Do-While Loop in C
Both 'While' and 'Do-While' loops in C mostly have a similar concept, and the code of both loops runs for mainly similar purposes in the same manner. But, there is a sole and significant difference between them. In the do-while loop, the execution of the body takes place at least one time before checking the termination condition. Then it executes the command according to the termination condition. Whereas, in the case of the while loop, the termination condition is checked before the execution of the body.
In other words, in the do-while loop, the body is executed first, and after that, it checks whether the termination condition results in true or false. In contrast, in the while loop, it first executes the termination condition and checks whether the results in true or false. If the result is True, then the statement is executed, otherwise not.
Syntax of the do-while loop in C:
do
{
/* main body used for the loop */
}
while (termination condition);
Working of the do-while loop in C
- Initially, the body of do-while executes only one time. After that, the control executes the termination condition.
- It then checks whether the termination command results in true. If yes, it will print the desired outcome on the screen, and it will continue to execute the termination condition again and again.
- One more thing is that the execution will run multiple times until the condition become false.
- As soon as the results become false after the execution of the termination command, then the loop will be automatically dismissed.
Representation of do-while loop with a flow chart:
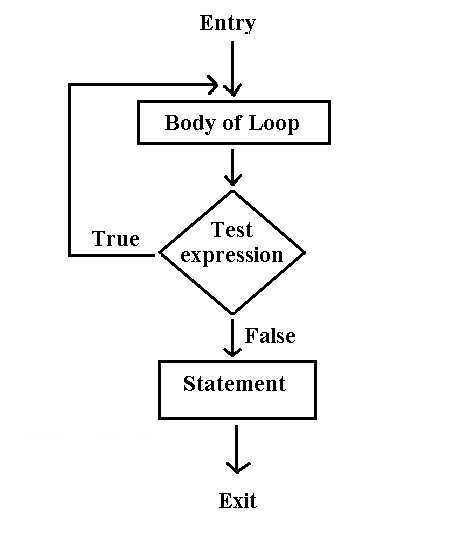
Example 01: Program of do-while loop:
/* code for adding integers until the user assigns the value zero */
#include <stdio.h>
int main()
{
double number, total = 0;
do
{
printf("Enter a number: ");// body of do-while loop
scanf("%lf", &number); // it is executed only once
total += number;
}
while(number != 0.0);
printf("Sum = %.2lf",total);
return 0;
}
Output:
Enter a number: 3
Enter a number: 4
Enter a number: -5
Enter a number: 6
Enter a number: 0
Sum = 8.00
Full explanation:
In the above program, we used the do-while loop, which processes the code in the following manner:
It lets the user enter the numbers, and it will be executed until the user enters 0. If the condition becomes false, then the loop terminates, and the code adds all the entered numbers before zero and displays the sum of those numbers on the screen.
Let's see a few examples of a do-while loop.
Example 02: Program of do-while loop:
/* this is a program to print numbers to a specific point */
#include <stdio.h>
int main()
{
int a;
printf("enter the number (less than 3) to start from:\n");
scanf("%d",&a); // assigning the value entered to a
do //execute the task without checking the condition only once
{
printf("the value of a is %d\n",a);
a++;//increment statement
}
while(a<=3);//this is termination condition
return 0;
}
Output:
enter the number (less than 3) to start from:
-3
the value of a is -3
the value of a is -2
the value of a is -1
the value of a is 0
the value of a is 1
the value of a is 2
the value of a is 3
Example 03: Program of do-while loop:
/* this program will calculate the sum of all multiples (10)
of the number entered by user */
#include <stdio.h>
int main()
{
int num ,sum, a=0;//initialsing the value of a
printf("enter the table you want to add:\n\n");
scanf("%d",&num);//assigning the value of a entered by user
do
{
printf("%d * %d = %d \n\n",num,a,num * a);
a++;// increment statement
}
while(a<=10);// termination condition
sum=num*55;
{
printf("the sum of all multiples of this number is %d\n\n",sum);
}
return 0;
}
Output:
enter the table you want to add:
8
8 * 0 = 0
8 * 1 = 8
8 * 2 = 16
8 * 3 = 24
8 * 4 = 32
8 * 5 = 40
8 * 6 = 48
8 * 7 = 56
8 * 8 = 64
8 * 9 = 72
8 * 10 = 80
the sum of all multiples of this number is 440