Palindrome Number in C
What is a Palindrome?
A number that doesn't change when reversed is known as a palindrome.
We reverse a number and compare it to the original number to determine whether it is a palindrome or not. It is a palindrome if both are the same; otherwise, it is not.
For example: abcba, 121, MALYALAM, 45454 etc.
These examples show that the number will remain the same whether we read them forward or backward. Therefore, this kind of number is a palindrome.
Logical Approach:
- Get input from the user through the keyboard.
- Store this input in any random variable.
- Find the reverse of the input which is entered by the user.
- Compare the reverse of input with the random variable.
- If the reverse and random variables match, the input will be a palindrome.
- If the reverse and random variables do not match, then the input number will not be a palindrome.
Program to check whether the number is palindrome or not.
Using For loop:
#include<stdio.h>
int main()
{
int number,i,remainder,random_variable,reversible_no=0;
printf("\n Enter The Number:");
scanf("%d",&number);
random_variable = number;
//For Loop to find the reversible number
for(i=number;i>0; )
{
remainder=i%10;
reversible_no=reversible_no*10+remainder;
i=i/10;
}
// if else conditional statement to check whether the reversible number and input number are the same or not
if(reversible_no==number)
{
printf("\n %d is a Palindrome Number", random_variable);
}
else
{
printf("\n %d is not a Palindrome Number", random_variable);
}
return 0;
}
Explanation:
- Here we have declared five variables “number”, “i”, “remainder”, “random_variable”, and “reversible_no”.
- We stored the “number” variable in the “random_variable”.
- We have applied for loop to find the reverse of input.
- In for loop “i” variable is assigned to the “number” variable.
- Given the condition (i>0) in a for loop, the body of the loop will be executed up to the point where “i” is greater than 0. Following that, the loop will be broken, and control will shift outside of it.
- In for loop curly braces, we have three action statements. The first statement gives us the “remainder” variable value. The second statement gives us the “reversible_no” variable value. And the last statement gives us a new ”i” value.
- These Three statements will be executed until the condition is false.
- Then we use the if-else conditional statement to check whether the entered number and the reversible number are the same or not.
- If they both are the same, then the input number is a palindrome number.
- If they are not identical, then the input number is not a palindrome number.
Program Output:
When the condition is satisfied
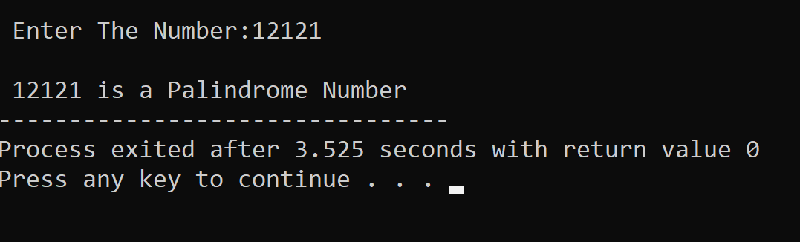
When the condition is not satisfied
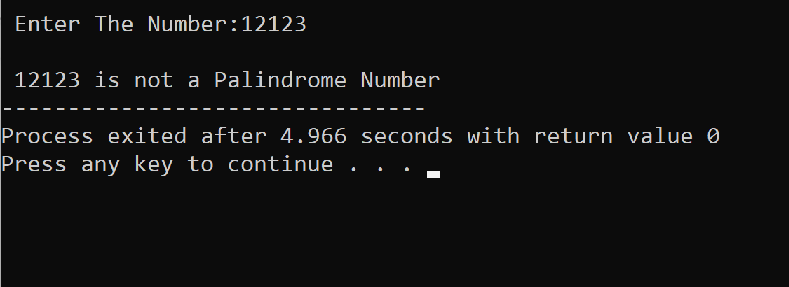
C program to determine whether a string is a palindrome or not.
- Palindrome of string denotes that if we read a sting from right to left or left to right, we get similar output.
- So, if we want to check whether a given or input string is palindrome or not, we do a comparison between characters of a string.
- If the string has 5 characters, so in that case, we will compare the first and last character of a string and then the second and second last character of a string; if they are the same, then this string will be a palindrome string.
Program:
#include <stdio.h>
#include <string.h>
int main()
{
char str[20];
int i, string_length, string_flag = 0;
//Give input from the user through the keyboard
printf("For a palindrome check, enter a string: ");
scanf("%s", str);
// count the length of the string
string_length = strlen(str);
for(i = 0; i < string_length; i++)
{
if(str[i] != str[string_length-i-1])
{
string_flag = 1;
break;
}
}
if(string_flag==0)
{
printf("The provided string is a palindrome.");
}
else {
printf("The provided string is not a palindrome.");
}
return 0;
}
Explanation:
- In above code, three variables with the int data type are present here: “i”, "string length," and "string flag." Additionally, we have a variable called "str" with the data type char.
- To check a string for palindrome, we used a for loop to compare the first character with the final character and the second character with the second last character. We repeated this process until the middle character of the string was reached.
- If any comparison results in a mismatch, end the for loop and set the " string_flag" value to 1.
- If we determine that "string _flag" is equal to 0, using the if-else conditional expression, the supplied string will be a palindrome string.
- However, the supplied string won't be a palindrome if "string_flag" is not equal to 0.
Program Output:
When the condition is satisfied
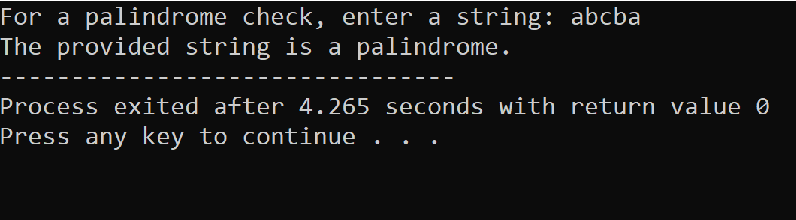
When the condition is not satisfied
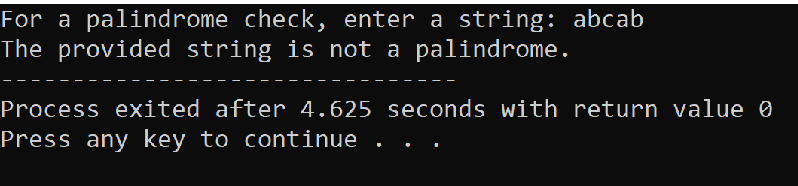