Free() Function in C
Free() function is a built-in function which is define in the stdlib.h header file. If we want to use this function in our program, we must include the stdlib.h header file. The purpose of this function is to deallocate the memory block. The free() function is one of the functions in the concept called dynamic memory allocation. The memory allocated using malloc(), calloc() or realloc() functions is deallocated using free() function.
The function deallocates the memory and returns the memory to the heap. We need to deallocate memory because our computed contains a limited amount of memory, so we cannot run the infinite program in it. In the case of automatic allocation, the computer takes care of the free-up, but in the case of dynamic memory allocation, we need to free up the heap memory.
In C, the memory of a variable is deallocated automatically at compile time, but in the case of dynamic memory allocation, we need to deallocate the memory explicitly. In case we do not deallocate the memory, we may encounter out-of-memory errors. We have to free the memory because another process can use the use the same block of memory.
Syntax
void free( void *ptr);
ptr is the address of the memory block which has previously been allocated using malloc(), calloc() or realloc() function.
Parameter
The free () function takes a pointer variable as a parameter or the address of the memory, which we have to deallocate. Pointer variable is a variable which stores the address of another variable. We can pass only the address or pointer variable. We cannot pass ordinary variables or numerical values.
Return value
Free() function won't return any value. It deallocates or frees up the memory which has been previously allocated.
Example Programs on Implementation of Free() Function
// program to deallocate the memory using the free() function, which has previously allocated
#include<stdio.h>
#include <stdio.h>
int main() {
int* ptr; // declaring the pointer variable
ptr = malloc(10 * sizeof(*ptr)); // memory allocation to ptr using malloc() function
// dynamically
if (ptr != NULL) // checking the memory is allocated or not
{
/* if the memory is allocated, then the ptr stores the address of the memory block, so we gave the condition as ptr ! = NULL */
*(ptr) = 100; // Assigning the value to the memory block
// printing the assigned values to the memory block
printf(" \nThe assigned to the memory block is = %d",*(ptr));
}
free(ptr); // deallocating the memory of ptr using free() function.
}
Output:
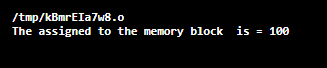
Example 2:
// program to implement the free() function using calloc function
// program to read and print the array elements using dynamic memory allocation
#include <stdio.h>
#include <stdlib.h>
int main()
{
int* p; // pointer variable declaration
int n;
printf(" \nEnter the size of the array: ");
scanf("%d",&n); // reading the size of the array
// allocating the memory using calloc() function
p = (int*) calloc(n, sizeof(int));
if(p == NULL) // checking whether memory is allocated or not
{
printf(" \nMemory not allocated \n");
} // if
else
{
printf(" \nMemory allocated successfully: ");
printf(" \nEnter the array Elements: ");
// Reading the array elements
for (int i=0; i<n; i++)
{
scanf("%d", &p[i]);
}
printf(" \nThe elements of the array are: ");
// printing the array elements
for (int i=0; i<n; i++)
{
printf("%d ", p[i]);
}
} // else
free(p); // deallocating the memory of p using free() function
return 0;
} // main
Output:
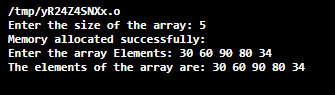
Example 3:
// program to show the implementation of free() function in strings
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main ()
{
char *ptr; // declaring the pointer variable
// dynamic memory allocation to the ptr using malloc() function
ptr = (char *) malloc(15);
strcpy(ptr, "JavaTpoint"); // copying the string in ptr using string handling function
// printing the string and address of the memory block
printf("String = %s, Address = %u\n", ptr, ptr);
/* Increasing the memory size by reallocating the memory using realloc() function */
ptr = (char *) realloc(ptr, 25);
strcat(ptr, ".com"); // adding .com to the string using string handling functions
// printing the updated string and its address
printf("String = %s, Address = %u\n", ptr, ptr);
/* Deallocate allocated memory */
free(ptr);
return(0);
}
Output:
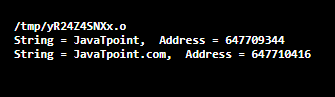
If we see the output, both statements' address is different. By this, we can conclude that the address is not stable. It changes at every instance. If we run this program again we get another address.
Conclusion
In this article, we learned about the free() function in C, the detailed information of free() function parameter passing and return type in free() function. We discussed the syntax of the free() function. We did examples of the free() function and dynamic memory allocation, and deallocation of memory. Examples on free() function using malloc(), calloc() and reallloc() function.