Bank management system in C
This is a mini project that is constructed completely using the C language. The bank management system is a beginner friendly project. To construct this user only needs to know about basic data structures and syntax of the c language. No advanced topic needs to be known. Here our bank management system will consist of all the processes that used to be done in ordinary banks, such as creating a new account, registering for net banking, depositing money, withdrawing, etc. Firstly we will look into the code part by part. Each part will consist of a feature. Understanding each feature individually will make things easier as the code will be vast and bulky, so let's get started. Let us construct the blueprint of our bank management system.
Features of the bank management system
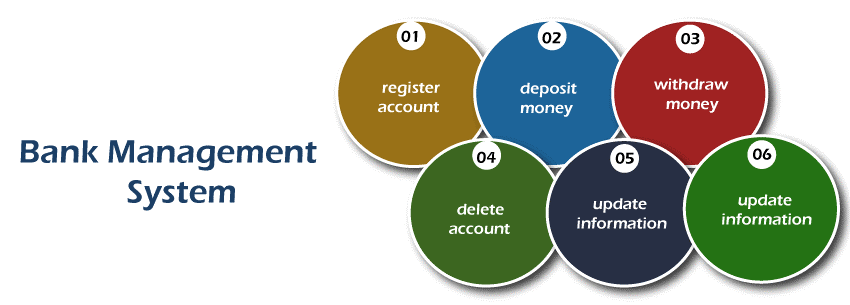
- Register account - through this, the user will be able to register them, and their data will be kept until the account is deleted.
- Deposit money - through this, the user can deposit money to their existing account.
- Withdraw money - through this feature, the user can withdraw money from their account. It would consist of one more feature: we always need to check whether the user has sufficient balance to withdraw. If yes, only the money will be withdrawn, and the amount will decrease from the balance.
- Check balance - through this feature, the user will be able to check the available balance of their account.
- Update information - through this feature, users can update their existing features, such as phone number, date of birth, email ID, etc.
- Delete the account - through this feature, we can delete any particular user or the registered account holder.
So now we have made the blueprint and let's code our mini project.
Bank. c
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
#include<math.h>
struct date{
int month,day,year;
};
struct user {
char name[50];
int phone_no;
int acc_no;
char password[50];
float avl_bal;
struct date dob;
struct date deposit;
int age;
char address[50];
char citizenship[50];
int amt;
}add,upd,check,rem,transaction;
void close()
{
printf("closing the current opeartion ! :) thank you ");
}
void new_acc()
{
int choice;
printf("\t\t\t ADD RECORD ");
printf("\n\n\nEnter today's date(mm/dd/yyyy):");
scanf("%d/%d/%d",&add.deposit.month,&add.deposit.day,&add.deposit.year);
printf("\nEnter the account number:");
scanf("%d",&check.acc_no);
while(scanf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",&add.acc_no,add.name,&add.dob.month,&add.dob.day,&add.dob.year,&add.age,add.address,add.citizenship,&add.phone_no,&add.amt,&add.deposit.month,&add.deposit.day,&add.deposit.year)!=EOF)
{
if (check.acc_no==add.acc_no)
{printf("Account no. already in use!");
printf("\n exiting -------");
}
}
add.acc_no=check.acc_no;
printf("\nEnter the name:");
scanf("%s",add.name);
printf("\nEnter the date of birth(mm/dd/yyyy):");
scanf("%d/%d/%d",&add.dob.month,&add.dob.day,&add.dob.year);
printf("\nEnter the age:");
scanf("%d",&add.age);
printf("\nEnter the address:");
scanf("%s",add.address);
printf("\nEnter the citizenship number:");
scanf("%s",add.citizenship);
printf("\nEnter the phone number: ");
scanf("%d",&add.phone_no);
printf("\nEnter the amount to deposit:$");
scanf("%d",&add.amt);
printf("\nType of account:\n\t#Saving\n\t#Current\n\t#Fixed1(for 1 year)\n\t#Fixed2(for 2 years)\n\t#Fixed3(for 3 years)\n\n\tEnter your choice:");
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
printf("\nAccount created successfully!");
}
void edit()
{
printf("\nEnter the account no. of the customer whose info you want to change:");
scanf("%d",&upd.acc_no);
int test =0,choice;
while(scanf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",&add.acc_no,add.name,&add.dob.month,&add.dob.day,&add.dob.year,&add.age,add.address,add.citizenship,&add.phone_no,&add.amt,&add.deposit.month,&add.deposit.day,&add.deposit.year)!=EOF)
{
if (add.acc_no==upd.acc_no)
{ test=1;
printf("\nplease let us know Which information do you want to change?\n1.Address\n2.Phone\n\npleaseEnter your choice(1 for address and 2 for phone):");
scanf("%d",&choice);
if(choice==1)
{printf("please Enter the new address:");
scanf("%s",upd.address);
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
printf("Changes are saved now! :) happy banking");
}
else if(choice==2)
{
printf("Enter the new phone number:");
scanf("%d",&upd.phone_no);
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
printf("Changes are saved now! :) happy banking");
}
}
else
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
}
if(test!=1)
{
int k;
printf("\nOops :( Record not found!!\t\t\t");
printf("\nEnter 0 if you want to try again,or 1 if you want to return to main menu and 2 if you want to exit:");
scanf("%d",&k);
if (k==1)
menu();
else if (k==2)
close();
else if(k==0)
edit();
else
{printf("\nInvalid!\a");
}
}
else
{
int main_exit;
printf("\n\n\nEnter 1 to go to the main menu and 0 to exit:");
scanf("%d",&main_exit);
system("cls");
if (main_exit==1)
menu();
else
close();
}
}
void erase()
{
printf("\nEnter the account no. of the customer whose info you want to change:");
scanf("%d",&upd.acc_no);
int test =0,choice;
while(scanf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",&add.acc_no,add.name,&add.dob.month,&add.dob.day,&add.dob.year,&add.age,add.address,add.citizenship,&add.phone_no,&add.amt,&add.deposit.month,&add.deposit.day,&add.deposit.year)!=EOF)
{
if (add.acc_no==upd.acc_no)
{
printf("\nRecord deleted successfully!\n");
}
else
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
}
if(test!=1)
{
int k;
printf("\nOops :( Record not found!!\t\t\t");
printf("\nEnter 0 if you want to try again,or 1 if you want to return to main menu and 2 if you want to exit:");
scanf("%d",&k);
if (k==1)
menu();
else if (k==2)
close();
else if(k==0)
edit();
else
{printf("\nInvalid!\a");
}
}
else
{
int main_exit;
printf("\n\n\nEnter 1 to go to the main menu and 0 to exit:");
scanf("%d",&main_exit);
system("cls");
if (main_exit==1)
menu();
else
close();
}
}
void transact()
{
int choice,test=0;
printf("Enter the valid account number of the customer please,note- YOU CANNOT DEPOSIT OR WITHDRAW CASH IN FIXED ACCOUNTS!, so proceed further only if the account is not fixed account :");
scanf("%d",&transaction.acc_no);
while(scanf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",&add.acc_no,add.name,&add.dob.month,&add.dob.day,&add.dob.year,&add.age,add.address,add.citizenship,&add.phone_no,&add.amt,&add.deposit.month,&add.deposit.day,&add.deposit.year)!=EOF)
{
if(add.acc_no==transaction.acc_no)
{ test=1;
printf("\n\nDo you want to\n1.Deposit\n2.Withdraw?\n\nEnter your choice(1 for deposit and 2 for withdraw):");
scanf("%d",&choice);
if (choice==1)
{
printf("Enter the amount you want to deposit:$ ");
scanf("%d",&transaction.amt);
add.amt+=transaction.amt;
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
printf("\n\nthe entered amount is Deposited successfully! happy BANKING");
}
else
{
printf("Enter the amount you want to withdraw:$ ");
scanf("%d",&transaction.amt);
add.amt-=transaction.amt;
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
printf("\n\nthe entered amount isWithdrawn successfully!, happy banking ");
}
}
else
{
printf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
}
}
if(test!=1)
{
int k;
printf("\nOops :( Record not found!!\t\t\t");
printf("\nEnter 0 if you want to try again,or 1 if you want to return to main menu and 2 if you want to exit:");
scanf("%d",&k);
if (k==1)
menu();
else if (k==2)
close();
else if(k==0)
edit();
else
{printf("\nInvalid!\a");
}
}
else
{
int main_exit;
printf("\n\n\nEnter 1 to go to the main menu and 0 to exit:");
scanf("%d",&main_exit);
system("cls");
if (main_exit==1)
menu();
else
close();
}
}
void view_list()
{
printf("this will help in viewing the list ");
}
void see()
{
int test=0,rate;
int choice;
float time;
float interest;
char acc_type[10]="fixed1";
printf("Do you want to check by\n1.Account no\n2.Name\nEnter your choice:");
scanf("%d",&choice);
if (choice==1)
{ printf("Enter the account number:");
scanf("%d",&check.acc_no);
while (scanf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",&add.acc_no,add.name,&add.dob.month,&add.dob.day,&add.dob.year,&add.age,add.address,add.citizenship,&add.phone_no,&add.amt,&add.deposit.month,&add.deposit.day,&add.deposit.year)!=EOF)
{
if(add.acc_no==check.acc_no)
{ system("cls");
test=1;
if(strcmp(acc_type,"fixed1")==0)
{
time=1.0;
rate=9;
intrst=(time*add.amt*rate)/100;
printf("\n\nYou will get %d as interest on %d/%d/%d",intrst,add.deposit.month,add.deposit.day,add.deposit.year+1);
}
else if(strcmp(acc_type,"fixed2")==0)
{
time=2.0;
rate=11;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get %d as interest on %d/%d/%d",intrst,add.deposit.month,add.deposit.day,add.deposit.year+2);
}
else if(strcmp(acc_type,"fixed3")==0)
{
time=3.0;
rate=13;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get %d as interest on %d/%d/%d",intrst,add.deposit.month,add.deposit.day,add.deposit.year+3);
}
else if(strcmp(acc_type,"saving")==0)
{
time=(1.0/12.0);
rate=8;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get %d as interest on %d of every month",intrst,add.deposit.day);
}
else if(strcmp(acc_type,"current")==0)
{
printf("\n\nYou will get no interest\a\a");
}
}
}
}
else if (choice==2)
{ printf("Enter the name:");
scanf("%s",&check.name);
while (scanf("%d %s %d/%d/%d %d %s %s %d %d %d %d/%d\n",&add.acc_no,add.name,&add.dob.month,&add.dob.day,&add.dob.year,&add.age,add.address,add.citizenship,&add.phone_no,&add.amt,&add.deposit.month,&add.deposit.day,&add.deposit.year)!=EOF)
{
if(strcmpi(add.name,check.name)==0)
{ system("cls");
test=1;
printf("\nAccount No.:%d\nName:%s \nDOB:%d/%d/%d \nAge:%d \nAddress:%s \nCitizenship No:%s \nPhone number:%.0lf \nType Of Account:%s \nAmount deposited:$%.2f \nDate Of Deposit:%d/%d/%d\n\n",add.acc_no,add.name,add.dob.month,add.dob.day,add.dob.year,add.age,add.address,add.citizenship,add.phone_no,
acc_type,add.amt,add.deposit.month,add.deposit.day,add.deposit.year);
if(strcmpi(acc_type,"fixed1")==0)
{
time=1.0;
rate=9;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get $.%.2f as interest on %d/%d/%d",intrst,add.deposit.month,add.deposit.day,add.deposit.year+1);
}
else if(strcmp(acc_type,"fixed2")==0)
{
time=2.0;
rate=11;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get $.%.2f as interest on %d/%d/%d",intrst,add.deposit.month,add.deposit.day,add.deposit.year+2);
}
else if(strcmp(acc_type,"fixed3")==0)
{
time=3.0;
rate=13;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get $.%.2f as interest on %d/%d/%d",intrst,add.deposit.month,add.deposit.day,add.deposit.year+3);
}
else if(strcmpi(acc_type,"saving")==0)
{
time=(1.0/12.0);
rate=8;
intrst=interest(time,add.amt,rate);
printf("\n\nYou will get $.%.2f as interest on %d of every month",intrst,add.deposit.day);
}
else if(strcmp(acc_type,"current")==0)
{
printf("\n\nYou will get no interest\a\a");
}
}
}
}
int main_exit=1;
if(test!=1)
{ system("cls");
printf("\nRecord not found!!\a\a\a");
see_invalid:
printf("\nEnter 0 to try again,1 to return to main menu and 2 to exit:");
scanf("%d",&main_exit);
system("cls");
if (main_exit==1)
menu();
else if (main_exit==2)
close();
else if(main_exit==0)
see();
else
{
system("cls");
printf("\nInvalid!\a");
goto see_invalid;}
}
else
{printf("\nEnter 1 to go to the main menu and 0 to exit:");
scanf("%d",&main_exit);}
if (main_exit==1)
{
system("cls");
menu();
}
else
{
system("cls");
close();
}
}
void menu()
{ int choice;
printf("\n\n\t\t\t-------------CUSTOMER ++++++++++++++++++++++ACCOUNT creation in BANKING MANAGEMENT SYSTEM-------------");
printf("\n\n\t\t\t -------------WELCOME TO THE MAIN MENU in BANKING MANAGEMENT SYSTEM------------- \t\t\t");
scanf("%d",&choice);
switch(choice)
{
case 1:
new_acc();
break;
case 2:
edit();
break;
case 3:
transact();
break;
case 4:
see();
break;
case 5:
erase();
break;
case 6:
view_list();
break;
case 7:
close();
break;
}
}
int main()
{
menu();
}
Output :
Registering the account :
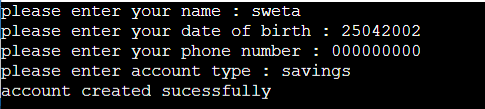
This is how we can register our account , when register account function is called, user would have to give all the necessary details , then his credential will be saved .
Depositing money in account :
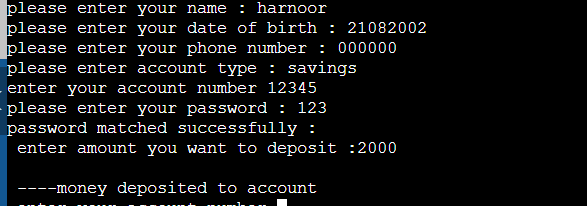
If user wants to deposit money then the deposit function will be called , and then the user has to enter all the necessary details , and if the password matches the user can deposit the amount to his bank account.
Withdrawing money from account :
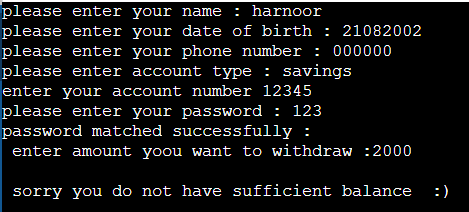
If the user wants to withdraw money then the withdraw function will be called , and then the user has to enter all the necessary details , and if the password matches the user can withdraw the amount from his bank account only if he has sufficient balance left.
Account details :
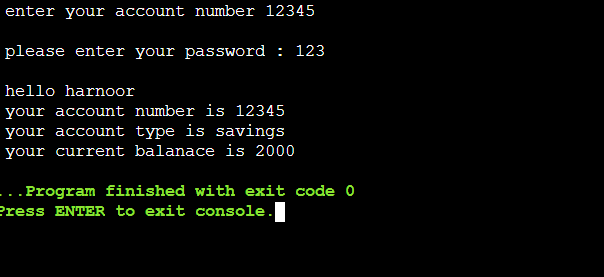
This is kind of balance checking stuff , where the user has to enter the account number and password then he will be able to see details of the current balance of his account.
Deleting account :
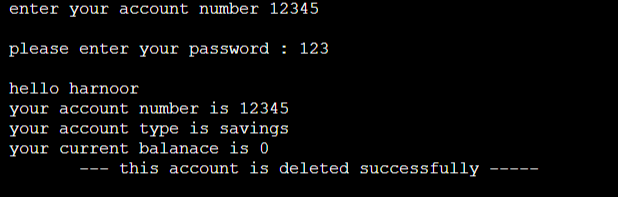
If the user wants to delete an account then the delete function will be called , and then the user has to enter all the necessary details , and if the password matches then the user account will be deleted.
So above the whole code of the bank management system is given, this might seem bulky, but if you try to understand the functions part by part, it would not be tough anymore as each feature is deployed using a function. If we had not created the functions, it might have made the code complicated as you would not be able to understand the different features, and their starting and ending point can also not be understood. All you need to do is just go through each function and try to understand. Each function performs a different task, and all of these are implemented using very basic syntax and operations of the C language. We are taking inputs and keeping them for future reference. We are also deleting the information if any particular customer wants to leave the bank services. We have also implemented the basic deposit and withdrawal feature. It is a simple way of bookkeeping, if the customer is depositing money, then we are just adding that particular to the existing amount, and if the customer wants to withdraw money, then we first check whether the customer has that amount in their account, if not then it throws an error and the program is terminated else it withdraws the money. That particular amount is deducted from the existing amount. The next feature is the seen feature. This feature is simply for account inquiries. If the user wants to check the details of their account, then this feature helps them to do so. The next and last feature is an update. We allow users to update certain values such as phone number and date of birth. So this was all about our bank management mini project. You can try to implement it by yourself. You will gain good knowledge and exception handling skills as you will face some challenges related to basic operations and syntax, so constructing it by yourself would benefit you. You can use this article as a helping resource to build your project.