SQL SELECT Statement
The SQL SELECT statement is used to retrieve the data from the tables. We can also retrieve the selected records from the table using the query condition. The SQL SELECT statement is also used to retrieve the selected records from the selected column name.
We use the WHERE clause in the SELECT query to retrieve the selected records from the table by applying the condition. With the WHERE clause, we can use the comparison, arithmetic, bitwise, and logical operators.
The first syntax of the SELECT statement is as follows:
SELECT * FROM Table_Name;
The asterisk (*) symbol retrieves all the records from the table without applying any conditions in the query.
The second syntax of the SELECT statement is as follows:
SELECT Column_Name_1, Column_Name_2, Column_Name_3, Column_Name_4, Column_Name_5 FROM Table_Name;
The above second syntax is used to retrieve records from the specified columns in the query table.
The third syntax of the SELECT statement with the WHERE Clause is as follows:
SELECT * FROM Table_Name WHERE conditions;
In the third syntax, we used the WHERE clause in the query to retrieve or display selected records from the table by applying the conditions in the query.
Let’s understand the SQL SELECT statement with the help of examples.
Examples of SQL SELECT Statement
Consider the already existing table, which has the following data:
Table Name:- Diploma_Student
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202111 | Vaishnavi Patil | 94 | 91 | 88 | 85 | 95 | 92 | 91 | 6 |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202113 | Yash Dhull | 90 | 88 | 94 | 87 | 85 | 90 | 89 | 3 |
202114 | Sonali Patole | 95 | 90 | 92 | 88 | 92 | 90 | 91 | 4 |
202115 | Axar Patel | 85 | 80 | 82 | 86 | 92 | 84 | 85 | 1 |
202116 | Meena Mishra | 78 | 75 | 80 | 74 | 85 | 77 | 78 | 3 |
202117 | Mahesh Kumbhar | 75 | 80 | 75 | 78 | 80 | 76 | 77 | 5 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202119 | Sopan Bhore | 70 | 68 | 75 | 75 | 80 | 80 | 75 | 2 |
202220 | Prajwal Lokhande | 80 | 85 | 85 | 75 | 78 | 80 | 81 | 4 |
202221 | Anuja Wanare | 85 | 88 | 86 | 82 | 84 | 85 | 85 | 7 |
202222 | Venkatesh Iyer | 90 | 89 | 87 | 90 | 92 | 91 | 90 | 3 |
202223 | Anushka Sen | 70 | 75 | 71 | 74 | 80 | 78 | 75 | 1 |
202224 | Aakash Jain | 80 | 75 | 72 | 74 | 85 | 80 | 78 | 7 |
202225 | Akshay Agarwal | 85 | 80 | 78 | 88 | 90 | 82 | 84 | 7 |
202226 | Shwetali Bhagwat | 90 | 80 | 85 | 88 | 90 | 80 | 86 | 6 |
202227 | Mayuri Wagh | 80 | 80 | 85 | 80 | 82 | 85 | 82 | 4 |
202228 | Utkarsh Rokade | 85 | 80 | 80 | 90 | 84 | 84 | 84 | 5 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |
202230 | Mayur Jain | 80 | 88 | 87 | 90 | 92 | 90 | 88 | 1 |
Example 1: Write a query to retrieve the student’s information from the Diploma_Student table.
SELECT * FROM Diploma_Student;
The above example fetched the student's information from the Diploma_Student table.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202111 | Vaishnavi Patil | 94 | 91 | 88 | 85 | 95 | 92 | 91 | 6 |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202113 | Yash Dhull | 90 | 88 | 94 | 87 | 85 | 90 | 89 | 3 |
202114 | Sonali Patole | 95 | 90 | 92 | 88 | 92 | 90 | 91 | 4 |
202115 | Axar Patel | 85 | 80 | 82 | 86 | 92 | 84 | 85 | 1 |
202116 | Meena Mishra | 78 | 75 | 80 | 74 | 85 | 77 | 78 | 3 |
202117 | Mahesh Kumbhar | 75 | 80 | 75 | 78 | 80 | 76 | 77 | 5 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202119 | Sopan Bhore | 70 | 68 | 75 | 75 | 80 | 80 | 75 | 2 |
202220 | Prajwal Lokhande | 80 | 85 | 85 | 75 | 78 | 80 | 81 | 4 |
202221 | Anuja Wanare | 85 | 88 | 86 | 82 | 84 | 85 | 85 | 7 |
202222 | Venkatesh Iyer | 90 | 89 | 87 | 90 | 92 | 91 | 90 | 3 |
202223 | Anushka Sen | 70 | 75 | 71 | 74 | 80 | 78 | 75 | 1 |
202224 | Aakash Jain | 80 | 75 | 72 | 74 | 85 | 80 | 78 | 7 |
202225 | Akshay Agarwal | 85 | 80 | 78 | 88 | 90 | 82 | 84 | 7 |
202226 | Shwetali Bhagwat | 90 | 80 | 85 | 88 | 90 | 80 | 86 | 6 |
202227 | Mayuri Wagh | 80 | 80 | 85 | 80 | 82 | 85 | 82 | 4 |
202228 | Utkarsh Rokade | 85 | 80 | 80 | 90 | 84 | 84 | 84 | 5 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |
202230 | Mayur Jain | 80 | 88 | 87 | 90 | 92 | 90 | 88 | 1 |
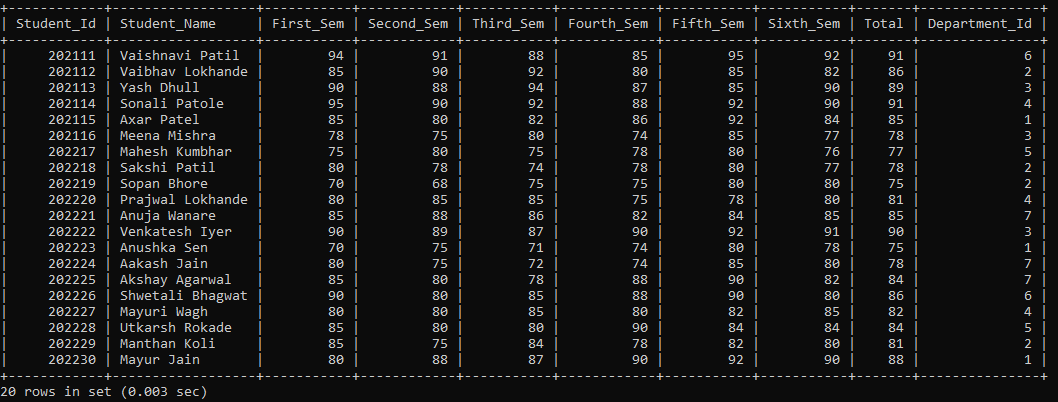
SQL SELECT Statement with the WHERE Clause
Example 2: Write a query to retrieve the information of those students from the Diploma_Student table whose student department id is 2
SELECT * FROM Diploma_Student WHERE Department_Id = 2;
In this example, we fetch the information whose department id is 2 from the table. Here, we applied the condition using the WHERE clause in the SELECT statement.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202119 | Sopan Bhore | 70 | 68 | 75 | 75 | 80 | 80 | 75 | 2 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |

SQL SELECT Statement with the ORDER BY Clause
Example 3: Write a query to retrieve the student’s information from the Diploma_Student table in the descending order by Total column.
SELECT * FROM Diploma_Student ORDER BY Total DESC;
In this query, we fetched the student’s information from the Diploma_Student table in the descending order by Total column.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202111 | Vaishnavi Patil | 94 | 91 | 88 | 85 | 95 | 92 | 91 | 6 |
202114 | Sonali Patole | 95 | 90 | 92 | 88 | 92 | 90 | 91 | 4 |
202222 | Venkatesh Iyer | 90 | 89 | 87 | 90 | 92 | 91 | 90 | 3 |
202113 | Yash Dhull | 90 | 88 | 94 | 87 | 85 | 90 | 89 | 3 |
202230 | Mayur Jain | 80 | 88 | 87 | 90 | 92 | 90 | 88 | 1 |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202226 | Shwetali Bhagwat | 90 | 80 | 85 | 88 | 90 | 80 | 86 | 6 |
202221 | Anuja Wanare | 85 | 88 | 86 | 82 | 84 | 85 | 85 | 7 |
202115 | Axar Patel | 85 | 80 | 82 | 86 | 92 | 84 | 85 | 1 |
202228 | Utkarsh Rokade | 85 | 80 | 80 | 90 | 84 | 84 | 84 | 5 |
202225 | Akshay Agarwal | 85 | 80 | 78 | 88 | 90 | 82 | 84 | 7 |
202227 | Mayuri Wagh | 80 | 80 | 85 | 80 | 82 | 85 | 82 | 4 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |
202220 | Prajwal Lokhande | 80 | 85 | 85 | 75 | 78 | 80 | 81 | 4 |
202224 | Aakash Jain | 80 | 75 | 72 | 74 | 85 | 80 | 78 | 7 |
202116 | Meena Mishra | 78 | 75 | 80 | 74 | 85 | 77 | 78 | 3 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202117 | Mahesh Kumbhar | 75 | 80 | 75 | 78 | 80 | 76 | 77 | 5 |
202119 | Sopan Bhore | 70 | 68 | 75 | 75 | 80 | 80 | 75 | 2 |
202223 | Anushka Sen | 70 | 75 | 71 | 74 | 80 | 78 | 75 | 1 |
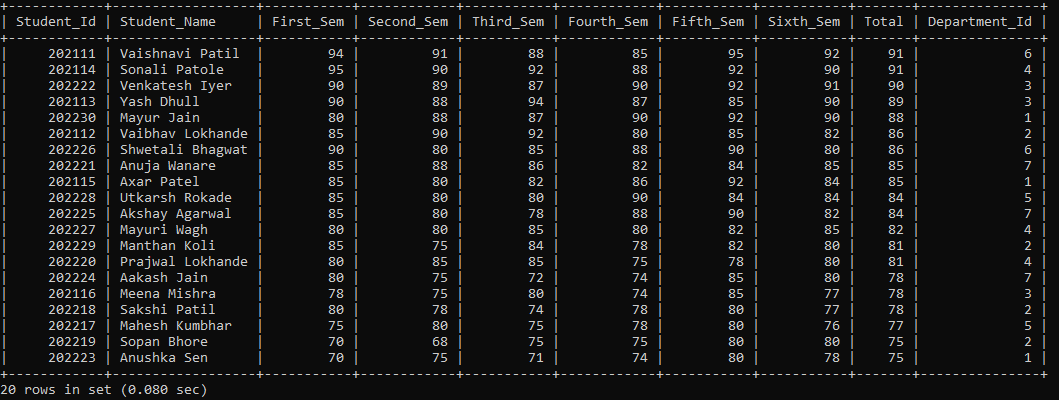
SQL SELECT Statement with the GROUP BY Clause
Example 4: Write a query to retrieve the student’s information from the Diploma_Student table GROUP BY First_Sem column.
SELECT * FROM Diploma_Student GROUP BY First_Sem;
The above example fetched the student's information from the Diploma_Student table GROUP BY First_Sem column.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202119 | Sopan Bhore | 70 | 68 | 75 | 75 | 80 | 80 | 75 | 2 |
202117 | Mahesh Kumbhar | 75 | 80 | 75 | 78 | 80 | 76 | 77 | 5 |
202116 | Meena Mishra | 78 | 75 | 80 | 74 | 85 | 77 | 78 | 3 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202113 | Yash Dhull | 90 | 88 | 94 | 87 | 85 | 90 | 89 | 3 |
202111 | Vaishnavi Patil | 94 | 91 | 88 | 85 | 95 | 92 | 91 | 6 |
202114 | Sonali Patole | 95 | 90 | 92 | 88 | 92 | 90 | 91 | 4 |

SQL SELECT Statement with the AND – OR Operator
Example 5: Write a query to retrieve the information of those students from the Diploma_Student table whose student department id is 2 and the percentage of sixth sem is greater than 75.
SELECT * FROM Diploma_Student WHERE Department_Id = 2 AND Sixth_Sem > 75;
In the above example, we fetched the information of those students from the Diploma_Student table whose student department id is 2 and the percentage of sixth sem is greater than 75.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202119 | Sopan Bhore | 70 | 68 | 75 | 75 | 80 | 80 | 75 | 2 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |

Example 6: Write a query to retrieve the information of those students from the Diploma_Student table whose First_Sem percentage is greater than 80 or Fifth_Sem percentage is greater than 80.
SELECT * FROM Diploma_Student WHERE First_Sem > 85 OR Fifth_Sem > 80;
In the above example, we fetched the information of those students from the Diploma_Student table whose First_Sem percentage is greater than 80 or Fifth_Sem percentage is greater than 80.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202111 | Vaishnavi Patil | 94 | 91 | 88 | 85 | 95 | 92 | 91 | 6 |
202112 | Vaibhav Lokhande | 85 | 90 | 92 | 80 | 85 | 82 | 86 | 2 |
202113 | Yash Dhull | 90 | 88 | 94 | 87 | 85 | 90 | 89 | 3 |
202114 | Sonali Patole | 95 | 90 | 92 | 88 | 92 | 90 | 91 | 4 |
202115 | Axar Patel | 85 | 80 | 82 | 86 | 92 | 84 | 85 | 1 |
202116 | Meena Mishra | 78 | 75 | 80 | 74 | 85 | 77 | 78 | 3 |
202221 | Anuja Wanare | 85 | 88 | 86 | 82 | 84 | 85 | 85 | 7 |
202222 | Venkatesh Iyer | 90 | 89 | 87 | 90 | 92 | 91 | 90 | 3 |
202224 | Aakash Jain | 80 | 75 | 72 | 74 | 85 | 80 | 78 | 7 |
202225 | Akshay Agarwal | 85 | 80 | 78 | 88 | 90 | 82 | 84 | 7 |
202226 | Shwetali Bhagwat | 90 | 80 | 85 | 88 | 90 | 80 | 86 | 6 |
202227 | Mayuri Wagh | 80 | 80 | 85 | 80 | 82 | 85 | 82 | 4 |
202228 | Utkarsh Rokade | 85 | 80 | 80 | 90 | 84 | 84 | 84 | 5 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |
202230 | Mayur Jain | 80 | 88 | 87 | 90 | 92 | 90 | 88 | 1 |
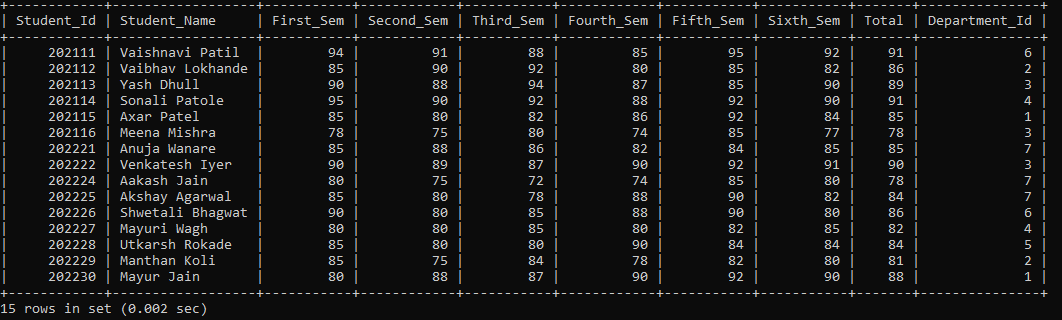
SQL SELECT Statement with the BETWEEN – LIKE Operator:
Example 7: Write a query to retrieve the student's information from the Diploma_Student table where the Second_Sem percentage is exist between 75 and 85.
SELECT * FROM Diploma_Student WHERE Second_Sem BETWEEN 75 AND 85;
In the above example, we fetched the student's information from the Diploma_Student table, where the student Second_Sem percentage is exist between 75 and 85.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202115 | Axar Patel | 85 | 80 | 82 | 86 | 92 | 84 | 85 | 1 |
202116 | Meena Mishra | 78 | 75 | 80 | 74 | 85 | 77 | 78 | 3 |
202117 | Mahesh Kumbhar | 75 | 80 | 75 | 78 | 80 | 76 | 77 | 5 |
202118 | Sakshi Patil | 80 | 78 | 74 | 78 | 80 | 77 | 78 | 2 |
202220 | Prajwal Lokhande | 80 | 85 | 85 | 75 | 78 | 80 | 81 | 4 |
202223 | Anushka Sen | 70 | 75 | 71 | 74 | 80 | 78 | 75 | 1 |
202224 | Aakash Jain | 80 | 75 | 72 | 74 | 85 | 80 | 78 | 7 |
202225 | Akshay Agarwal | 85 | 80 | 78 | 88 | 90 | 82 | 84 | 7 |
202226 | Shwetali Bhagwat | 90 | 80 | 85 | 88 | 90 | 80 | 86 | 6 |
202227 | Mayuri Wagh | 80 | 80 | 85 | 80 | 82 | 85 | 82 | 4 |
202228 | Utkarsh Rokade | 85 | 80 | 80 | 90 | 84 | 84 | 84 | 5 |
202229 | Manthan Koli | 85 | 75 | 84 | 78 | 82 | 80 | 81 | 2 |
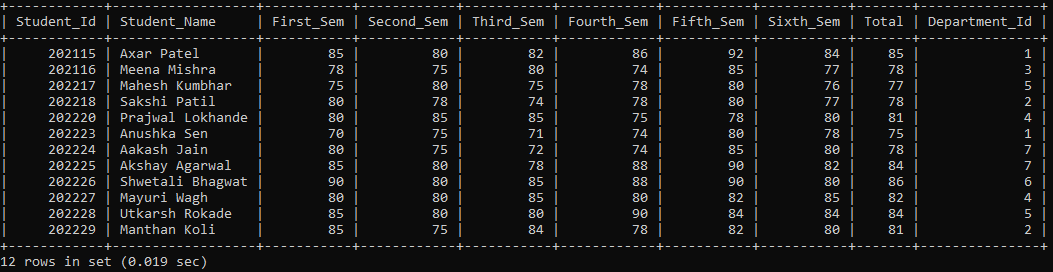
Example 8: Write a query to retrieve the student's information from the Diploma_Student table where the student's name starts with the letter 'A'.
SELECT * FROM Diploma_Student WHERE Student_Name LIKE ‘A%’;
In the above example, we fetched the student's information from the Diploma_Student table, where the student's name starts with the letter 'A'.
The output of the above query is as follows:
Student_Id | Student_Name | First_Sem | Second_Sem | Third_Sem | Fourth_Sem | Fifth_Sem | Sixth_Sem | Total | Department_Id |
202115 | Axar Patel | 85 | 80 | 82 | 86 | 92 | 84 | 85 | 1 |
202221 | Anuja Wanare | 85 | 88 | 86 | 82 | 84 | 85 | 85 | 7 |
202223 | Anushka Sen | 70 | 75 | 71 | 74 | 80 | 78 | 75 | 1 |
202224 | Aakash Jain | 80 | 75 | 72 | 74 | 85 | 80 | 78 | 7 |
202225 | Akshay Agarwal | 85 | 80 | 78 | 88 | 90 | 82 | 84 | 7 |
