SQL Order BY LIMIT
SQL is a common computer language used to handle relational databases. It has been around for decades and is the most widely used language for interacting with databases. The capacity to get data from a database and arrange it based on certain criteria is one of the most important components of SQL.
The ORDER BY and LIMIT clauses are two crucial SQL features that aid in the organisation and management of data extraction from a database. In this post, we'll look at some of these two clauses and see how they work.
In SQL, the ORDER BY phrase can be used to arrange data in a table depending on one or more columns. Sorting could be done either ascending or descending. The sorting order is ascending by default, but it may be altered to descendant by using the DESC keyword. The following is the syntax for the ORDER BY clause:
Syntax:
SELECT column1, column2, column3
FROM table_name
ORDER BY column_name ASC/DESC;
Columns 1, 2, and 3 are the columns we wish to extract from table name, and column name is the column on which the sorting must be executed. The ASC/DESC keyword can be used to determine the sorting order.
The LIMIT clause in SQL is used to restrict the number of rows returned from a table. To extract a fixed amount of rows after sorting the data, the LIMIT clause is frequently used in combination with the ORDER BY clause. The LIMIT clause has the following syntax:
Syntax:
SELECT column1, column2, column3
FROM table_name
ORDER BY column_name
LIMIT number_of_rows;
The number of rows parameter specifies the number of rows to fetch from the table.
Consider the following situation to better understand how the ORDER BY and LIMIT clauses work:
Consider the table "employees," which holds information about a company's employees. The columns in the table are as follows:
id, name, age, salary, department
Assume we want to find the top ten employees in the organisation with the highest salaries. We may accomplish this by running the SQL query:
Program Code:
SELECT name, salary
FROM employees
ORDER BY salary DESC
LIMIT 10;
The query extracts the name and salary fields from the workers table, arranges the data in decreasing order based on the salary column, and returns just the top ten rows.
It is worth mentioning that not all relational databases support the LIMIT clause. The analogous clause in Microsoft SQL Server, for example, is named TOP, and the syntax is somewhat different. The similar phrase in Oracle is named ROWNUM, and the syntax is different. As a result, before using the LIMIT clause, be sure to read the documentation for the database you're using.
Sorting data in increasing order by a specified column is a typical use case for the ORDER BY clause. For instance, if we wish to extract the names of all employees in the "employees" database, ordered by age in ascending order, we may use the query:
Program Code:
SELECT name, age
FROM employees
ORDER BY age ASC;
Output:
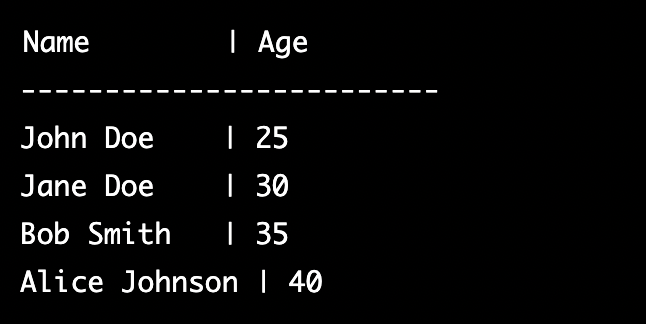
Another use is sorting data by many columns. For example, if we wish to extract the names and salaries of all workers in the "employees" database, ordered by department in increasing order and then followed by salary in descending order, we may use the following query:
Program Code:
SELECT name, salary, department
FROM employees
ORDER BY department ASC, salary DESC;
Output:
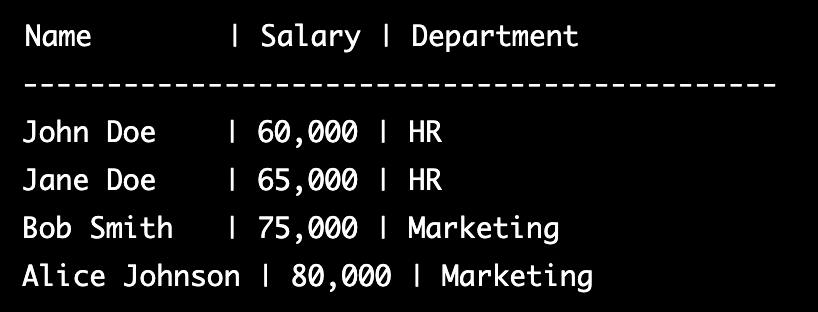
The LIMIT clause may also be used to obtain a certain range of rows from a table. For instance, if we wish to extract the names and salaries of the second through fifth best paid employees in the "employees" database, we may use the following query:
Program Code:
SELECT name, salary
FROM employees
ORDER BY salary DESC
LIMIT 1, 4;
Output:
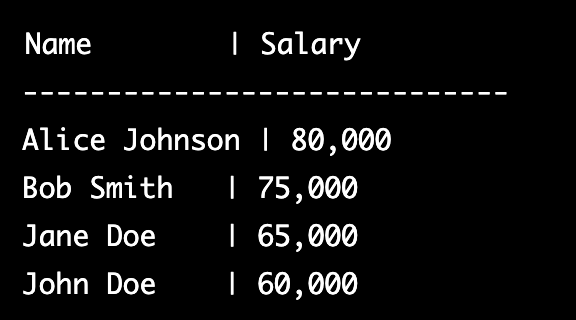
The first parameter in the LIMIT clause, 1, indicates the first row to be retrieved, and the second value, 4, specifies the number of rows to return.
This indicates that the query will return four rows, beginning with the employee with the second-highest salary.
It's worth noting that the ORDER BY clause may be used in subqueries as well. For instance, if we wish to find out who the highest-paid person in each department is, we may perform the following query:
Program Code:
SELECT name, salary, department
FROM employees
WHERE (department, salary) IN (SELECT department, MAX(salary)
FROM employees
GROUP BY department)
ORDER BY department;
Output:
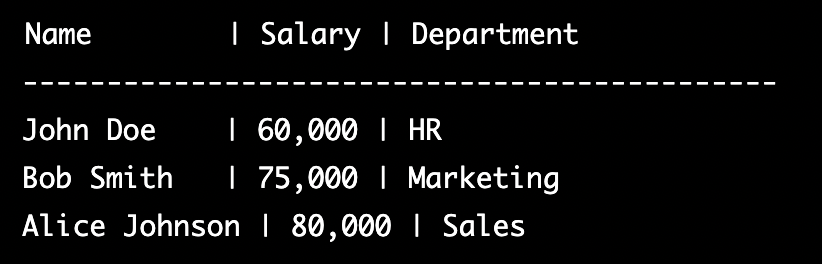
The subquery returns the department as well as the employee with the highest salary within every department, while the main query returns the name, salary, and department of an employee with the highest salary within every department. The results are then ranked in ascending order by department.
Conclusion:
Finally, the ORDER BY LIMIT clauses in SQL are key aspects that aid in accessing and arranging data from a database.
The ORDER BY phrase can be used to sort data based on certain criteria, while the LIMIT clause can be used to restrict the number of rows returned from a table.
Understanding these clauses and their usage is a necessary step in comprehending SQL.