C Program to Count the Number of Vowels in a String
A string can be defined as a data type that is similar to an integer and also that a floating-point unit is used such that it represents text rather than numbers in a program. It is made up of a series of characters that includes both spaces and integers. For example, we take the words "burger" and "I ate 3 burgers," which are both strings.
As we already know, there are 26 letters in the English alphabet, out of which five of them are vowels (a, e, i, o, u), and the rest are consonants.
The vowels a, e, i, o, and u are to be referred to as the lowercase or the uppercase, and the computer will find and count both. So, in this article we will create different C programs to Count Vowels in a given string.
Count vowels in a string in C
#include <stdio.h>
int main()
{
int c = 0, count = 0;
char s[1000];
printf("Input a string\n");
gets(s);
while (s[c] != '\0') {
if (s[c] == 'a' || s[c] == 'A' || s[c] == 'e' || s[c] == 'E' || s[c] == 'i' || s[c] == 'I' || s[c] =='o' || s[c]=='O' || s[c] == 'u' || s[c] == 'U')
count++;
c++;
}
printf("Number of vowels in the string: %d", count);
return 0;
}
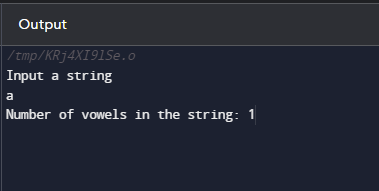
Program to Count the Number of Vowels in a String using Functions
// Counting Vowels using a function
#include <stdio.h>
#include <ctype.h>
int isVowel(char ch)
{
// to reduce the number of or cases of upper / lower cases
// by converting input char to lowercase or uppercase
ch = tolower(ch);
if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u')
return 1;
return 0;
}
int getVowelCount(char a[])
{
int count = 0, i = 0;
char ch;
while (a[i] != '\0'){
ch = a[i];
if (isVowel(ch))
count++;
i++;
}
return count;
}
int main()
{
char str[100];
printf("Enter a string\n");
// way to read string rather than gets/fgets
scanf("%[^\n]s",&str);
// gets(str); is not use
printf("Total Vowels: %d\n", getVowelCount(str));
return 0;
}
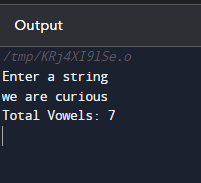
Using Pointers and While Loop
1. Here we consider P as the pointer variable which points to the string s.
2. the loop checks if the first character of the string has existed or not.
a) If it exists then we check if it is in the alphabet or not. If it is an alphabet then check if it is a vowel or consonant. And if it is a vowel then we increase the vowel count otherwise we increase the consonant count.
b) When we increase the p count, then the pointer variable points to the next character of the string. Then we check the while condition for the next character of the string.
We repeat the same step until the character at the pointer variable p becomes null.
3) Lastly we print the vowels and consonant count.
#include <stdio.h>
#include <string.h>
int main()
{
char s[1000],*p;
int vowels=0,consonants=0;
printf("Enter the string : ");
gets(s);
p=s;
while(*p)
{
if( (*p>=65 && *p<=90) || (*p>=97 && *p<=122))
{
if(*p=='a'|| *p=='e'||*p=='i'||*p=='o'||*p=='u'||*p=='A'||*p=='E'||*p=='I'||*p=='O' ||*p=='U')
vowels++;
else
consonants++;
}
p++;
}
printf("vowels = %d\n",vowels);
printf("consonants = %d\n",consonants);
return 0;
}
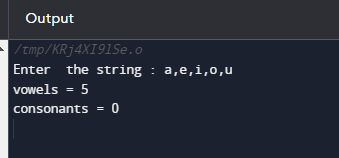
Using Recursion
1. Firstly the main() function calls tries to call the string count(char *s) using the function by passing the string as an argument.
2) The function string count that is (char *s)
Now initialize vowels=0, and consonants=0.
a) If s[i] is null then we print both vowels count and consonants count.
b) And if s[i] is not null then we check that s[i] is an alphabet or not, based on ASCII value.
i) If s[i] is an alphabet then we compare s[i] with vowels and if it is a vowel then we increase the vowel count otherwise we increase the consonant count.
ii) The value of i will be increased by 1. The function will call itself.
And the function calling itself is recursive up to s[i] which might be equal to null.
The Program is given Below:
#include <stdio.h>
#include <string.h>
void stringcount(char *s)
{
static int i,vowels=0,consonants=0;
if(!s[i])
{
printf("vowels = %d\n",vowels);
printf("consonants = %d\n",consonants);
return;
}
else
{
if((s[i]>=65 && s[i]<=90)|| (s[i]>=97 && s[i]<=122))
{
if(s[i]=='a'|| s[i]=='e'||s[i]=='i'||s[i]=='o'||s[i]=='u'||s[i]=='A'||s[i]=='E'||s[i]=='I'||s[i]=='O' ||s[i]=='U')
vowels++;
else
consonants++;
}
I++;
stringcount(s);
}
}
int main()
{
char s[1000];
printf("Enter the string: ");
gets(s);
stringcount(s);
}
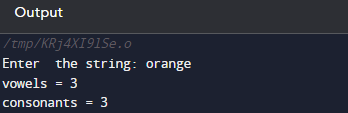