Use of Structure in C
We can usually use arrays in C programming to store elements of the same data type. We can use strings to store multiple elements of a character data type. Still, there is a programming need to store multiple items of different data types, for example, when collecting data from students whose names are of the character data type. The second type of data that a roll number has is a number.
Structures
This structure is used to store multiple elements of different data types. The structure can contain "n" elements. This means any number of elements. Now let's look at the syntax.
Syntax
struct structurename
{
datatype ele1;
datatype ele2;
.
.
.
.
};
We should always start with the keyword "struct" and add the struct name that the programmer can want. Next, we need to open the square bracket declaration. Finally, close the parentheses after declaring all the elements with the data type. It is important to add a semicolon at the end.
Syntax Example
struct Customer
{
int customerid;
char name[20];
};
main()
{
int a;
Struct Customer c[25];
}
When we declare a variable in the main function, we need to add the data type and then the variable name. For structures, the data type name is "struct structurename" or "struct Customer". Next, we need to add the variable name "c". This allocates memory for the variable. Structure variables always containa base address. This refers only to the data type, but sinceit is an internal pointer value, there is no pointer here. A " c " structural variable is allocated 24 bytes of memory [20]. Each character occupies 1 byte of memory. Characters mean 20 bytes for 20 characters, and integer variables are 4 bytes on 64-bit systems for 24 bytes. We have saved the information so far. Let's move to the access part.
Accessing
We can access the structure simply by entering the dot ".". Operators such as "c.customerid" and "c.name". Before we go any further, we need to know how to declare the size of the structure.
Size of Structure
We can use the keyword "sizeof" to find the size, sizeof (variable name). We can also use sizeof (struct structname). Check out the examplesbelow to get a quick idea.
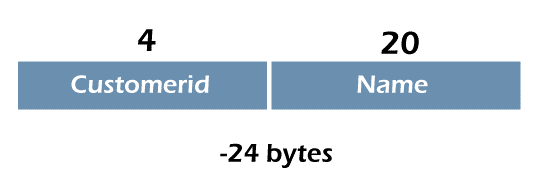
#include<stdio.h>
struct Customer
{
int customerid;
char name[20];
};
void main()
{
struct Customer c;
printf("size of Customer structure is %lu \n",sizeof(c));
printf("size of Customer is %lu ",sizeof(struct Customer));
}
Output:
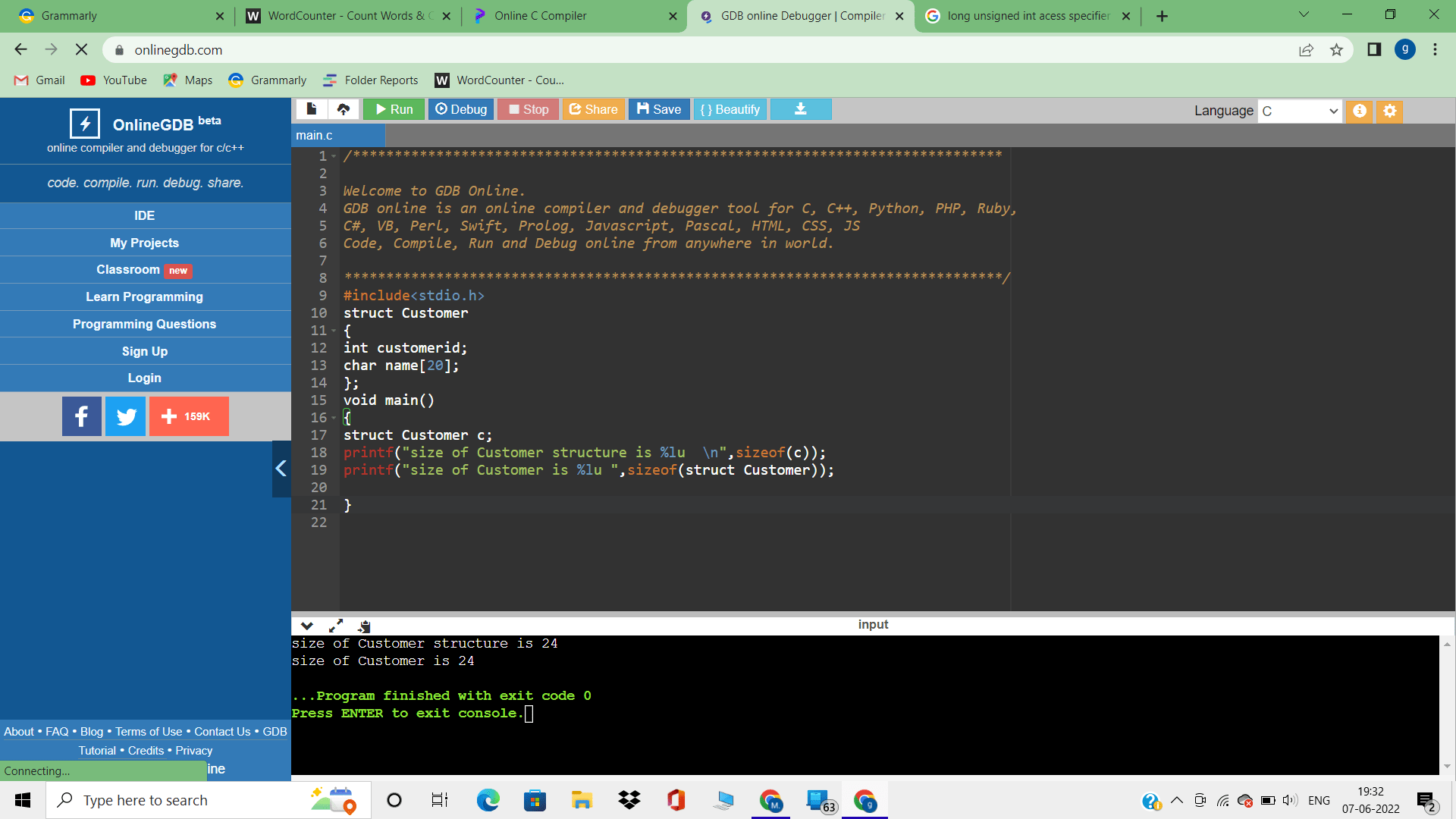
We used "% ul" here. This represents an unsigned long or unsigned int. We can also use “%d”, but the C compiler will display a small warning. Finally, sizeof () allows us to pass either a variable name or a data type name. Next, let's move on to the typeof structure.
Types of Structures
There are two maintypes of structures.
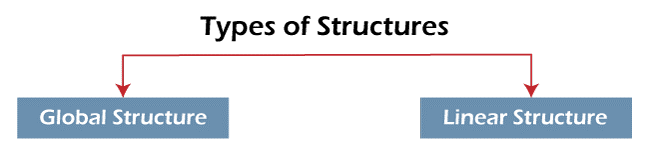
1. Global Structure
The structure definition, which is common for all functions that are entire programs is called global structure.
Global Structure Declaration
#include<stdio.h>
struct globalstruct
{
int a,b;
};
void main()
{
struct globalstruct g;
}
int try()
{
struct globalstruct l;
//It also executes here
}
Output
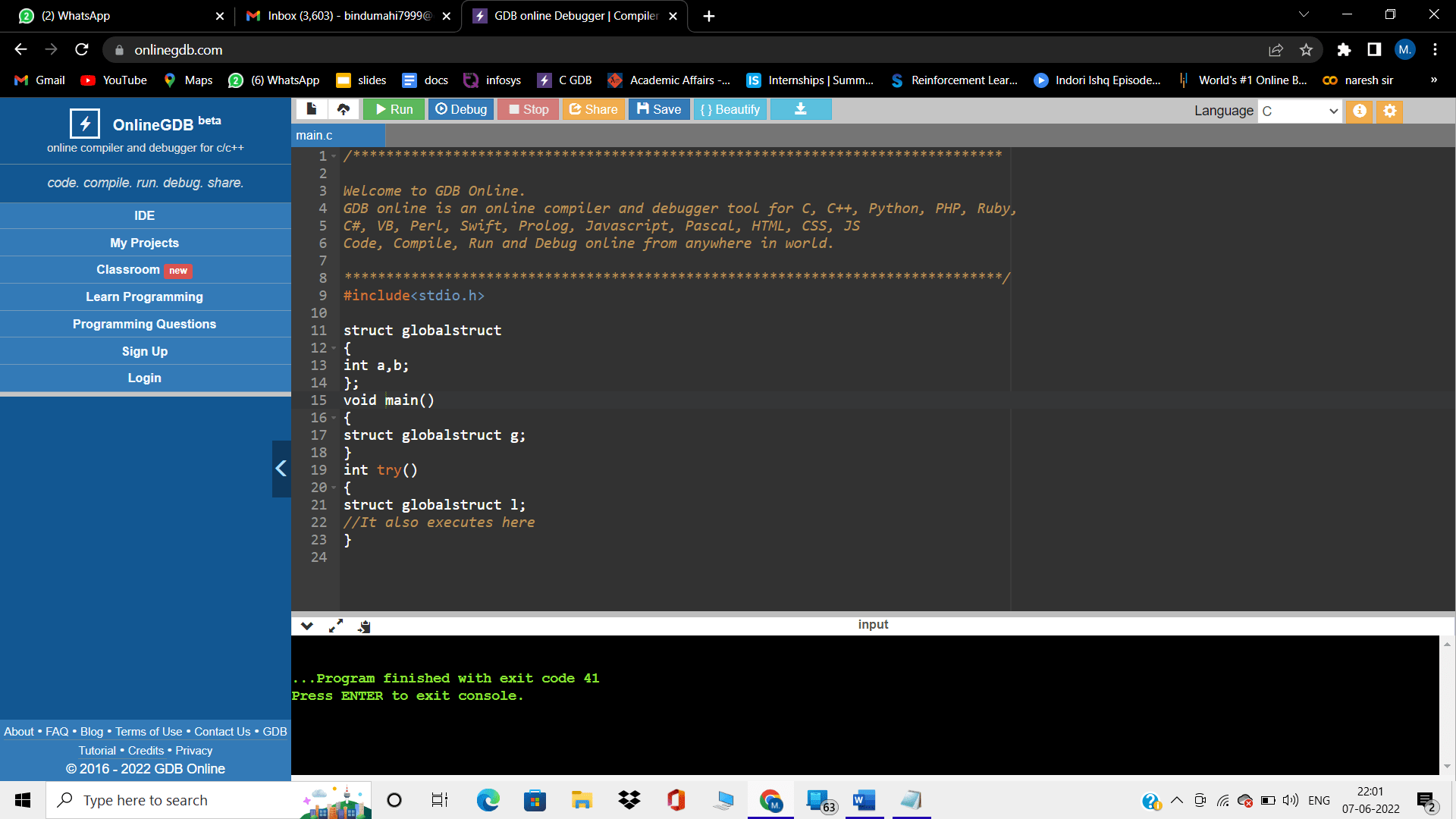
Here the program is executing successfully.
2. Local Structure
The structure which is only for a specific function is called Local structure. Here, is the declaration of structures inside a particular function.
Declaration of local structure
#include<stdio.h>
void main()
{
struct localstruct
{
int a,b;
};
struct localstruct l;
//It will work here
}
int try()
{
struct localstruct l;
//It won't execute here
}
Output:
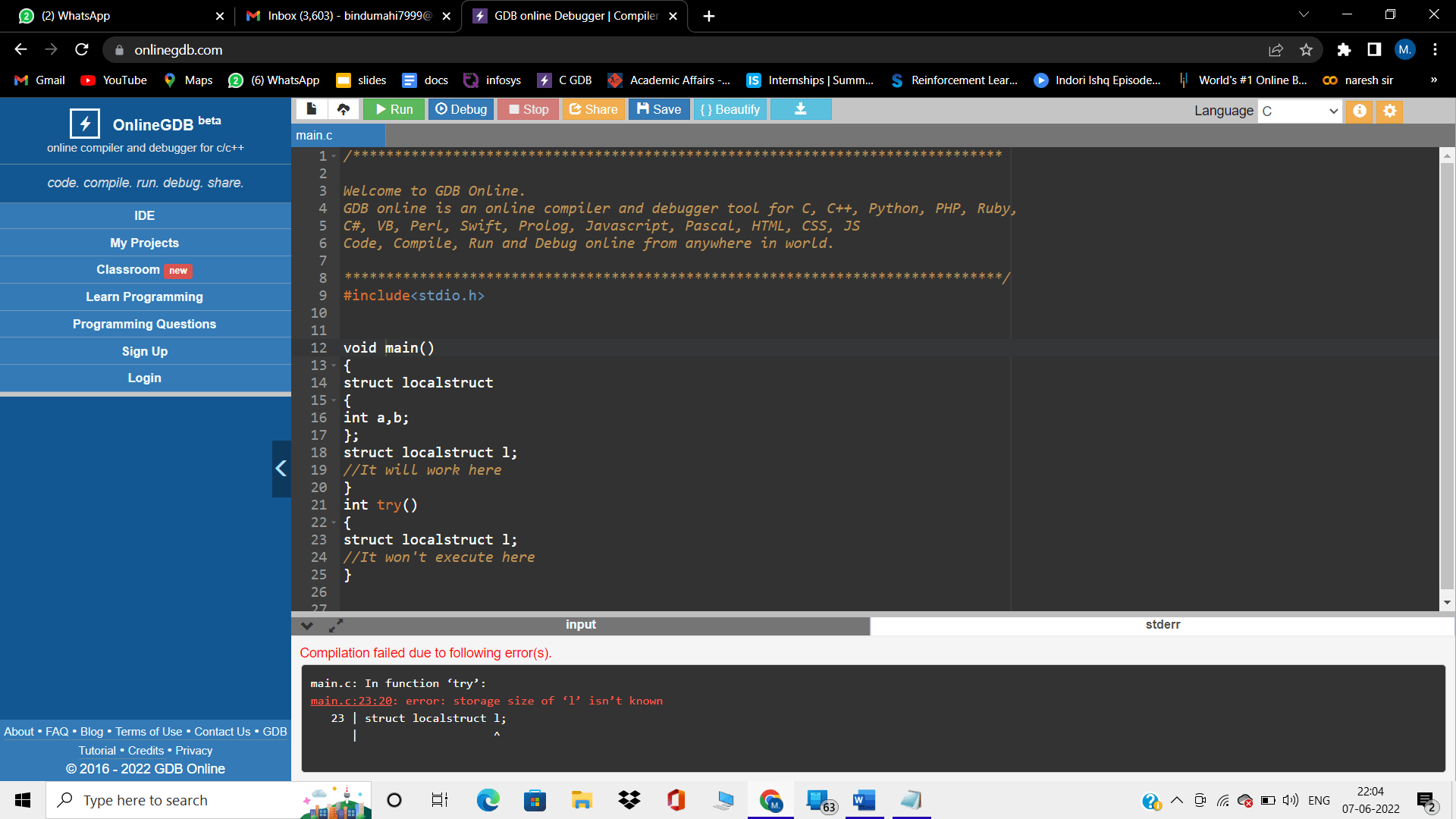
Here we can access it only with the main function. That is why we got compilation error that “l” is not known. Simply it is like local and global variables concept.
Well, each type has its own application and advantages but both save time and helps in efficiently data efficiently.
Advantages of structures
1. Unlike arrays and strings, it is possible to create data types with different data types. For example, if we want to keep student records, including student names, roll numbers, grades, and addresses, the items can be of different types. For example, the roll number is an integer, the name is a string, the grade is a floating-point, and the address is a string.
2. Reduce complexity and increase productivity.
3. Because it is heterogeneous, it eliminates the heavy load of saving to different files.
4. Code maintainability is improved
5. Very suitable for some math operations