Javascript Find Object In Array
What is find() method?
It returns the value of the first element in the given array that fulfils the given testing function. However, if no values from the array are able to satisfy the testing function, then “undefined
” is returned by the find() method.
The find() method does not execute the function on empty arrays, i.e, the array with no elements.
The execution of this method does not modify the original array.
This method is supported by mostly all the browsers like Google chrome 45, safari 7.1, Microsoft Edge 12, Opera 32, Firefox 25 etc. However, it does not provide support to Internet Explorer.
The find() method can take various parameters such as:
- Callback Function
It refers to the function that executes each value in the array. It usually takes three arguments, that are:
- Element
It means the current element of the array. It is a compulsory argument.
- Index
It refers to the position(index) of the current element of the array. It is an optional argument.
- Array
It refers to the array on which the find() method was called on. It is an optional argument.
- This value
It means the value that can be passed to the function to be used as its "this" value.
However, if this parameter is empty, the value "undefined" is passed as its "this" value. It is an optional argument.
Find() method and callback function
For every index of the array, the find method executes the “Callback function” atleast once, until the “callback function” returns the “true” value.
- If it happens, the find() method immediately returns the value of that element and skips checking the remaining values.
- Else if it returns “false”, the find() method returns undefined.
Syntax:
array.find(function(currentValue, index, arr),thisValue)
Example 1:
<!DOCTYPE html>
<html>
<head>
<title>
Javascript Find object in array
</title>
</head>
<body>
<h2>JavaScript find() method in arrays</h2>
<p>The example show how the find() method returns the value of the first element in an array that passes the condition, which is provided in the a function.</p>
<p id="tcontent"></p>
<script>
const values = [3, -10, 18, -6,-9,20];
document.getElementById("tcontent").innerHTML = values.find(checkPos);
function checkPos(values) {
return values > 0;
}
</script>
<p>Here, the find() method is searching for positive numbers in the array, and returns the first positive number it encounters.</p>
</body>
</html>
Output:
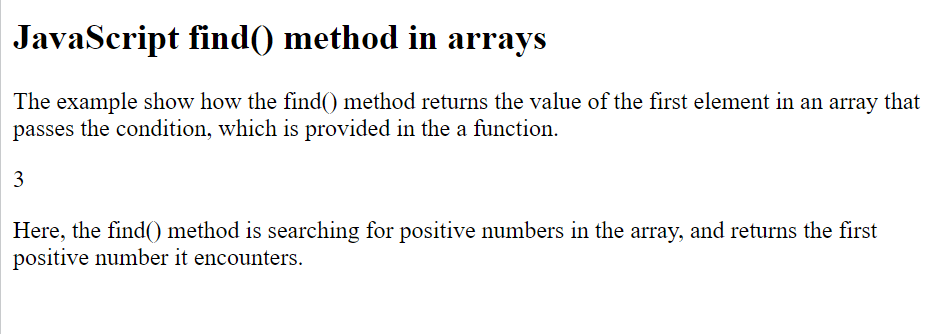
Example 2:
<!DOCTYPE html>
<html>
<head>
<title>
Javascript Find object in array
</title>
</head>
<body>
<h2>JavaScript find() method in arrays</h2>
<p>The example show how the find() method returns the value of the first element in an array that passes the condition, which is provided in the a function.</p>
<p><input type="number" id="CheckPosNum" value="0"></p>
<button onclick="myFunctiondemo()">Click here</button>
<p id="tcontent"></p>
<script>
const values = [3, -10, 18, -6,-9,20];
function checkPos(values) {
return values > document.getElementById("CheckPosNum").value;
}
function myFunctiondemo() {
document.getElementById("tcontent").innerHTML = values.find(checkPos);
}
</script>
<p>Here, the find() method is searching for positive numbers in the array, and returns the first positive number it encounters.</p>
</body>
</html>
Output:
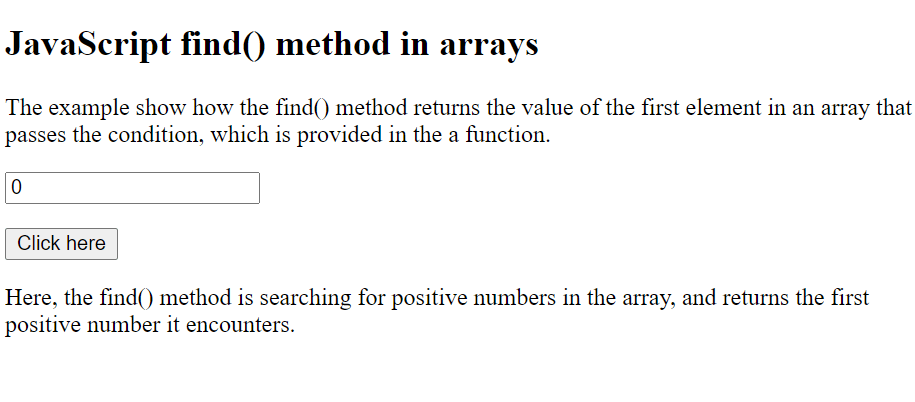
After clicking on the button, the function check the value of elements in the array with “value” provided in the input tag(specified by the user), and displays the first element fulfilling the condition,
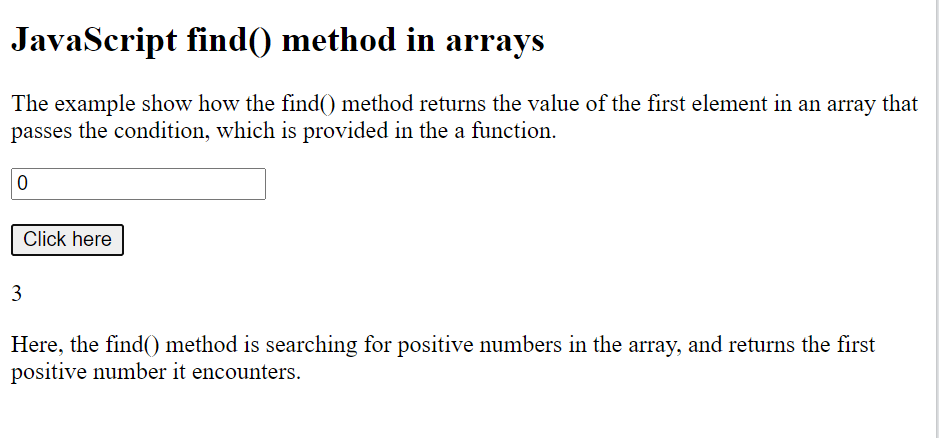
NOTE: The value in the <input> tag can be incremented or decremented by the user accordingly, through the arrow buttons, as shown below:
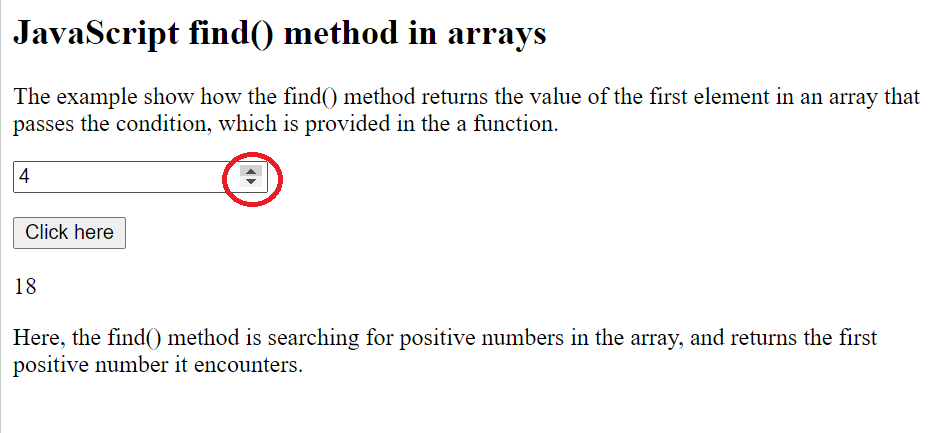