SQL Left Join
The SQL Left Join query displays all the records from the table and displays similar records from the right table. The query displays zero records if it doesn’t find any similar records. If similar records are not found in the right table, the NULL keyword is returned in the row of the left table.
Syntax of the SQL Left Join
SELECT Column_Name1, Column_Name2, Column_Name3, Column_Name4 FROM Table_1 LEFT JOIN Table_2 ON Expression;
Column_Name1, Column_Name2, Column_Name3, Column_Name4 columns from both the table.
Left Table: Table_1, Right Table: Table_2.
Expression: Condition expression with ON clause.
This conditional expression is a comparison condition of comparison operators and logical operators of SQL. Remember that Comparison Operators are >
, <
, >=
, <=
, =
, !=
, LIKE
, BETWEEN
, etc., and Logical Operators are AND
, OR
, and NOT
.
There is one too many relationships between the Student and the Course table. One to Many relationships means that one Student can apply for one course or more than one course. When we execute the SQL Left Join query on these tables on Students_Id and Course_Id, all the records of the student table are displayed in the result set with similar records in the Course records.
Students who have not applied for any courses will be in the result set with the NULL keyword from the right side table.
Example of Left Join in Structured Query Language:
Table Number 1: Student
Student_Id | Student_Name | City | Age |
1 | Pratik Srivastav | Pune | 23 |
2 | Utkarsh Rokade | Mumbai | 22 |
3 | Sourabh Chougale | Nashik | 23 |
4 | Prateek Zimbre | Pune | 24 |
5 | Sakshi Patil | Aurangabad | 22 |
6 | Shruti Sharma | Mumbai | 21 |
7 | Pranoti Shende | Aurangabad | 23 |
8 | Harshada Dhanwat | Nashik | 24 |
9 | Tejas Bairagi | Nashik | 21 |
10 | Nikhil Patil | Pune | 24 |
11 | Samaira Sharma | Mumbai | 22 |
12 | Anushka Sen | Aurangabad | 23 |
Table Number 2: Course
Course_Id | Course_Name | Student_Id | Duration |
11 | Cloud Computing | 2 | 3 |
12 | SQL Database | 1 | 1 |
13 | Advance Java | 4 | 3 |
14 | Data Structures | 7 | 6 |
15 | AWS | 8 | 2 |
16 | Angular Js | 10 | 3 |
17 | Oracle Integration Cloud | 11 | 6 |
18 | Python | 6 | 1 |
19 | ReactJs | 1 | 3 |
20 | Computing | 6 | 4 |
Example 1: Execute a left joins query on the Student and Course table name.
SELECT S.Student_Id, S.Student_Name, S.City, S.Age, C.Course_Id, C.Course_Name, C.Duration FROM Student S LEFT JOIN Course C ON S.Student_Id = C.Student_Id;
The above left join query joins the employees' table and course table and retrieve the data from the tables where S.Student_Id = C.Student_Id.
The output of the above query is as follows:
Student_Id | Student_Name | City | Age | Course_Id | Course_Name | Duration |
1 | Pratik Srivastav | Pune | 23 | 12 | SQL Database | 1 |
1 | Pratik Srivastav | Pune | 23 | 19 | ReactJs | 3 |
2 | Utkarsh Rokade | Mumbai | 22 | 11 | Cloud Computing | 3 |
3 | Sourabh Chougale | Nashik | 23 | NULL | NULL | NULL |
4 | Prateek Zimbre | Pune | 24 | 13 | Advance Java | 3 |
5 | Sakshi Patil | Aurangabad | 22 | NULL | NULL | NULL |
6 | Shruti Sharma | Mumbai | 21 | 18 | Python | 1 |
6 | Shruti Sharma | Mumbai | 21 | 20 | Computing | 4 |
7 | Pranoti Shende | Aurangabad | 23 | 14 | Data Structures | 6 |
8 | Harshada Dhanwat | Nashik | 24 | 15 | AWS | 2 |
9 | Tejas Bairagi | Nashik | 21 | NULL | NULL | NULL |
10 | Nikhil Patil | Pune | 24 | 16 | Angular Js | 3 |
11 | Samaira Sharma | Mumbai | 22 | 17 | Oracle Integration Cloud | 6 |
12 | Anushka Sen | Aurangabad | 23 | NULL | NULL | NULL |
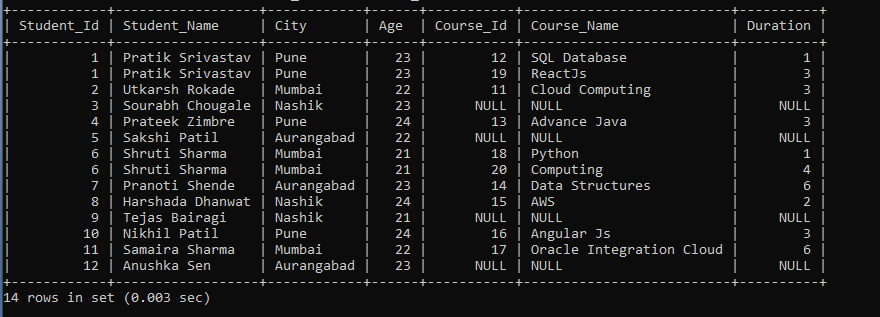
Example 2: Execute a left join query on the Student and Course table name using the WHERE Clause.
SELECT S.Student_Id, S.Student_Name, S.City, S.Age, C.Course_Id, C.Course_Name, C.Duration FROM Student S LEFT JOIN Course C ON S.Student_Id = C.Student_Id WHERE City IN ('Pune', 'Mumbai', 'Aurangabad');
The above left join query joins the employees' table and manager table and retrieve the data from the tables where S.Student_Id = C.Student_Id. The Student records show students who reside in the 'Pune', 'Mumbai' and 'Aurangabad' cities. We have applied the WHERE clause condition on the City of the Student table.
The output of the above query is as follows:
Student_Id | Student_Name | City | Age | Course_Id | Course_Name | Duration |
1 | Pratik Srivastav | Pune | 23 | 12 | SQL Database | 1 |
1 | Pratik Srivastav | Pune | 23 | 19 | ReactJs | 3 |
2 | Utkarsh Rokade | Mumbai | 22 | 11 | Cloud Computing | 3 |
4 | Prateek Zimbre | Pune | 24 | 13 | Advance Java | 3 |
5 | Sakshi Patil | Aurangabad | 22 | NULL | NULL | NULL |
6 | Shruti Sharma | Mumbai | 21 | 18 | Python | 1 |
6 | Shruti Sharma | Mumbai | 21 | 20 | Computing | 4 |
9 | Tejas Bairagi | Nashik | 21 | NULL | NULL | NULL |
10 | Nikhil Patil | Pune | 24 | 16 | Angular Js | 3 |
11 | Samaira Sharma | Mumbai | 22 | 17 | Oracle Integration Cloud | 6 |
12 | Anushka Sen | Aurangabad | 23 | NULL | NULL | NULL |
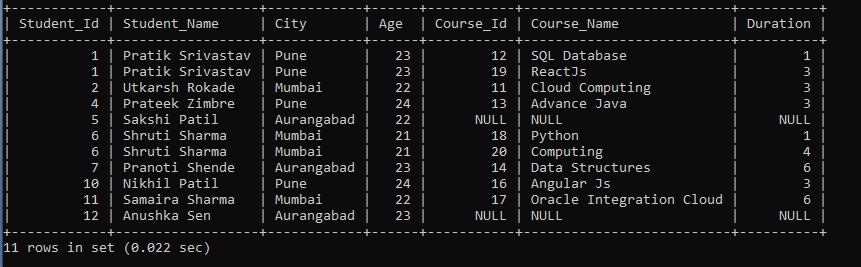
Example 3: Execute a left join query on the Student and Course table name using the ORDER BY Clause.
SELECT S.Student_Id, S.Student_Name, S.City, S.Age, C.Course_Id, C.Course_Name, C.Duration FROM Student S LEFT JOIN Course C ON S.Student_Id = C.Student_Id ORDER BY Student_Name;
The above left join query joins the employees' table and manager table and retrieves the data from the tables where S.Student_Id = C.Student_Id and the Student's records will display in the ascending order as we used the ORDER BY clause in the query.
The output of the above query is as follows:
Student_Id | Student_Name | City | Age | Course_Id | Course_Name | Duration |
12 | Anushka Sen | Aurangabad | 23 | NULL | NULL | NULL |
8 | Harshada Dhanwat | Nashik | 24 | 15 | AWS | 2 |
10 | Nikhil Patil | Pune | 24 | 16 | Angular Js | 3 |
7 | Pranoti Shende | Aurangabad | 23 | 14 | Data Structures | 6 |
4 | Prateek Zimbre | Pune | 24 | 13 | Advance Java | 3 |
1 | Pratik Srivastav | Pune | 23 | 12 | SQL Database | 1 |
1 | Pratik Srivastav | Pune | 23 | 19 | ReactJs | 3 |
5 | Sakshi Patil | Aurangabad | 22 | NULL | NULL | NULL |
11 | Samaira Sharma | Mumbai | 22 | 17 | Oracle Integration Cloud | 6 |
6 | Shruti Sharma | Mumbai | 21 | 18 | Python | 1 |
6 | Shruti Sharma | Mumbai | 21 | 20 | Computing | 4 |
3 | Sourabh Chougale | Nashik | 23 | NULL | NULL | NULL |
9 | Tejas Bairagi | Nashik | 21 | NULL | NULL | NULL |
2 | Utkarsh Rokade | Mumbai | 22 | 11 | Cloud Computing | 3 |
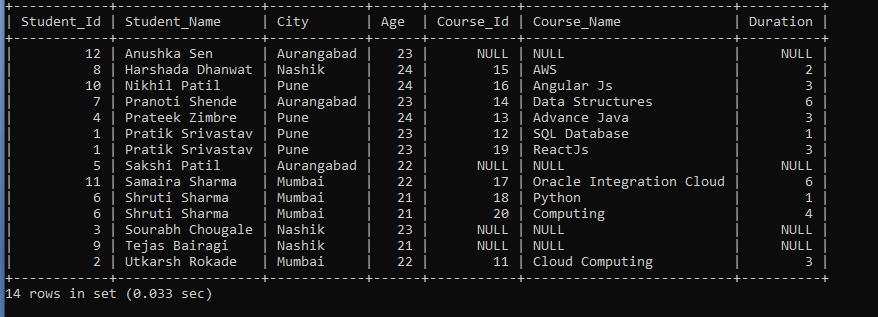