Entry Control Loop in C
Initially, an entry control loop verifies the termination condition at the entry point. After that, its control passes to the main body of the while or for loop if the termination condition or test expression becomes true. This kind of loop usually regulates the ‘while’ and ‘for’ loop entrance. Due to this, it is known as an entry control loop in C.
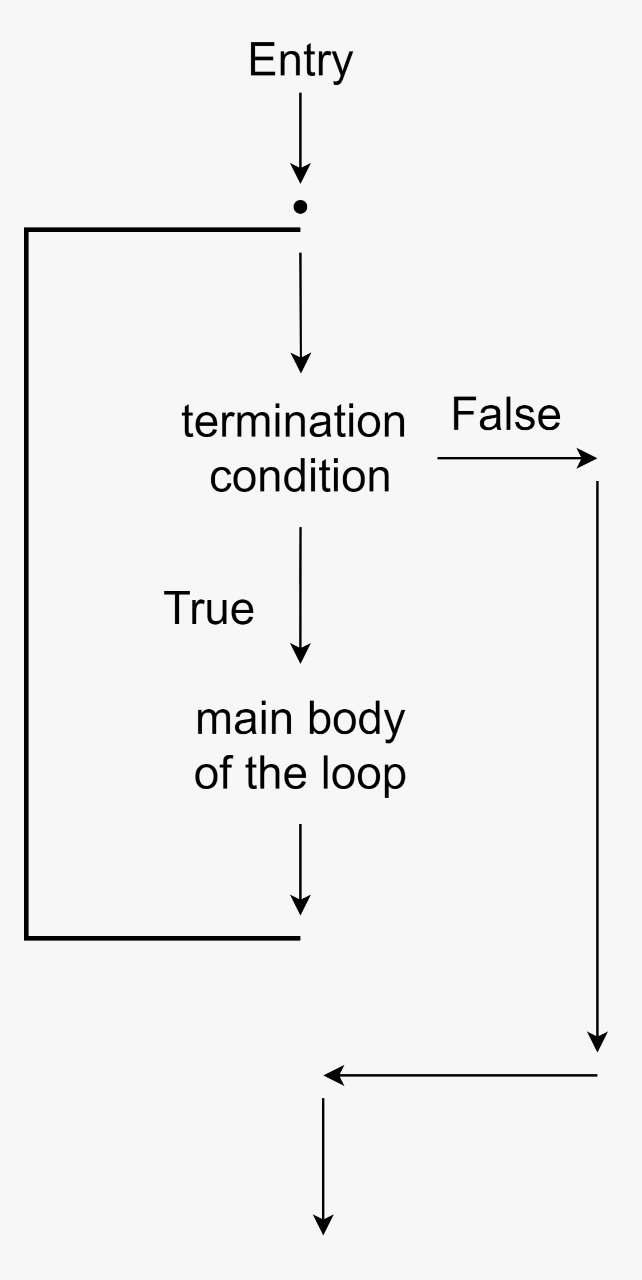
Diagram: Entry control loop in C
While loop:
C’s most straightforward looping form is none other than the “while” loop. The “while” loop is a looping entry control arrangement.
The “while” loop is used to run the program command continuously until the provided termination condition is false.
The specified test expression or termination condition will be examined at the time of entrance in the “while” loop, and if it finds out to be true, then the control will be moved to the main body of the “while” loop.
After that, the statements written within the main body of the “while” loop will be executed.
The counter will change the value to enter in the while loop, and the specified test expression/ termination condition will be evaluated once again. This procedure will be repeated until the supplied phrase is found to be false. The "while" loop consists of the termination condition portion in starting.
That's why the while loop is called the entry-controlled loop in C.
Syntax:
while(termination condition)
{
// main body of the "while" loop
}
while( i <= 10) // initialization statement
{
printf("\n Value of I is %d", i) ;
i++ ; // modifying (increment/ decrement statement) counter value
}
Program:
#include "stdio.h"
#include "conio.h"
void main()
{
int n, i, ans; // declaring integers
clrscr();
printf("\n Enter No. to print Table : ");
scanf("%d", &n); // assigning value to n entered by user
i = 1; // setting 1 as the counter value
while(i <= 10) // termination condition
{
ans = n * i;
printf("\n %d * %d = %d", n,i, ans) ;
i++; // modifying (increment/ decrement statement) counter value
}
getch();
}
Example:
For loop:
C's most powerful and versatile looping structure is none other than the “for” loop. With the help of the “for” loop, we may easily accomplish any difficult task in C.
Syntax:
for (initialization statement; termination condition; increment/decrement statement)
{
// main body of the "for" loop in C
}
There are three main parts of the "for-loop", which are mentioned below:
1. Initialization statement,
2. Termination condition, and
3. Increment or decrement statement.
Initially, when the execution of the loop takes place, a counter variable is initiated with the help of the initialization statement. After that, the termination condition, which is given inside the expression section, is examined.
If the termination condition finds to be true, the control will be automatically entered into the main body of the “for” loop. And, at last, the program statements written inside the “for” loop would likely be executed.
In the last step, the control will be transferred to the third portion to modify the counter value (either for incrementing or decrementing the value).
The counter value will be evaluated through the termination condition after the modification of the value and will check that the control can enter into the "for" loop for execution.
The entire procedure explained above will run again and again until and unless the result of the termination condition becomes false.
When the test expression returns a false value, the control will skip the whole "for" loop and executes the statement line which has been written just after the 'for' loop.
Example:
for (i=0; i<5; i++)
{
printf("Hello world!!!") // main body of the "for" loop in C
}
Program:
The following program enables the user to print a multiplication table of a natural number with the help of the “for” loop.
#include "stdio.h"
#include "conio.h"
void main()
{
int n, a, ans; // declaring variables
clrscr();
printf("\n Enter No. to print Table : ");
scanf("%d", &n); // assigning value to a entered by the user
/* for(initialization condition,
termination condition, increment or decrement condition) */
for(a = 1; a <=10 ; a++ )
{
ans = n * a;
printf("\n %d * %d = %d", n,a, ans) ;
}
getch();
}