Calloc in C
The calloc() is a library function in C which is used for memory allocation. The calloc() function dynamically allocates multiple blocks of memory to complex data structures.
This includes data structures such as arrays.
An array is a collection of items or logically related variables of identical data type which are stored in contiguous memory locations. Once the size of array is declared, it cannot be changed and hence the size of array declared may not be sufficient enough for the task it was declared for. This may lead to unwanted termination of the program or not getting the desired outputs.
This problem can be resolved by allocating the memory during run time, this process has to be manual. This manual declaration of memory at run time is known as dynamic memory allocation and this is carried by a special set of functions known as library functions. The calloc() is a library function in C which is used for dynamic memory allocation.
The malloc() function also performs the task of memory allocation but the allocated memory is uninitialized.
Meaning
The term calloc is short for contiguous allocation and is a library function in C which allocates the memory which is requested and returns a pointer to it. The pointer is returned to the beginning of the block of memory.
Note: The block of memory allocated is set to zero, i.e., all bits are set to zero.
Declaration
void *calloc (n, size)
where, n = number of elements to be allocated
size = Size of the elements
Eg: p = (int*) calloc(10, sizeof(int)); /*This statement allocates contiguous space in
memory for 10 elements of type int */
Program: C program for showing the usage of calloc() function
#include <stdio.h>
#include <stdlib.h>
int main ()
{
int i, n; //declaring the local variables
int *p;
printf("Enter number of elements : "); //number of elements required
scanf("%d",&n); //scanning the input
p = (int*)calloc(n, sizeof(int)); //declaring the calloc function
printf("Enter the numbers here (%d numbers) :\n",n); //numbers entered by user
for( i=0 ; i < n ; i++ ) {
scanf("%d",&p[i]);
}
printf("The numbers entered are as follows : ");
for( i=0 ; i < n ; i++ ) {
printf("%d ",p[i]);
}
free( p );
return(0);
}
Output of the following program:
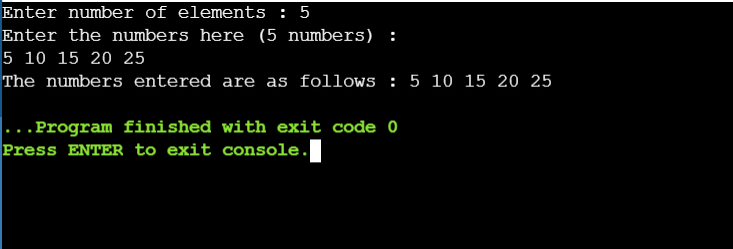
Conclusion
The calloc() function is a library function in C and it is used for allocation of space for complex data types such as an array. It allocates space for an array of elements, initializes them to zero and then returns a pointer to the memory.
Note 1: Difference between malloc and calloc
malloc() function allocates memory but does not set the memory to zero, i.e.,
memory is uninitialized.
calloc() function on the other hand allocates memory and set the memory to
zero, all bits are initialized to zero.
Note 2: The calloc() function returns a pointer to the allocated memory, but if there is an error while allocating memory space like shortage of memory, then the return value is NULL.