GPA Calculator in C
GPA stands for Grade Point Average. This GPA is used to measure the student's academic performance in educational institutes. By using this, segregation takes place and lets us know how much improvement is needed for the student.
GPA calculation process mathematically
- First, we must initialize grade points for certain grades like A+=10, A=9, B+=8, B=7, D+=6, D=5, and F=4.
- Then give the grades for the individual subjects that you have.
- Take the sum of the grade points and divide this by the number of subjects that you have.
- Then we are going to get GPA for the entire subjects that you have.
Program:
//Calculating GPA for 5 subjects
#include<stdio.h>
int main(){
int mark1, mark2, mark3, mark4, mark5, sum;
float avg, per;
printf("enter marks for subject 1 out of 100: ");
scanf("%d", &mark1);
printf("enter marks for subject 2 out of 100: ");
scanf("%d", &mark2);
printf("enter marks for subject 3 out of 100: ");
scanf("%d", &mark3);
printf("enter marks for subject 4 out of 100: ");
scanf("%d", &mark4);
printf("enter marks for subject 5 out of 100: ");
scanf("%d", &mark5);
sum=mark1+mark2+mark3+mark4+mark5;
printf("obtained sum is: %d", sum);
avg=sum/5;
printf("\nAverage=%0.2f",avg);
per=(sum*100)/500;
printf("\nPercentage=%0.4f", per);
if (per>=90 && per<=100)
{
printf("\nGPA = 4.0");
printf("\nGrade:A+");
}
else if(per>=85 && per<=89)
{
printf("\nGPA = 3.7");
printf("\nGrade:A");
}
else if(per>=80 && per<=84)
{
printf("\nGPA = 3.3");
printf("\nGrade:B+");
}
else if(per>=75 && per<=79)
{
printf("\nGPA = 3");
printf("\nGrade:B");
}
else if(per>=70 && per<=74)
{
printf("\nGPA = 2.7");
printf("\nGrade:B-");
}
else if(per>=65 && per<=69)
{
printf("\nGPA = 2.3");
printf("\nGrade:C+");
}
else if(per>=60 && per<=64)
{
printf("\nGPA = 2.0");
printf("\nGrade:C-");
}
else if(per>=55 && per<=59)
{
printf("\nGPA = 1.7");
printf("\nGrade:D+");
}
else if(per>=50 && per<=54)
{
printf("\nGPA = 1.3");
printf("\nGrade:D-");
}
else if(per>=0 && per<=50)
{
printf("\nGPA = 0.0");
printf("\nGrade:F");
}
}
Output:
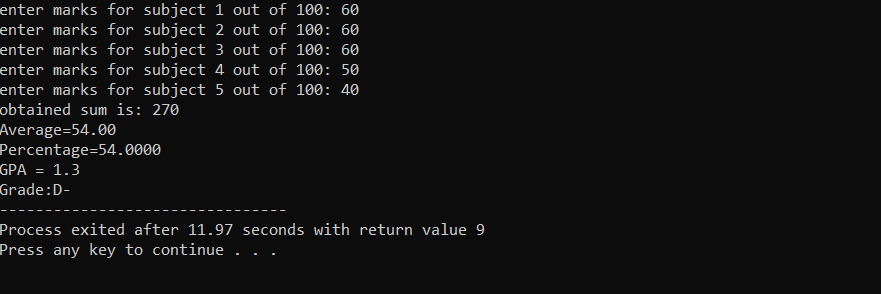
An explanation for the Program:
We used #include<stdio.h> to import standard input and output functions, which are used to take the input from the user and provide output for the user and guide the user on what to enter and prevent the mistake from popping the error. We also make use of comments which is usually text which is not going to be executed by the compiler. C language uses a compiler to convert one form programming language to another form programming language.
A computer or a system does not really read the characters, but it treats them as a symbol and converts them into binary code. We initialized five variables which are mark1, mark2, mark3, mark4, and mark5, used to take input of marks for five subjects. We also initialized three other variables in float datatype, which is used to initialize decimal values, which may be negative or positive, that are avg, and per. Here avg is the average of five marks that are taken as input, and per is the percentage calculating variable which is going to perform a mathematical operation in the variable.
Now we are going to use conditional statements if, else if, else in which we are going to write what to print in the terminal. Every time we have to write if as the first conditional statement and else as the conditional closing statement, at each interval of the percentage values, we are going to print the grade and the GPA.
Application of the GPA calculator:
- In educational institutes like school, universities and colleges, etc., we are going to use the GPA calculator to calculate the GPA for multiple students and that reduces the time for releasing results.
- The government uses this GPA calculator program in their special software that performs mathematical processes by which we can get results of GPA and grades.
Another approach for programming a GPA calculator:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char str[100], chr;
int grade[5], i;
float gradepoints[5], totalgradepoint = 0.0, gpa = 0.0;
printf("Enter the grade in capital letters and gradepoints in integer\n");
for (i = 0; i < 5; i++) {
printf("Subject %d(grade-gradepoints):", i + 1);
chr= getchar();
grade[i] = chr;
scanf("%f", &gradepoints[i]);
getchar();
}
printf("\nSubject - Grade - Gradepoint\n");
for (i = 0; i < 5; i++) {
printf(" %d - %c - %.0f\n", i + 1, grade[i], gradepoints[i]);
}
for (i = 0; i < 5; i++) {
switch (grade[i]) {
case 'S':
gpa = gpa + 10 * gradepoints[i];
totalgradepoint = totalgradepoint + gradepoints[i];
break;
case 'A':
gpa = gpa + 9 * gradepoints[i];
totalgradepoint = totalgradepoint + gradepoints[i];
break;
case 'B':
gpa = gpa + 8 * gradepoints[i];
totalgradepoint = totalgradepoint + gradepoints[i];
break;
case 'C':
gpa = gpa + 7 * gradepoints[i];
totalgradepoint = totalgradepoint + gradepoints[i];
break;
case 'D':
gpa = gpa + 6 * gradepoints[i];
totalgradepoint = totalgradepoint + gradepoints[i];
break;
case 'E':
gpa = gpa + 5 * gradepoints[i];
totalgradepoint = totalgradepoint + gradepoints[i];
break;
default:
printf("wrong grade\n");
exit(0);
}
}
printf("GPA: %f\tgradepoint: %f\n", gpa, totalgradepoint);
gpa = gpa / totalgradepoint;
printf("Total GPA for your score: %0.2f\n", gpa);
return 0;
}
Output:
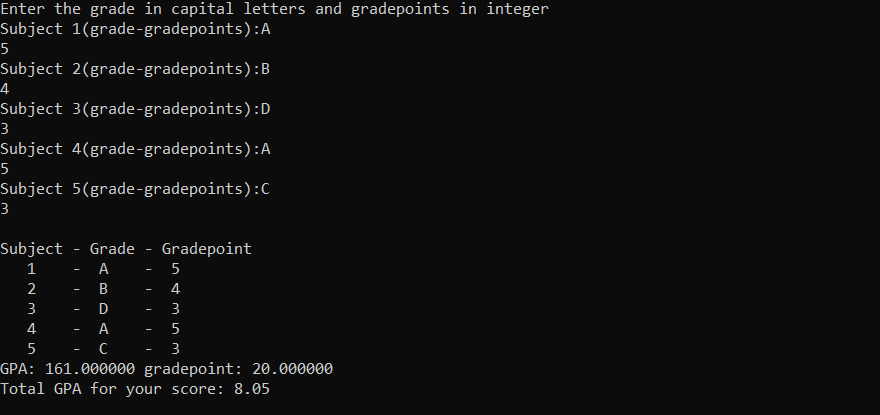
An explanation for the above Program:
In the above code, we used the switch concept, which replaces conditional statements and acts the same as conditional statements. We have to enter a grade and grade points, we are entering for five subjects, and we used for loop to take string value and integer value to enter the data, which makes code more efficient to work.