JavaScript moment date difference
Often date differences are required on the web platforms for various reasons. They can be:
- To find the duration, of course, someone is pursuing
- To find the age of a user from his birth-date
- Date customizations etc.
However, doing these calculations with the dates and time using JavaScript can become quite challenging, specifically if the database is huge. Therefore, here moment.js comes into the picture.
What is moment.js?
Moment.js is a JavaScript library. Ithelps in validating, manipulating, parsing and displaying date/time in JavaScript in a simple manner. This library allows displaying of date as per localization and in human readable format. Moment.js can be used directly inside any browser.
Wherever the user wishes to use this library, the following code should be included within the script tag:
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
Example:
To display the current date:
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<div style = "font-size:20px; color: red; " id = "currentdate"></div>
<script type = "text/JavaScript">
var val = moment().toString();
document.getElementById("currentdate").innerHTML = val;
</script>
</body>
</html>
Output:
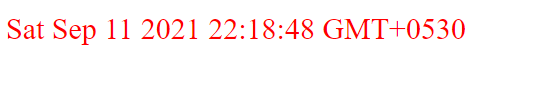
How are date-differences/durations calculated in JavaScript?
The following functions are used to find date-differences:
- moment.duration().asMilliseconds();
It is used to return the difference in dates or value in milliseconds. - moment.duration().asSeconds();
It is used to return the difference in dates or value in seconds. - moment.duration().asMinutes();
It is used to return the difference in dates or value in minutes. - moment.duration().asHours();
It is used to return the difference in dates or value in hours. - moment.duration().asDays();
It is used to return the difference in dates or value in days. - moment.duration().asWeeks();
It is used to return the difference in dates or value in weeks. - moment.duration().asYears();
It is used to return the difference in dates or value in years.
Syntax:
var duration = moment.duration(x.diff(y))
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in days</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var a = moment("2013-11-4"); //first date
var b = moment("2015-12-1"); // second date
var duration = moment.duration(b.diff(a)); // b-a
var days = duration.asDays();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + days+ " days";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in days
The difference between the dates is: 757 days
- Finding the difference between current date and given date:
- Date difference in seconds
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in seconds</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var now = moment(new Date()); //todays date(2021-9-11)
var end = moment("2021-2-6"); // another date
var duration = moment.duration(now.diff(end));
var val = duration.asSeconds();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " seconds";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in seconds
The difference between the dates is: 18831774.866 seconds
- Date difference in days
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in days</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var now = moment(new Date()); //todays date(2021-9-11)
var end = moment("2021-2-6"); // another date
var duration = moment.duration(now.diff(end));
var val = duration.asDays();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " days";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in days
The difference between the dates is: 217.96392319444445 days
- Date difference in months
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in months</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var now = moment(new Date()); //todays date(2021-9-11)
var end = moment("2021-2-6"); // another date
var duration = moment.duration(now.diff(end));
var val = duration.asMonths();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " months";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in months
The difference between the dates is: 7.161205484864318 months
- Date difference in years
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in years</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var now = moment(new Date()); //todays date(2021-9-11)
var end = moment("2018-12-16"); // another date
var duration = moment.duration(now.diff(end));
var val = duration.asYears();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " years";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in years
The difference between the dates is: 2.740550074861476 years
- Finding the difference between two given dates:
- Date difference in seconds
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in seconds</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var a = moment("2013-11-4"); //first date
var b = moment("2015-12-1"); // second date
var duration = moment.duration(b.diff(a)); // b-a
var val = duration.asSeconds();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " seconds";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in seconds
The difference between the dates is: 65404800 seconds
- Date difference in weeks
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in days</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var a = moment("2013-11-4"); //first date
var b = moment("2015-12-1"); // second date
var duration = moment.duration(b.diff(a)); // b-a
var val = duration.asWeeks();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " weeks";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in days
The difference between the dates is: 108.14285714285714 weeks
- Date difference in months
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in months</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var a = moment("2013-11-4"); //first date
var b = moment("2015-12-1"); // second date
var duration = moment.duration(b.diff(a)); // b-a
var val = duration.asMonths();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " months";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in months
The difference between the dates is: 24.871147251483603 months
- Date difference in years
Example:
<!DOCTYPE html>
<html>
<head>
<title>MomentJS Example</title>
<script type = "text/JavaScript" src = "https://MomentJS.com/downloads/moment.js"></script>
</head>
<body>
<h2>The following is an example to demonstrate the working of moment.js</h2>
<br>
<p>Getting Difference between two dates in years</p>
<br>
<p id="tcontent"></p>
</body>
<script>
console.clear();
var a = moment("2013-11-4"); //first date
var b = moment("2015-12-1"); // second date
var duration = moment.duration(b.diff(a)); // b-a
var val = duration.asYears();
document.getElementById("tcontent").innerHTML = "The difference between the dates is: " + val+ " years";
</script>
</html>
Output:
The following example demonstrates the working of moment.js
Getting Difference between two dates in years
The difference between the dates is: 2.0725956042903 years