Prototype in C
A prototype is nothing but a model, a model of initial creation of an intended product. Similarly, in the C programming language, all functions have a prototype. In general, all functions in C must have a prototype but there exist programs in which the functions exist or can exist without having a prototype.
Function prototype in C
A prototype is nothing but a model, a model of initial creation of an intended product. Similarly, In C programming, a function prototype can be defined as the declaration in the code that tells the compiler about the following information of a function;
- Data type of the function
- return type
- Parameter list
In simple terms, a function prototype is a function which tells or informs the compiler about the return type, name of the function and its arguments or parameters. This is done in order to match with the given function calls when it is necessary or when it is required.
Syntax
return_type function_name ( dataType arg1, dataType arg2, …);
Prototype declaration in C consists of three parts:
- Function Name
- return type
- parameter list
Example of prototype declaration in C
- int add( int m, int n);
In the above example, the name of the function is add and the return type of this ‘add’ function is the integer datatype.
‘m’ and ‘n’ are the arguments which are passed to the function. The arguments ‘m’ and ‘n’ are both integer types.
Note: In the above program, we can declare ‘n’ number of prototypes but they all must be different in terms of function name or arguments or parameter list. The only thing left is to define a prototype in the code. After this, we can call it anytime by simply using the name of the function.
Use of prototype in C
- It helps the compiler to check the correctness of a function.
- We can declare ‘n’ number of prototypes but they all must be different in terms of function name or arguments or parameter list. As discussed above, the function prototypes contains;
- The name of the function
- return type
- Argument list
- Suppose, a function call is generated somewhere, but the body of the function is not defined yet. Its body is defined later (after the current line), then it may generate issues.
What happens is, the compiler will not be able to find what is the function and what is its signature. In such cases, we need prototypes.
Scope of prototype in C
The scope of the function prototype in C is determined by its position in the program. In C programming, the function prototype is located after the header files in the program.
Note: The scope of the function prototype is weighed (prototype is considered) within the same block as the function call.
Program 1: Calculating sum of two numbers
#include <stdio.h>
int add( int x , int y ); // prototype for the function
int main() // main function
{
int num1,num2,result; // declaring the local variables
printf( " Please enter 2 numbers to find their sum : " ) ;
scanf( "%d %d" , &num1 , &num2 ) ;
result = add( num1 , num2 ) ; // function call
printf( " The sum of the given numbers is = %d " , result ) ;
return 0 ;
}
int add( int x , int y) // function definition for prototype
{
int sum;
sum = x + y ; //sum stores the result of the calculation made
return sum ;
}
Output of the program:

Code explanation:
From the above code, we can observe that initially we are declaring the function prototype for calculating the sum of two numbers with function name as “add” which is of integer return type. The arguments passed are named as x and y (both integers) into the function.
There are three local variables of type int that are passed in the main class, the defined three integers are num1, num2, and result.
After the declarations are done, we are taking input from the users using the scanf() function and then storing the results of the add function of the two given numbers in the result variable.
Function call is generated by calling “add“, here “add” function is used again. In the end in the function definition, you can observe that we are passing the logic. The logic is simple and performs addition of two given numbers and stores it in the variable created by the name ‘sum’.
Program 2: Calculating product of two numbers
#include <stdio.h>
int mul( int x , int y ); // prototype for the function
int main() // main function
{
int num1,num2,result;
printf( " Please enter 2 numbers to find their product : " ) ;
scanf( "%d %d" , &num1 , &num2 ) ;
result = mul( num1 , num2 ) ; // function call
printf( " The product of the given numbers is = %d ", result ) ;
return 0 ;
}
int mul( int x , int y) // function definition for prototype
{
int product;
product = x * y ; //product stores the result of the calculation made
return product;
}
Output of the program:
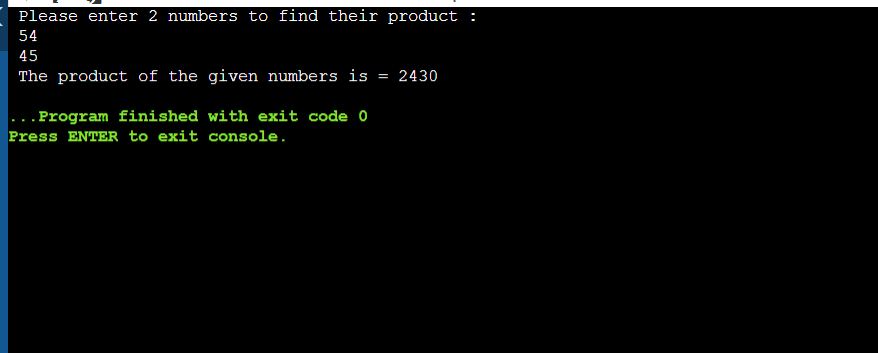