Calendar application in C
Introduction to the calendar application
We all are familiar with the calendar. It plays a crucial role in our daily life. We run toward the calendar whenever we want to know today's date or what day it is. We also run towards the calendar when we want to check holidays and vacations. Nowadays, everything has been digitalized. We barely use physical calendars, but we all have at least one calendar at home. We use our mobile phone to check the date and days, and nowadays it is also visible on the lock screen. Mobile phones and desktops consist of the calendar application from the very beginning. When everything was not digitalized as there were still calendar applications, today we will understand how calendar apps can be made by just using the c language. Let us understand the features and the blueprint of the whole application.
Features of the calendar application: here, we will make a calendar for any specific year, and the year will be taken as per the user's choice. Suppose the user enters 2000, then we will make a calendar for 2000.
We have to specify months and days accordingly, which should be in proper calendar format. So we have to take care of the user interface and the logic behind it. So let's code.
Code for the calendar application
calender_app.c
#include<stdio.h>
#define TRUE 1
#define FALSE 0
int days_in_months[]={0,31,28,31,30,31,30,31,31,30,31,30,31};
char *months[]=
{
" ",
"\n\n\nJanuary( jan)", // this will show the januray month name at the top of the dates
"\n\n\nFebruary (feb)",// this will show the February month name at the top of the dates
"\n\n\nMarch (march) ",// this will show the march month name at the top of the dates
"\n\n\nApril (april)",// this will show the april month name at the top of the dates
"\n\n\nMay(may)",// this will show the may month name at the top of the dates
"\n\n\nJune (june)",// this will show the June month name at the top of the dates.
"\n\n\nJuly ( july) ",// this will show the July month name at the top of the dates.
"\n\n\nAugust (august)",// this will show the august month name at the top of the dates
"\n\n\nSeptember (sept)",// this will show the september month name at the top of the dates
"\n\n\nOctober (October) ",// this will show the October month name at the top of the dates.
"\n\n\nNovember ( nov)",// this will show the November month name at the top of the dates
"\n\n\nDecember (dec )"// this will show the December month name at the top of the dates.
};
int inputyear(void)
{
int year_entered;
printf("Please enter a year (example: 2000 ) : ");
scanf("%d", &year_entered);// this is for taking the year input from the user
return year_entered;
}
int determine_day_code(int year_entered)
{
int day_code;
int d1, d2, d;
d = (year_entered - 1.)/ 4.0;
d1 = (year_entered - 1.)/ 100.;
d2 = (year_entered - 1.)/ 400.;
day_code = (year_entered + d - d1 + d2) %7; //this is for the calculations of proper day code
return day_code;
}
int determine_leap_year(int year_entered)
{
if(year_entered% 4 == FALSE && year_entered%100 != FALSE || year_entered%400 == FALSE)
{
days_in_months[2] = 29;
return TRUE;
}
else
{
days_in_months[2] = 28;
return FALSE;
}
}
void calendar_application(int year_entered, int daycode)
{
int month, day;
for ( month = 1; month <= 12; month++ )
{
printf("%s", months[month]);
printf("\n\nSun Mon Tue Wed Thu Fri Sat\n" );
// this is to Correct the position for the first date of the calendar_application
for ( day = 1; day <= 1 + daycode * 5; day++ )
{
printf(" ");
}
// this is for Printing all the dates for one month of the calendar_application
for ( day = 1; day <= days_in_months[month]; day++ )
{
printf("%2d", day );
//this is for checking. Is the day before Sat? If yes, then executed Else, start next line Sun will be executed
if ( ( day + daycode ) % 7 > 0 )
printf(" " );
else
printf("\n " );
}
//this is for Setting a position for next month in the application
daycode = ( daycode + days_in_months[month] ) % 7;
}
}
int main(void)
{
int year_entered, daycode, leapyear;
year_entered = inputyear();
daycode = determine_day_code(year_entered);
determine_leap_year(year_entered);
calendar_application(year_entered, daycode);
printf("\n");
}
Output: here, only a few months are shown in the output. If you run the code above, you can witness that it will show you the calendar from January to December, which will be in proper format as shown below. Please check the output attached below.
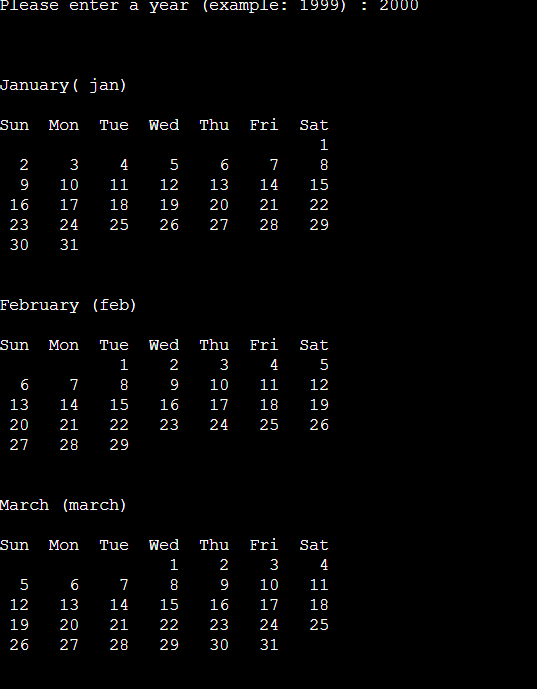
Explanation: Let us understand the code part by part for better understanding.
Declaring the total number of days as it stays fix
int days_in_months[]={0,31,28,31,30,31,30,31,31,30,31,30,31};
Here we have provided the total number of days for each month, we know no matter what this will remain fixed for every year, but there is a problem as you know February is different from the rest of the month, there are two reasons first it has mostly 28 days second sometimes it has 29 days if the year is a leap year, we need to check whether the year entered by the user is a leap year or not, if yes then we have to add 29 days in February instead of 28, but here we have given 28 as most of the times it remains 28.
Checking for leap year :
int determine_leap_year(int year_entered)
{
if(year_entered% 4 == FALSE && year_entered%100 != FALSE || year_entered%400 == FALSE)
{
days_in_months[2] = 29;
return TRUE;
}
else
{
days_in_months[2] = 28;
return FALSE;
}
}
So once the user enters the year's value, we call for this function, and then we check whether the year is divisible by particular values. If it results true, we manipulate the second value of the days_in_months array, and if it turns out to be false, then we let it be 28 as the year is not a leap year. So this is how we deal with the problem of the total number of days in the leap year, and we produce a calendar for the leap year.
Printing the day code( name of weekdays )
// this is for Printing all the dates for one month of the calendar_application
for ( day = 1; day <= days_in_months[month]; day++ )
{
printf("%2d", day );
//this is for checking. Is the day before Sat? If yes, then executed Else, start next line Sun will be executed
if ( ( day + daycode ) % 7 > 0 )
printf(" " );
else
printf("\n " );
}
//this is for setting a position for next month in the application
daycode = ( daycode + days_in_months[month] ) % 7;
}
So we all know there are a total of 7 days in a week. They follow from Sunday to Saturday, and we must manage proper day codes for each day. It is also a crucial step that must be done carefully. We check the last day of the previous month, and then it is divided by 7 to get day codes for the next month. It is a conventional method to produce the correct day code; this same method is used in various aptitude problems to find the correct day code of that particular day. It is a mathematical calculation.
Lastly, we call every function and thus, it produces the required calendar.
year_entered = inputyear();
daycode = determine_day_code(year_entered);
determine_leap_year(year_entered);
calendar_application(year_entered, daycode);
printf("\n");
We ask for the year from the user, and when a user enters it, we pass the year's value in our functions, and the calendar is produced.
So, this was brief about our calendar application, which can also be added to the resume to show proficiency in the C language. We can even build an app by this logic of calendar generation by improving the user interface and adding a few more functionalities to this project.