multiple views in a scroll view, you can create a view group like LinearLayout or ConstraintLayout as a direct child, and then you may add many other views within it. ScrollView only supports vertical scrolling, so use the HorizontalScrollView to create a display that allows horizontal scrolling.
ScrollView XML attributes
Attribute | Description |
android:fillViewport | Declares whether the content is enlarged by the ScrollView to fill the viewport. |
From View
Attributes | Description |
android:alpha | The alpha property of the view, with a value from 0 (fully transparent) to 1 (fully opaque). |
android:background | For specifying background of the view. |
android:clickable | Defines if this view will respond to click events. |
android:contentDescription | Specifies the text that briefly gives an idea about the view’s content. |
android:id | Gives an identifier name for this view, for retrieving it later with View.findViewById() or Activity.findViewById(). |
android:isScrollContainer | If the view will provide a scrolling container, add this attribute. |
android:minHeight | Specify the view’s minimum height. |
android:minWidth | Specify the view’s minimum width. |
android:onClick | The name of the method in the context of the view that is called when the view is clicked. |
android:padding | Adds the padding according to your need. |
android:scrollbars | Declares that scroll bar displays or not when scrolling. |
Demonstration
Here's a demonstration of implementing a simple ScrollView in an Android application. In the demonstration, let’s build an android application that will display text about Android using ScrollView.
1. In Android Studio, make a new project and name it as ScrollView Example. If you come across any problem in creating a new project, please refer to Android Studio Tutorial. Remember to choose the Java language.
2. Insert some strings inside the strings.xml file to display those strings in the app.
strings.xml
<resources>
<string name ="app_name">ScrollView</string>
<string name ="text_ScrollView"> What is Android?
The Android is an operating system that is most used on various mobile platforms around the world. It accounts for about 72.18% of the global market share by the end of July 2021. Companies like OpenHandsetAlliance developed the first Android that relied on custom versions of the Linux kernel and other open-source software. In the early stages of 2005, Google sponsored the project and acquired the entire company. In September 2008, the first Android devices hit the market, dominating the mobile industry, with features such as customizing, manufacturing, and customizing Android devices from large enterprises supported by the user-friendly community. As a result, the market is investigating the demand for developing Android-enabled devices with smart developers. Therefore, the Android operating system is a complete set of operating systems for various devices such as wearables, mobile phones, laptops, smart TVs, tablets, and set-top boxes.
About Android Operating System
GNU libraries are not supported, and there is no native X Windows system for them. The Linux kernel contains drivers for display, camera, flash memory, keyboard, WLAN, and sound. The Linux kernel acts as an abstraction between the hardware and the rest of the software on the smart device. It is also responsible for basic system services such as security, network stack, process management, and memory management. It is mainly developed for devices with touch displays such as smartphones, watches, and tablets. Mobile operating systems have changed a lot in the past fifteen years, from black and white feature phones to the latest smartphones, tablets, or minicomputers.
Android software was founded in Palo Alto, California in 2003.
Android is a powerful operating system that provides support to a variety of different applications on smart devices. These android applications are more user-friendly and easier to operate. The hardware is based on the ARM architecture platform which is supported by the Android software. Android is an open-source operating system, which means it is free of cost and anyone from around the world can use it. There are millions of Android applications that can help you manage your life in one way or another, which is why it is so popular in the market.
Software developers who wish to create applications for the Android operating system may download a specific version of the Android Software Development Kit (SDK) as per their needs. The SDK includes a debugger, library, simulator, some documents, code examples, and tutorials. All parties can use a graphical integrated development environment (IDE) such as Android Studio or IntelliJ to write applications using Java or Kotlin Language.
Android Features
• It provides a variety of features, such as weather information, RSS feeds (Really Simple Syndication), etc.
• It provides push notification, GPS, and SQLite storage options.
• It provides connectivity, such as Bluetooth, WiFi, GSM, CDMA, LTE, WiMax, NFC, etc.
• It provides messaging functions, such as SMS and MMS.
• It provides a web browser that uses an open-source web design engine that is linked to Google Chrome and supports HTML5 and CSS3.
</string>
</resources>
3. Insert the ScrollView and nest a TextView inside the ScrollView to display the strings that are placed in the strings.xml file.
<?xml version ="1.0" encoding ="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app ="http://schemas.android.com/apk/res-auto"
xmlns:tools ="http://schemas.android.com/tools"
android:layout_width ="match_parent"
android:layout_height ="match_parent"
tools:context =".MainActivity">
<ScrollView
android:layout_width ="match_parent"
android:layout_height ="match_parent"
android:paddingStart ="4dp"
android:paddingEnd ="4dp"
app:layout_constraintEnd_toEndOf ="parent"
app:layout_constraintStart_toStartOf ="parent"
app:layout_constraintTop_toTopOf ="parent">
<TextView
android:id ="@+id/scrolltext"
style ="@style/Theme.MyApplication"
android:layout_width ="match_parent"
android:layout_height ="wrap_content"
android:text ="@string/text_ScrollView"
android:textColor ="@color/teal_700" />
</ScrollView>
</androidx.constraintlayout.widget.ConstraintLayout>
4. There is nothing to modify in the ScrollViewActivity.java file, so keep it unchanged.
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import com.example.myapplication.R;
public class ScrollViewActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_scroll_view);
}
}
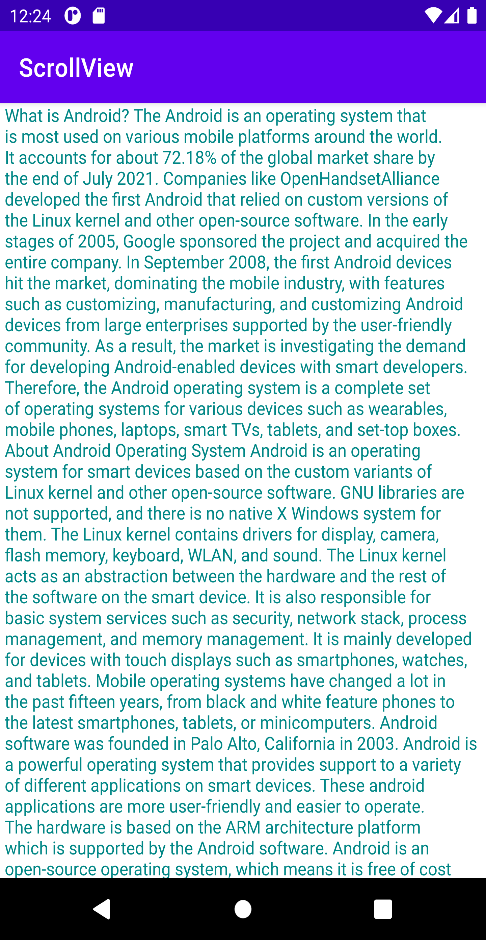
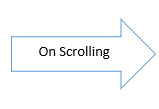
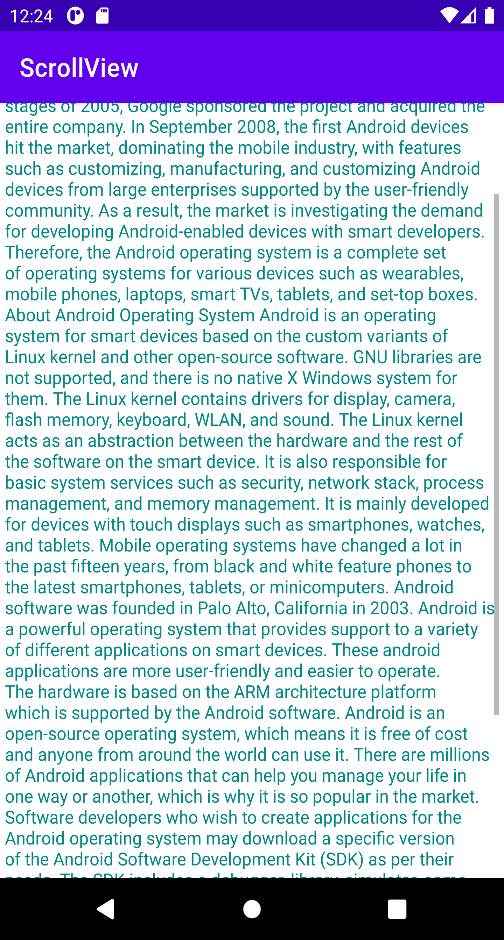