Passing Array to Function in C
Need to pass Arrays?
The need to pass arrays to a function arises when we need to pass a list of values to a given function.
During the course of our programming journey in C, there are various instances which may require passing more than one variable (variables of the same type) to a function. In such cases, we can declare and initialize an array and pass that array into the function rather than declaring multiple variables and passing them one by one as parameters of the function.
The arrays can be passed to function in one of the following ways:
1) By declaring array as a parameter in the function
Eg: int sum (int arr[]);
2) By declaring a pointer in the function to hold the base address of the array
Eg: int sum (int* ptr);
Before jumping into the depths of this topic, let’s discuss the key aspects in order to clearly understand the given topic.
Array
An array can be defined as a collection of items or logically related variables of identical data type which are stored in contiguous memory locations.
The drawback with using an array is that, once the size of array is declared it cannot be changed and hence it may result in the size of array declared which may not be sufficient for the task it was declared for.
Declaration :
data_type name_of_array[sizeOfArray];
Example:
int arr[15];
Function
A function is a self-contained block of statements that performs a specific task. A function wraps a number of statements which are repeated in a program into a single unit or block. This wrapping of statements(unit) is identified by a name known as a function name.
Note: Every C program no matter how easy and low in the number of lines of code will always consist of at least one function, which is the main() function.
Declaration :
returnType functionName( parameters)
{
function body
}
Example:
#include<stdio.h>
int multiplication(); // function declaration
int main()
{
int output; //local variable definition
output = multiplication();
printf("The product of the two numbers is: %d\n",output);
return 0;
}
int multiplication()
{
int num1 = 5;
int num2 = 15;
return num1 * num2;
}
Passing Array to function in C
Method 1: By declaring array as a parameter in the function
We can pass the one dimensional and multidimensional array in function as an argument in C.
We pass the array to a function in order to pass a list of values to the function and to make it accessible within the function.
Here, one must follow that when an entire array is passed to a function, then the corresponding function can access all the elements which are declared in the array.
Let’s understand this method using a program
Program 1: Passing Array to function in C using Method 1
#include <stdio.h>
int sum(int arr[])
{
int array_sum =0;
for (int i = 0; i<5; ++i)
{
array_sum += arr[i];
}
return array_sum;
}
int main()
{
int result, array[] = {3,6,9,12,15};
result = sum(array); // function call
printf("The Sum of the passed values is = %d", result);
return 0;
}
Output of the program:
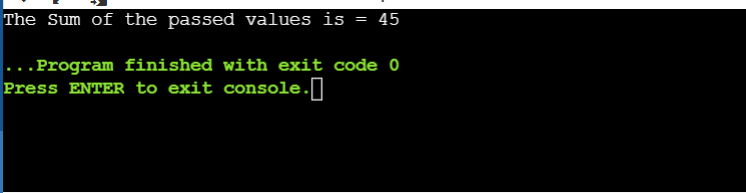
Method 2: By declaring a pointer in the function to hold the base address of the array
When we pass the address of an array while making a function call, then this method of calling a function is referred to as call by reference.
Here, one must follow that when an address is passed as an argument in the function, the pointer in the function will receive the address(location) of the corresponding array.
Declaration : int fun (int *ptr) { ... }
Note:
We must follow that, when any change is made in the array using pointers in the functions, the corresponding array will also be affected by this.
For Example, the array element arr[1] = 33, and in function we code *(ptr+1) = 65,
then the element which is stored at arr[1] becomes equal to 65.
Program 2: Passing Array to function in C using Method 2
#include <stdio.h>
int func_one(int array[])
{
for (int i = 0; i < 5; i++)
{
printf("The value at %d is %d\n", i, array[i]);
}
/* array[0] = 382; if you change any value here, it gets reflected in main() */
return 0;
}
void func_two(int *ptr)
{
for (int i = 0; i < 5; i++)
{
printf("The value at %d is %d\n", i, *(ptr + i));
}
*(ptr + 2) = 1234;
}
void func_three(int arr[2][2])
{
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 2; j++)
{
printf("The value at %d, %d is %d\n", i, j, arr[i][j]);
}
}
}
int main()
{
int arr[][2] = {{3, 69}, {6, 9}};
func_three(arr); // function call
return 0;
}
Output of the program:
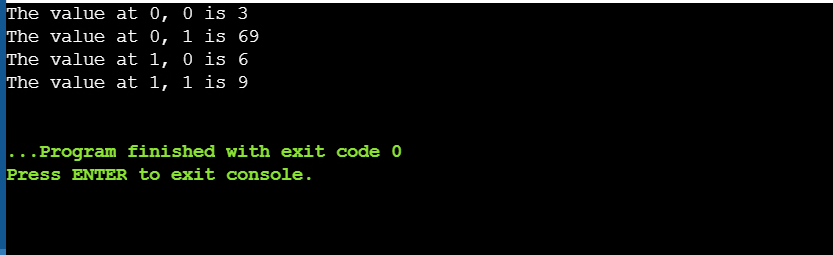
Conclusion:
In C programming, there are various problems which may require us to pass multiple variables of the same type into a function and declaring each variable separately and then passing it into the function will not only take more time but will also reduce the efficiency of the program.
The solution to such instances is to pass arrays to a function when we need to pass a list of values to a given function.
The arrays can be passed to function in one of the following ways:
- By declaring array as a parameter in the function
- By declaring a pointer in the function to hold the base address of the array.