Advantages of Dynamic Memory Allocation in C
Dynamic Memory Allocation
Dynamic memory allocation is a process of assigning or allocating memory to a variable during the execution of a program. Dynamic memory allocation provides storage according to the user's need and also helps to reduce the wastage of memory.
Different ways to allocate memory dynamically:
- Malloc() – this function allocates a block of memory and returns a pointer to the first byte of the allocated space.
- Calloc() – this function allocates memory for n number of elements and returns a pointer to the memory
- Realloc() – this function allocates new memory for previously allocated memory.
- Free() – this function free up space acquired by some pointer.
Malloc () function
Malloc allocates a block of memory and returns a pointer to the first byte of allocated memory. It returns NULL if requested memory cannot be allocated.
Syntax:
Ptr = (data type *)malloc(size);
Example-
A = (int *)malloc(10 *sizeof(int));
In this, a memory block of 10 * 4(size of integer) will be allocated, and the pointer 'a' will point to the first byte of allocated memory.
Code example:
#include <stdio.h>
#include <stdlib.h>
int main()
{
int i, n, *p;
printf("Enter number of elements: ");
scanf("%d", &n);
p=(int*)malloc(n * sizeof(int)); //memory allocated using malloc
if(p == NULL) {
printf("memory cannot be allocated\n");
}
else {
printf("Enter elements in array:\n");
for(i=0; i<n; i++) {
scanf("%d",&*(p+i));
}
printf("Elements of array are\n");
for(i=0; i<n; i++) {
printf("%d ",*(p+i));
}
return 0;
}
}
OUTPUT
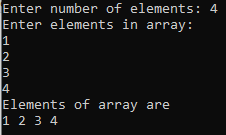
Calloc () function
The callocfunction allocates more than one memory block, each of the same size. It is the same as the malloc function, but the only difference is it takes two arguments: the number of elements and size.
Syntax-
ptr = (data type *)calloc(elements, size);
Example-
x =( int *)calloc(10, sizeof(int));
In this, 10 blocks of memory are allocated of the size of integer 'x'
pointer will point at the first block of memory.
Code example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int count, i, *ptr;
printf("Enter number of elements: ");
scanf("%d", &count);
ptr = (int*) calloc(count, sizeof(int));
if(ptr == NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
printf("Enter elements: ");
for(i = 0; i < count; i++) {
scanf("%d", ptr + i);
}
return 0;
}
OUTPUT
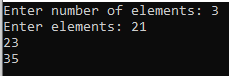
Free () function
Free function frees up the space/ memory allocated previously by calloc or malloc.
Syntax-
Free(ptr);
Example-
Free(x);
Code example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int* ptr, n;
printf("Enter number of Elements: ");
scanf("%d", &n);
ptr = (int*)malloc(n * sizeof(int));
if (ptr == NULL) {
printf("Memory not allocated \n");
exit(0);
}
free(ptr);
printf("Successfully freed memory using free function.");
return 0;
}
OUTPUT

Realloc () function
The realloc function is used to alter the size of memory allocated previously. It is helpful if a user needs more memory during the execution of a program.
Syntax-
realloc(pointer, size);
Example-
x =( int *)calloc(10, sizeof(int));
x= (int *)realloc(x, 100);
In this, the realloc function increases the memory for 'x' pointer to 100 bytes.
Code example:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main () {
char *str;
str = (char *) malloc(15);
strcpy(str, "burger");
printf("String = %s, Address = %u\n", str, str);
/* Reallocating memory */
str = (char *) realloc(str, 25);
strcat(str, ".com");
printf("\nString = %s, Address = %u\n", str, str);
free(str);
return(0);
}
OUTPUT
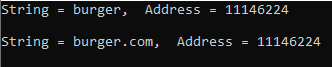
Advantages of dynamic memory allocation
- Allocation of memory
In static memory allocation, space is provided at compile time, resulting in much memory waste. But in dynamic memory allocation, memory is assigned at run time so that the memory can be used accordingly with zero wastage of memory. - Efficiency
Dynamic memory allocation is more efficient than static memory and flexible. - Reusability of memory
The previously used memory can be used again using the free () function. This function frees the memory allocated before to use in the future. - Memory Reallocation
In static memory allocation, we cannot change the size of any data structure if memory is allocated. But in dynamic memory allocation, we can change the size at our convenience by using the realloc() function. - Cost-effective
Dynamic memory allocation is cost-effective as no extra cost is required for memory because memory is allocated per the user's needs.