OpenGL in C
OpenGL stands for Open Graphics Library. It is a low-level, widely supported modeling and rendering software package that is widely available across all the platforms. It is an API used for rendering 2D and 3D vector graphics cross-platform and cross-language. It is mainly used for graphics applications, such as games, CAD design, or modeling. It uses polygons to represent images. It provides a graphics rendering option for many 3D games, such as Quake 3. The OpenGL API is designed mostly in hardware.
- Design: This API is described as a collection of functions that the client program can call. Although the functions are comparable to those of the C language, it is not language specific.
- Development: This API is always changing, and Chronos Group often releases updated versions with more features than before. Vendors of GPUs might also offer some extra functionality in the form of an extension.
- Associated Libraries: The OpenGL utility library, a companion library, is included in the first edition. However, since OpenGL is a rather complicated procedure. Therefore, other libraries are introduced to simplify things, such as the OpenGL Utility Toolkit, which is eventually replaced by free glut. GLEE, GLEW, and gliding were later added to the library.
- Implementation: OpenGL is implemented using Mesa 3D, an open-source program. By utilizing Direct Rendering Infrastructure, it can do pure software rendering and hardware acceleration on BSD, Linux, and other platforms.
How to install OpenGL on Ubuntu?
Enter the following command in the terminal to install OpenGL on Ubuntu (It is same as you do for any other installation):
Sudo apt-get install freeglut3-dev
To work on the Ubuntu operating system
gccfilename.c -lGL -lGLU -glut
Here, filename.c is the name of the file with which this program is saved.
Installing OpenGL in Code::Blocks on Windows
- First of all download code block and install it on the system.
- Navigate to the URL and download the zip file from the link that follows the freeglut MinGW package with the link named Download and extract freeglut 3.0.0 for MinGW.
- Run notepad as administrator,
- After that, select the File to open from this computer's C: (C-drive) > Program Files(x86) > CodeBlocks > share > CodeBlocks > templates (then click to show All Files).
- Next, search all the glut32 in glut.cbp and replace it with freeglut.
- After that, launch CodeBlocks from this computer's C: Program Filesx86CodeBlocksshareTemplatesWizardGlut (then click to show All Files).
- Open wizard.script and substitute freeglut for all glut32 there as well.
- Afterward, navigate to the freeglut folder (where it was downloaded), and
- Copy all four files from Include > GL.
- To paste it, navigate to This PC > C:(C-drive) > Program Files(x86) > CodeBlocks > MinGW > include > GL.
- Then, copy two files from the Download's freeglut > lib folder, then paste them into This PC > C:(C-drive) > Program Files(x86) > CodeBlocks > MinGW > lib.
- Once more, open the downloaded folder freeglut > bin, copy the freeglut.dll File there, and then navigate to This PC > C: (C-drive) > Windows > SysWOW64 to paste it.
- Open Code::Blocks now.
- Choose File> New > Project > GLUT project from the menu. Next,
- Enter anything for the project title, then select Next.
- For choosing the location of GLUT: This computer is located at C: Program Filesx86CodeBlocksMinGW.
- Click OK, Next, and Finish.
Code::Blocks is now prepared for testing with OpenGL files.
Working of OpenGL in C
Let’s see a simple program for circular drawing implemented in C, utilizing the OpenGL platform to demonstrate how OpenGL functions.
// C application to illustrate utilizing OpenGL to draw a circle
#include<stdio.h>
#include<GL/glut.h>
#include<math.h>
#define pi 3.142857
// work to initialize
voidmyInit (void)
{
// making the background black as the first argument and the other three being zero
glClearColor(0.0, 0.0, 0.0, 1.0);
// making the color of the image green (in RGB mode), with 1.0 as the central argument.
glColor3f(0.0, 1.0, 0.0);
// The picture's perimeter is 1 pixel wide
glPointSize(1.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
// setting the X and Y dimensions of a window
gluOrtho2D(-780, 780, -420, 420);
}
void display (void)
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_POINTS);
float x, y, i;
// up to 2*pi, or 360 degrees, iterate the y axis
// with a modest angle increment since glVertex2i only draws a point at the supplied coordinate
for( i = 0; i< (2 * pi); i += 0.001)
{
x = 200 * cos(i);
y = 200 * sin(i);
glVertex2i(x, y);
}
glEnd();
glFlush();
}
int main (intargc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
// window size in the X and Y directions
glutInitWindowSize(1366, 768);
glutInitWindowPosition(0, 0);
// naming the window
glutCreateWindow("Circle Drawing");
my init();
glutDisplayFunc(display);
glutMainLoop();
}
Output:
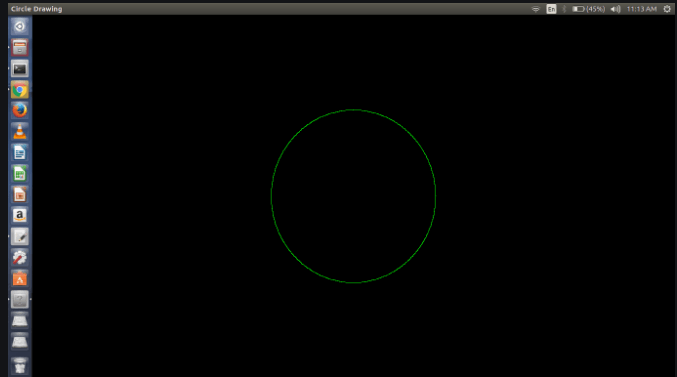