Structure in C
Why Structure came into the picture?
In the actual world, we deal with entities that are collections of objects rather than tiny bits of data like integers, characters, floats, etc. So, if we want to deal with such type of entities, like a student, so, in that case, we will need different types of data like the student’s name, Student's roll no, student ‘s percentage, etc.
Therefore, in this circumstance, we require a data type that can store different types of data, including character, integer, float, and others, in a single variable.
So, as a solution, C provides a data type called Structure for dealing with such types of distinct or even similar types of data.
What is Structure?
A structure is a keyword that produces customized or non-primitive data types.
It is a grouping of different or comparable types of data.
When we define a structure, we create a new data type that is user specified.
No memory is consumed in defining Structure.
When we build variables using user-defined data types that are formed at the time of defining a structure, these variables take some memory space which depends upon the member variables.
Moreover, Struct is a keyword that defines a structure and creates new data types.
How to Create a Structure?
Syntax:
struct book
{
int book_pages;
char book_name;
float book_price;
};
- We use the struct keyword for defining structure.
- Here book is the user-defined data type created by the struct keyword.
- Three variables, book_pages, book_name, and book_price, are enclosed in curly brackets and have the data types int, char, and float, respectively.
- The semicolon (;) follows the last curly bracket to end the sentence.
In this way, a structure is created.
How to Declare a Structure variable?
A Structure variable can be declared in one of two ways.
- We can declare variables after the structure definition.
struct date
{
int d,m,y;
} date1;
We can declare multiple variables with the separation of commas in this way
struct date
{
int d,m,y;
} date1,date2,date3;
- We can declare variables after structure declaration using the struct keyword.
struct player
{
char name[50];
int phone_no;
};
int main()
{
struct player Hrithik;
return 0;
}
In the above syntax,
- we declared a variable “Hrithik” using the struct keyword.
- Here data type is “player”.
Initializing of Structure Variable:
1. We can initialize a structural variable at the moment of declaration, just like any other variable in C.
Like an array, we assigned our values with the separation of commas in curly braces.
Syntax
struct student
{
char student_name;
int student_rollno;
float student_percentage;
}s1 ={“Omshiv”,72,96.2};
2. In initialization, we always remember assigning the values to the variable's name respectively.
We can also initialize the variable after the structure declaration
Syntax
struct student
{
char student_name;
int student_rollno;
float student_percentage;
};
int main()
{
struct student s1 ={“Omshiv”,72,96.2};
}
How to access structure elements?
Let's look at how to access the structure's components as now the structure type and structure variables have been declared.
Using a subscript, we can access specific array elements in arrays. But in the case of structure, for accessing the element or member variables, we use the dot(.) operator.
Member variables have no identity; they are present in the structure variable. So, to target them, we use the dot operator.
Examples: Let’s take some examples for better understanding:
Program-1
#include<stdio.h>
struct date
{
int d,m,y;
};
int main()
{
struct date m1;
m1.d=19;
m1.m=9;
m1.y=2022;
printf("The given date is: ");
printf("%d/%d/%d",m1.d,m1.m,m1.y);
return 0;
}
Explanation:
- Structure declaration begins with the struct keyword.
- Here we declared a Data type “date” using the struct keyword.
- In structure declaration, we have 3 member variables with integer data types.
- In the main function, we declared a variable m1 using the struct keyword, m1 has a “date” data type.
- For accessing the member variables, we use the dot operator.
- We assign some values to our member variables.
- Due to the dot operator, these values are directly assigned to the member variables.
Program Output:
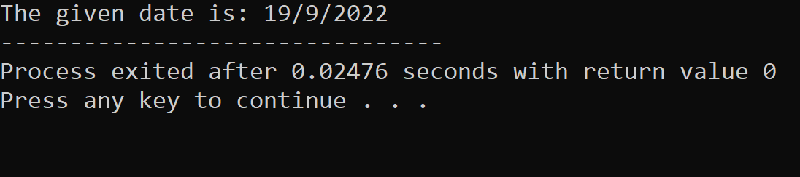
Program-2:
#include <stdio.h>
#include<string.h>
struct worker{
char worker_name[15];
int worker_age;
int worker_salary;
};
int main()
{
struct worker human;
strcpy(human.worker_name, "Swapnil");
human.worker_age = 25;
human.worker_salary =6000;
printf("See your details \n");
printf("Name :%s \n Age :%d \nSalary :%d \n", human.worker_name,human.worker_age,human.worker_salary);
return 0;
}
Explanation:
- Here we created the “worker” data type using the struct keyword.
- In the structure declaration, we have 3 variables.
char worker_name[15];
int worker_age;
int worker_salary;
- In main, we declare a variable “human” using struct keyword, and human has a worker data type.
- human.worker_name is assigned with value“Swapnil”. The function'strcpy()' copies the string referred to by the source to the destination.
- A value “25” is assigned to human.worker_age.
- A value “6000” is assigned to human.worker_salary.
Program Output:
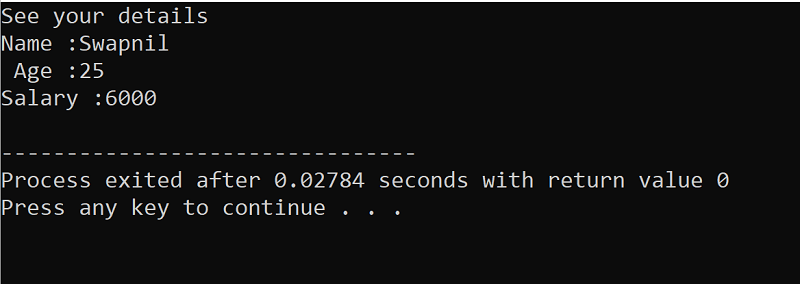