Static function in C
The functions in the C programming language are by default global. This means the programmer can easily access the function which is outside from the file where it was initially declared.
This can cause various issues and there must be a way to restrict this access. If the programmer wants to restrict this access, then there is a simple solution to that making the declared function as static.
A function can be made static by simply putting a keyword static in front of the intended function.
Function in C
A function is a self-contained block of statements that performs a specific task. A function wraps a number of statements which are repeated in a program into a single unit or block. This wrapping of statements(unit) is identified by a name known as a function name.
Note 1: Every C program no matter how easy and low in the number of lines of code will always consist of at least one function, which is the main() function.
Note 2: In C programming language, functions are global by default.
Declaration :
returnType functionName( parameters)
{
function body
}
Example:
#include<stdio.h>
int multiplication(); // function declaration
int main()
{
int output; //local variable definition
output = multiplication();
printf("The product of the two numbers is: %d\n",output);
return 0;
}
int multiplication()
{
int num1 = 5;
int num2 = 15;
return num1 * num2;
}
Static Function in C
The functions in the C programming language are by default global. This means the programmer can easily access the function which is outside from the file where it was initially declared.
A function can be made static by adding ‘static’ keyword before it.
Example 1: non static function
int sum(int a, int b)
{
printf(“This a non-static function, it is global by default”);
}
Example 2: static function
static int fun1(void)
{
printf(“This is a static function”);
}
In Example 1, the function sum() is not static. This means that if we want to access sum() outside of the file where sum() was declared, we can access it with ease.
But, In Example 2, the function fun1() is declared static. Unlike sum() which is a global function, access to fun1() is restricted to the file where fun1() is declared.
The other reason to make a function static is the reusability feature. Making functions static allows reuse of the same function name in other files. Also, By declaring a function as static would also provide the security.
Let’s understand the importance of static function with the help of an example.
Program 1
Suppose, inside your C project you have two files, file_1.c and main.c.
The contents of both files are as follows:
1) file_1.c
static int sum(int a, int b)
{
int result;
result = a+b;
return result;
}
2) main.c
int sum(int, int);
int main()
{
int addition = sum(5, 7);
printf(“%d”, addition);
return 0;
}
Code Explanation:
If the above code is compiled, then an error will be reflected on our console. This error occurs due to the declaration of variable sum as static. By declaring sum() as static, the function is only visible in file_1.c and hence its access is limited within this file.
Program 2: A program in C demonstrating static functions
#include<stdio.h>
static int mul(int a, int b) // declaring the function as static
{
int result; // declaring the local variables
result = a*b;
printf("We are inside the static function - mul() \n ");
return result;
}
int mul(int, int);
int main()
{
int product = mul(3, 8);
printf("The output of the intended calculation is %d", product);
return 0;
}
Output of the program:
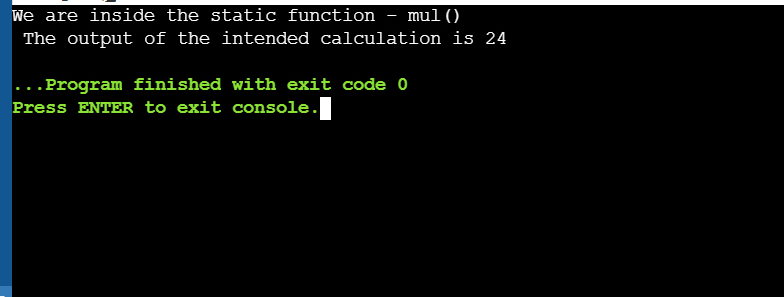
Code explanation:
In the above program, the function mul() is declared as a static function that performs multiplication operation on two numbers and prints a statement “We are inside the static function - mul() “ .
When a function call is performed by the main() function, the main() function calls the mul() function and we get the desired output.
The program works correctly as the static function mul() is called from its own file. i.e., both mul() and main() are declared inside one file and hence their access lies within this file. That’s why we are able to fetch the desired results from above code even though the mul() function is declared as static.