JavaScript focus on input
In JavaScript, the elements can be focused using either focus() method or onfocus() event.
What is focus() method?
When we want to focus on an element (if it can be focused) or basically highlight it, the focus() method is used.
This method is supported by mostly all the browsers like Google chrome, safari, Microsoft Edge/Internet Explorer, Oracle, Firefox etc.
NOTE: This method takes no parameters and has no return value as well.
Syntax:
element.focus()
Example:
<!DOCTYPE html>
<html>
<body>
Enter text here: <input type="text" id="tcontent">
<p>This is the example to show a how user can use focus method to an input element.</p>
<button type="button" onclick="myFunctiondemo()">Focus</button>
<script>
function myFunctiondemo() {
document.getElementById("tcontent").focus();
}
</script>
</body>
</html>
Output:
Intially,
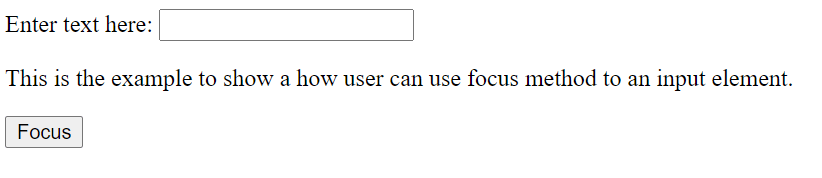
After the button has been selected, the text box gets highlighted
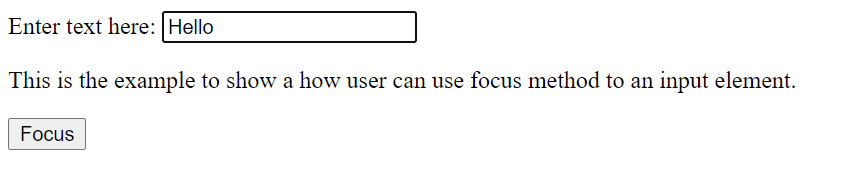
What is onfocus() event?
Whenever an element gets focus, the onfocus() event occurs.
The <input>, <select> and <a> use the onfocus() event commonly.
The onfocus() event can be used with all HTML tags expect for: <br>, <head>, <html>, <iframe>, <meta>, <param>, <script>, <style>, and <title> tags.
Similar to focus() method, the onfocus event is also supported by mostly all the browsers like Google chrome, safari, Microsoft Edge/Internet Explorer, Oracle, Firefox etc.
The input element and onfocus event
An input field is defined by the input tag where the user can enter data. The input tag element can be used to display several types of properties like radio buttons, text fields, checkboxes, dates, email Ids, passwords etc.
So, the onfocus() event or the focus method helps to set focus on the specified input element. The focused element helps in determining that which element will receive keyboard inputs and similar events by default.
The onfocus event can be used commonly in three ways:
- In HTML,
Syntax:
<element onfocus= functionname>
<script>
function functionname(){
//definition of the function
}
</script>
Example:
<!DOCTYPE html>
<html>
<body>
<p>This is the example to show a user can use "onfocus" event to an input element.</p>
Enter text here: <input type="text" id="tcontent" onfocus="myFunctiondemo()">
<script>
function myFunctiondemo() {
document.getElementById("tcontent").style.backgroundColor = "lightblue";
}
</script>
</body>
</html>
Output:
Initially, the input element looks like this,
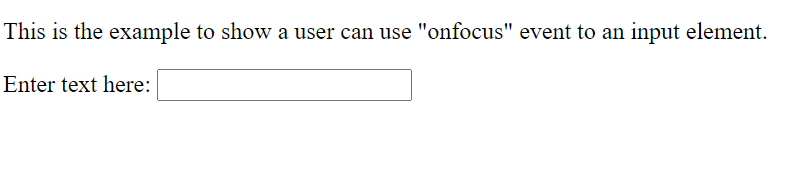
On focusing (selecting the input text-box):
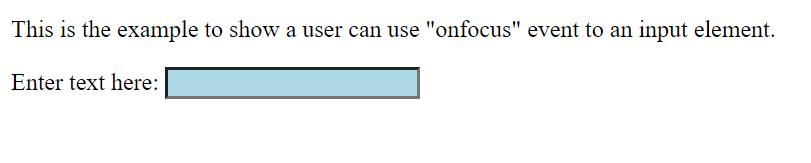
- In Javascript
Syntax:
<script>
object.onfocus = function(){functionname};
function functionname(){
//definition of the function
}
</script>
Example:
<!DOCTYPE html>
<html>
<body>
<p>This is the example to show a user can use "onfocus" event to an input element.</p>
Enter text here: <input type="text" id="tcontent">
<script>
document.getElementById("tcontent").onfocus = function() {myFunctiondemo()};
function myFunctiondemo() {
document.getElementById("tcontent").style.backgroundColor = "pink";
}
</script>
</body>
</html>
Output:
After the input element is focused (the text box is selected),
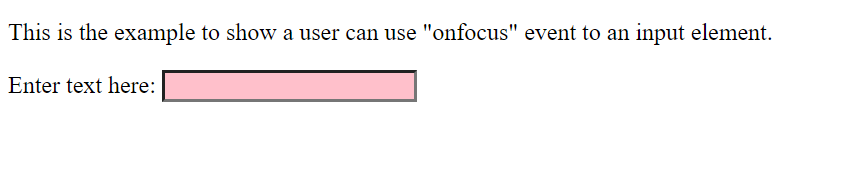
- Using addEventListener() method in JavaScript
Syntax:
<script>
object.addEventListener("focus", functionname);
function functionname(){
//definition of the function
}
</script>
Example:
<!DOCTYPE html>
<html>
<body>
<p>This is the example to show a user can use "onfocus" event to an input element.</p>
Enter text here: <input type="text" id="tcontent">
<script>
document.getElementById("tcontent").addEventListener("focus", myFunctiondemo);
function myFunctiondemo() {
document.getElementById("tcontent").style.backgroundColor = "yellow";
}
</script>
</body>
</html>
Output:
After the input element is focused (the text box is selected),

NOTE: Internet Explorers and older versions do not support the method of addEventListener().
How to remove focus from the element?
- By using the blur() method
Syntax:
element.blur()
Example:
<!DOCTYPE html>
<html>
<body>
Enter text here: <input type="text" id="tcontent">
<p>This is the example to show how a user can use blur method to an input element.</p>
<button type="button" onclick="myFunctiondemo()">Lose focus</button>
<script>
function myFunctiondemo() {
document.getElementById("tcontent").blur();
}
</script>
</body>
</html>
Output:
When the text box is highlighted initially,

After the button has been selected, the text box loses focus
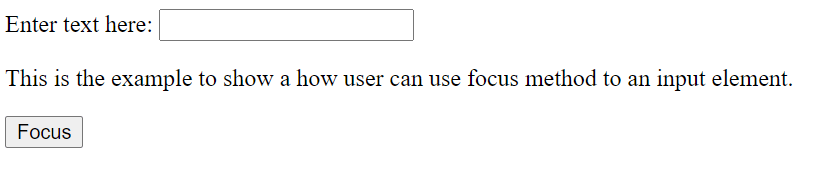
- By using the onblur() event
Syntax:
<element onblur= “functionname()”>
Example:
<!DOCTYPE html>
<html>
<body>
<p>This is the example to show a user can use "onblur" event to an input element.</p>
Enter text here: <input type="text" id="tcontent" onblur="myFunctiondemo()">
<script>
function myFunctiondemo() {
alert("Input field has lost focus.");
}
</script>
</body>
</html>
Output:
After the data has been entered in the input element, if the user clicks anywhere outside the field, it loses focus and an alert appears,
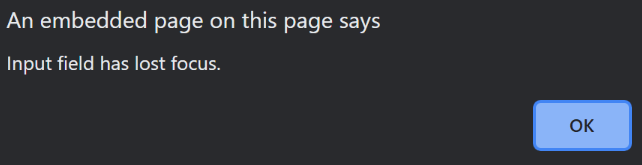