Addition of two Numbers in C
In this article, we will learn about the addition of two numbers program in C language. There are many methods to get a sum of two numbers, such as using a function and adding two numbers in the main program. Howeve, the main goal of the program is to add two numbers and display the output. Lets see different ways to get the addition of two numbers in C.
Example-1: Addition of two numbers in the main function
#include <stdio.h>
int main() {
int a=20;
int b=30;
int c;
//Adding two numbers using + operand
c=a+b;
printf("Sum of the %d and %d is %d",a,b,c);
return 0;
}
Output:
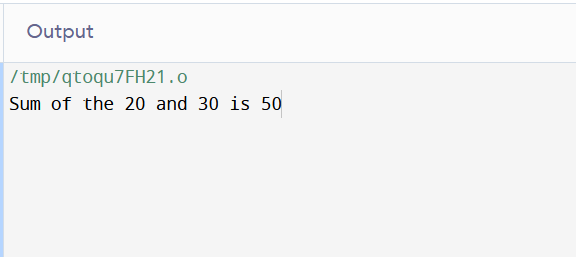
Explanation:
In the above code, we have declared three variables a,b and c. We have assigned values to the a and b variables as 20 and 30. In variable c, we stored the output of the sum of a and b. using print statement; we printed the output c.
Example-2: Addition of two numbers by taking the input from the user
#include <stdio.h>
int main() {
int a;
int b;
int c;
printf("Enter a value:");
//Using scanf statement to take the value from the user and storing it in a
scanf("%d",&a);
printf("Enter a value:");
//Using scanf statement to take the value from the user and storing it in b
scanf("%d",&b);
//Adding two numbers a and b using + operand
c=a+b;
printf("Sum of %d and %d is %d",a,b,c);
return 0;
}
Output:
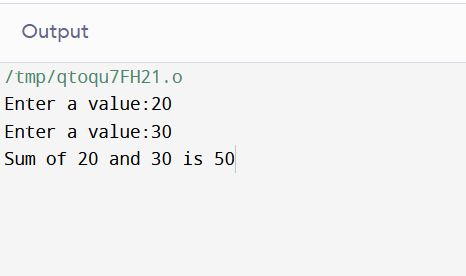
Explanation:
In the above example, we have taken the input from the user for adding two numbers. We declared three variables, a, b, and c. We have taken the input from the user to store in a and b using the scanf statement. In variable c, we have stored the output of addition of two numbers. Then using the print statement, we printed the output value c.
Example-3: Addition of two numbers using a function
#include <stdio.h>
//Created a function with a name sum that will return the value of the sum of two numbers
int sum(int a, int b){
return a+b;
}
int main()
{
int a, b,c;
printf("Enter first number: ");
//Using scanf statement to take the value from the user and storing it in a
scanf("%d", &a);
printf("Enter second number: ");
//Using scanf statement to take the value from the user and storing it in b
scanf("%d", &b);
//Calling the function sum and using the variable c, we are storing the returned value from the function
c = sum(a, b);
printf("Sum of the entered numbers: %d", c);
return 0;
}
Output:
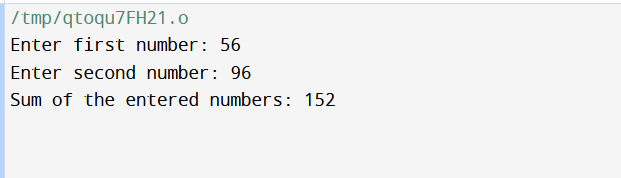
Explanation:
In the above code, we have created a function with the name sum, which returns the value by adding the two values. We have taken the input from the user using the scanf function. In the main function, we declared three variables, a b, and c. Then we called the function sum() by passing the parameters a and b. When the function is called, the sum of the passed parameters will return to the main function and be stored in variable c. Using the print statement; we have printed the output c.
Example: Addition of two numbers using function
#include <stdio.h>
//Created a function with a name sum that will return the value of the sum of two numbers
int sum(int a, int b){
int c;
c=a+b;
printf("Sum of the two number %d and %d is %d",a,b,c);
}
int main()
{
int a, b,c;
printf("Enter first number: ");
scanf("%d", &a);
printf("Enter second number: ");
scanf("%d", &b);
//Calling the function sum
sum(a, b);
return 0;
}
Output:
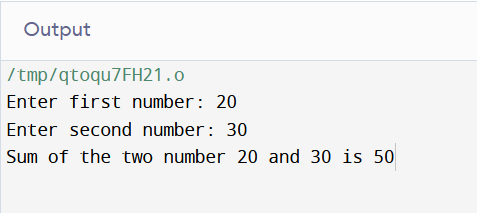
Explanation:
In the above code, we created a function with the name sum, which prints the sum of two numbers. In the main function, we declared three variables, a, b, and c. We have taken the input from the user using the scanf function. Then we called the function sum() by passing the parameters a and b. Unlike in the previous example, where the function returned value to the main, In this example, we created the sum function such that the sum of two numbers will be printed as soon as the function is called. When the function call takes place by passing parameters a and b in the function, a variable c is created, which stores the value of sum of two numbers. Using the print statement, we printed the output c in the sum function itself without returning any value.