How to add column in table in SQL ?
How to add column in table in SQL
Introduction
- To add a column in already created table, one needs to use the ALTER command along with the ADD clause.
- If in the query, it is not specified where the new column is to be added, then by default it will be added as a last column.
- One can also add a new column at the first or even after a specific column of an already created table.
- You can add one column or more than one columns at a time using a single SQL query.
(A). Adding a new column at the last of an existing table
Syntax:
ALTER TABLE tablename ADD (ColumnName datatype);
where,
- Tablename is the name of an already existing table to which you have to add a new column.
- Column_name is the name of the column which is to be added to an already existing table.
Example:
First, we will create a database with name “studentdb”. Then in that database we will create a table “student” and insert records into the table. We will consider the same database and also the same table for subsequent examples.
Now, we will add a new column ‘City’ to an existing table.
mysql> USE studentdb; Database changed mysql> SELECT *FROM student; +---------+-----------+-----------+-------------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | +---------+-----------+-----------+-------------+ | 1 | Prajakta | 101 | DBMS | | 2 | Shweta | 102 | CN | | 3 | Nikita | 103 | OS | | 4 | Ankita | 104 | C | +---------+-----------+-----------+-------------+ 4 rows in set (0.00 sec) mysql> ALTER TABLE student ADD (City VARCHAR(20)); Query OK, 4 rows affected (0.29 sec) Records: 4 Duplicates: 0 Warnings: 0 mysql> SELECT *FROM student; +---------+-----------+-----------+-------------+------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | City | +---------+-----------+-----------+-------------+------+ | 1 | Prajakta | 101 | DBMS | NULL | | 2 | Shweta | 102 | CN | NULL | | 3 | Nikita | 103 | OS | NULL | | 4 | Ankita | 104 | C | NULL | +---------+-----------+-----------+-------------+------+ 4 rows in set (0.00 sec)
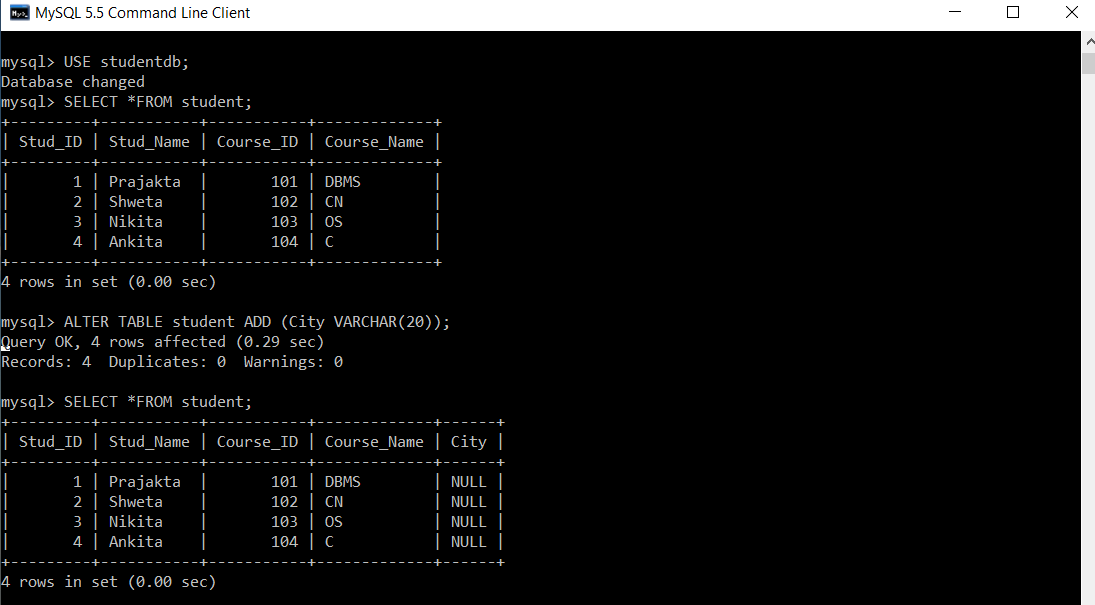
New column ‘City’ is added to an existing student table. Since, in the query we haven’t specified where it is to be added so by default it is added as the last column.
(B)Adding more than one column to an existing table
Syntax:
ALTER TABLE tablename ADD (ColumnName1 datatype, ColumnName2 datatype);
Example:
Now, we will add two new columns ‘City’ and ‘Marks’ to an existing table using a single query.
mysql> USE studentdb;
Output:
Database changed mysql> SELECT *FROM student; +---------+-----------+-----------+-------------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | +---------+-----------+-----------+-------------+ | 1 | Prajakta | 101 | DBMS | | 2 | Shweta | 102 | CN | | 3 | Nikita | 103 | OS | | 4 | Ankita | 104 | C | +---------+-----------+-----------+-------------+ 4 rows in set (0.00 sec) mysql> ALTER TABLE student ADD (City VARCHAR(20),Marks INT); Query OK, 4 rows affected (0.40 sec) mysql> SELECT *FROM student;
Output:
+---------+-----------+-----------+-------------+------+-------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | City | Marks | +---------+-----------+-----------+-------------+------+-------+ | 1 | Prajakta | 101 | DBMS | NULL | NULL | | 2 | Shweta | 102 | CN | NULL | NULL | | 3 | Nikita | 103 | OS | NULL | NULL | | 4 | Ankita | 104 | C | NULL | NULL | +---------+-----------+-----------+-------------+------+-------+ 4 rows in set (0.00 sec)

New columns ‘City’ and ‘Marks’ are added to an existing student table. Since, in the query we haven’t specified where it is to be added so by default both the columns are added at the last using a single query.
(C) Adding a column at the first position of an existing table
Syntax:
ALTER TABLE tablename ADD ColumnName datatype FIRST;
Example: Now, we will add a new column ‘Sr_No’ to an existing table as a first column.
mysql> USE studentdb;
Output:
Database changed mysql> SELECT *FROM student; +---------+-----------+-----------+-------------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | +---------+-----------+-----------+-------------+ | 1 | Prajakta | 101 | DBMS | | 2 | Shweta | 102 | CN | | 3 | Nikita | 103 | OS | | 4 | Ankita | 104 | C | +---------+-----------+-----------+-------------+ 4 rows in set (0.00 sec) mysql> ALTER TABLE student ADD Sr_No INT FIRST;
Output:
Query OK, 4 rows affected (0.24 sec) Records: 4 Duplicates: 0 Warnings: 0 mysql> SELECT *FROM student;
Output:
+-------+---------+-----------+-----------+-------------+ | Sr_No | Stud_ID | Stud_Name | Course_ID | Course_Name | +-------+---------+-----------+-----------+-------------+ | NULL | 1 | Prajakta | 101 | DBMS | | NULL | 2 | Shweta | 102 | CN | | NULL | 3 | Nikita | 103 | OS | | NULL | 4 | Ankita | 104 | C | +-------+---------+-----------+-----------+-------------+ 4 rows in set (0.00 sec)

New column ‘Sr_No’ is added to an existing student table. Since, in the query we have specified ‘FIRST’ keyword so ‘Sr_No’ is added as a first column.
(D) Adding a new column after a specific column of an existing table
Syntax:
ALTER TABLE tablename ADD ColumnName datatype AFTER column_name;
Example:
Now, we will add a new column ‘Marks’ to an existing table after the ‘Course_Name’ .
mysql> USE studentdb;
Output:
Database changed mysql> SELECT *FROM student;
Output:
+---------+-----------+-----------+-------------+------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | City | +---------+-----------+-----------+-------------+------+ | 1 | Prajakta | 101 | DBMS | NULL | | 2 | Shweta | 102 | CN | NULL | | 3 | Nikita | 103 | OS | NULL | | 4 | Ankita | 104 | C | NULL | +---------+-----------+-----------+-------------+------+ 4 rows in set (0.00 sec) mysql> ALTER TABLE student ADD Marks INT AFTER Course_Name;
Output:
Query OK, 4 rows affected (0.28 sec) Records: 4 Duplicates: 0 Warnings: 0
mysql> SELECT *FROM student;
Output:
+---------+-----------+-----------+-------------+-------+------+ | Stud_ID | Stud_Name | Course_ID | Course_Name | Marks | City | +---------+-----------+-----------+-------------+-------+------+ | 1 | Prajakta | 101 | DBMS | NULL | NULL | | 2 | Shweta | 102 | CN | NULL | NULL | | 3 | Nikita | 103 | OS | NULL | NULL | | 4 | Ankita | 104 | C | NULL | NULL | +---------+-----------+-----------+-------------+-------+------+ 4 rows in set (0.00 sec)

New column ‘Marks’ is added to an existing student table. Since, in the query we have specified ‘AFTER’ keyword with the column name after which the new column is to be added. Hence, ‘Marks’ is added after ‘Course_Name’.