Javascript Redirect Button
What is a redirect button?
When a button acts as a hyperlink, it is known as a redirect button. On clicking the button, it transfers the user to another page.
How to make a redirect button in JavaScript?
There are various ways to create a redirect link in JavaScript, the most common ways are using the following attributes:
- replace() attribute
It stimulates an HTTP Direct.
Syntax:
window.location.replace("url of the website/document");
Example:
<!DOCTYPE html>
<html>
<head>
<title>
Javascript redirect buttons
</title>
</head>
<body>
<h2>Javascript Redirect Button using replace() </h2>
<p>The following example demonstrates how the replace() method is used to replace the current document with a new one.</p>
<button onclick="myFunctiondemo()">Redirect Button</button>
<script>
function myFunctiondemo() {
location.replace("https://www.javatpoint.com/")
}
</script>
</body>
</html>
The above code can also be written in a slightly different way:
<!DOCTYPE html>
<html>
<head>
<title>
Javascript redirect buttons
</title>
</head>
<body>
<h2>Javascript Redirect Button using replace() </h2>
<p>The following example demonstrates how the replace() method is used to redirect the current web page to a new one.</p>
<button id="redirect">Redirect Button</button>
<script type="text/javascript">
document.getElementById("redirect").onclick = function () {
location.replace("https://www.javatpoint.com/")
};
</script>
</body>
</html>
Output:
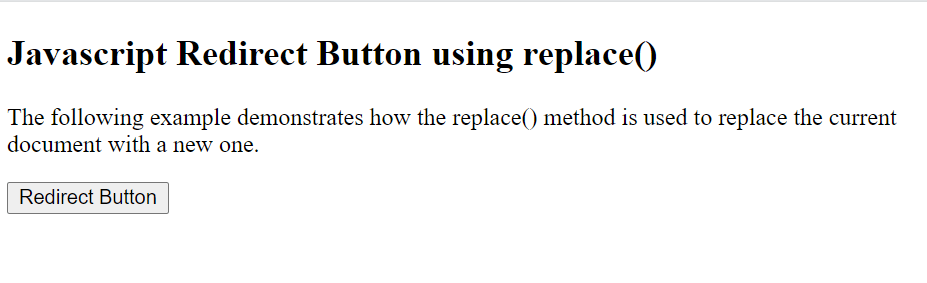
After clicking the button, the web page is now redirected to the Javatpoint website,
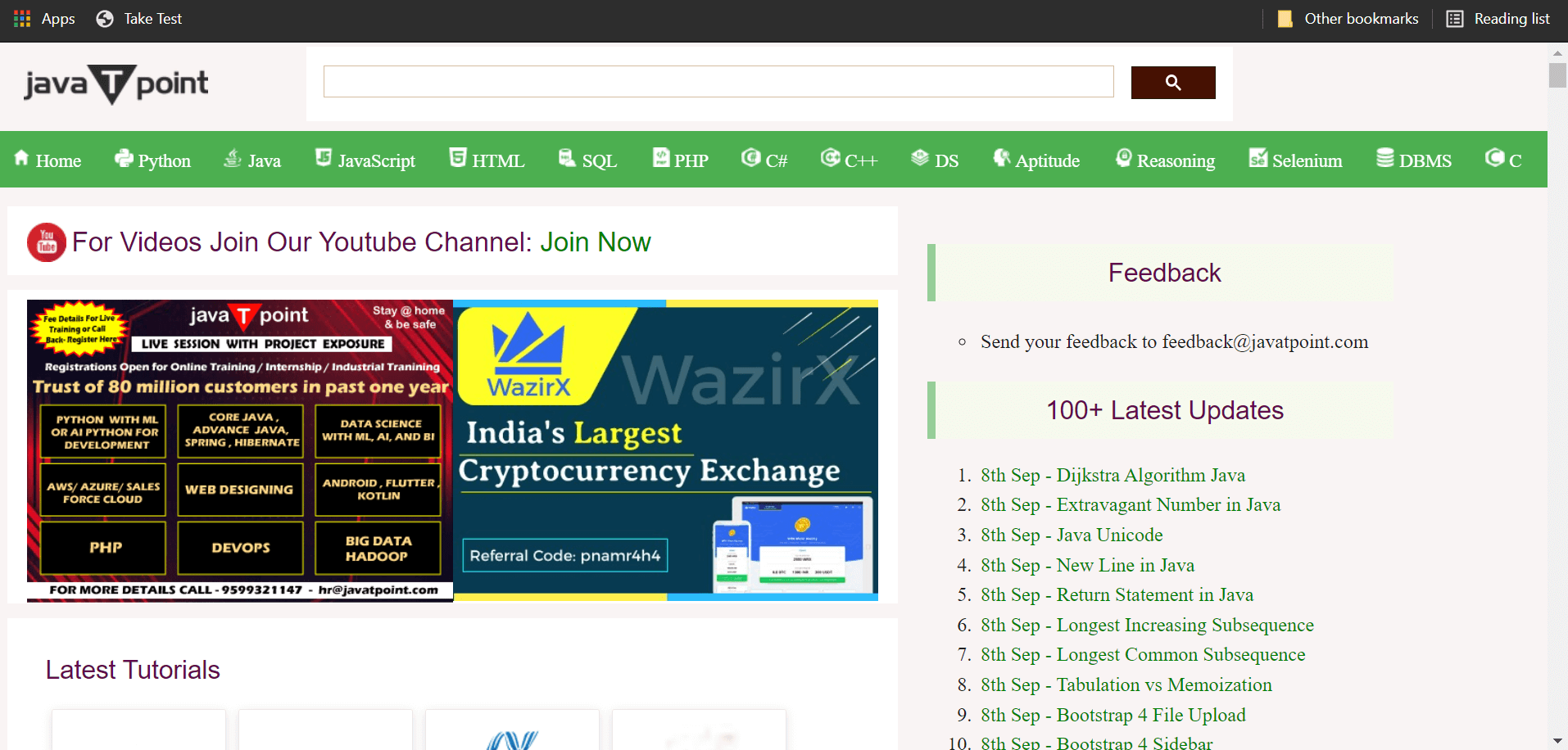
- href attribute:
It stimulates on a mouse click.
Syntax:
window.location.href = "url of the website/document";
Example:
<!DOCTYPE html>
<html>
<head>
<title>
Javascript redirect buttons
</title>
</head>
<body>
<h2>Javascript Redirect Button using href attribute</h2>
<p>The following example demonstrates how the href() attribute is used to redirect the current web page to a new one.</p>
<button onclick="myFunctiondemo()">Redirect Button</button>
<script>
function myFunctiondemo() {
location.href = ("https://www.javatpoint.com/";
}
</script>
</body>
</html>
The above code can also be written in a slightly different way:
<!DOCTYPE html>
<html>
<head>
<title>
Javascript redirect buttons
</title>
</head>
<body>
<h2>Javascript Redirect Button using href attribute</h2>
<p>The following example demonstrates how the href() attribute is used to redirect the current web page to a new one.</p>
<button id="redirect">Redirect Button</button>
<script type="text/javascript">
document.getElementById("redirect").onclick = function () {
location.href = ("https://www.javatpoint.com/");
};
</script>
</body>
</html>
Output:
Initially, the screen looks like this:
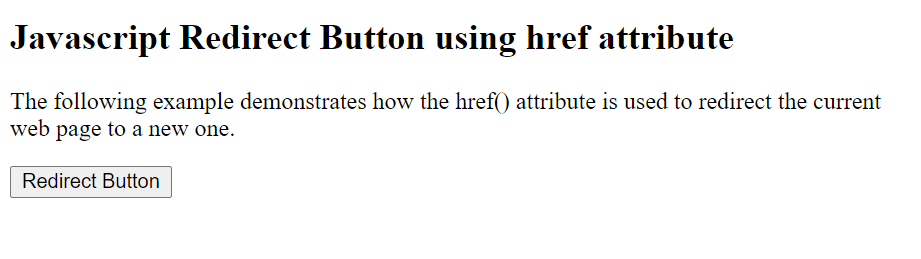
After clicking the button, the web page is now redirected to the Javatpoint website,
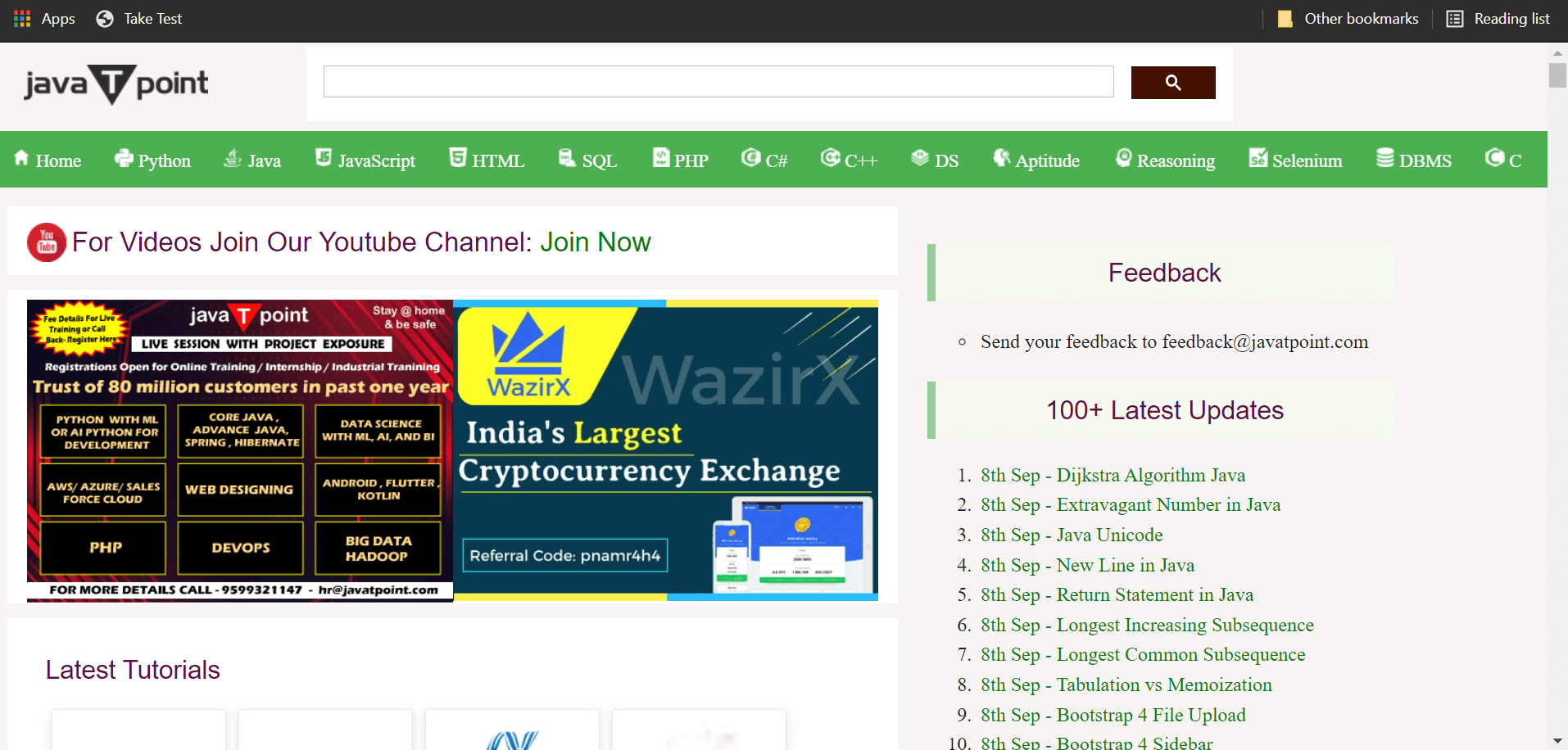
What is the difference between replace() and href?
The major difference between href and replace, is that replace() can
remove the URL of the current document or the current website from the history. It means that it is impossible navigate back to the original document, using the "back" button.
NOTE: Tags like the anchor tag <a> can also be used to create redirect buttons. However, no JavaScript is required in it.