Functions in C
What is function?
Functions are defined as the set of announcements which take inputs, perform operations and provide results. The operation of a function only performs when the function gets called. They accept arguments or values. Due to the presence of function, developers do not need to write the same code again and again. When an argument is passed to a function, the value of every argument is calculated before being passed to that function. Generally, a calling function asked a called function to perform the task when the task is successfully completed the call function reports back to the calling function. Functions are one of the most important terms used in computer science.
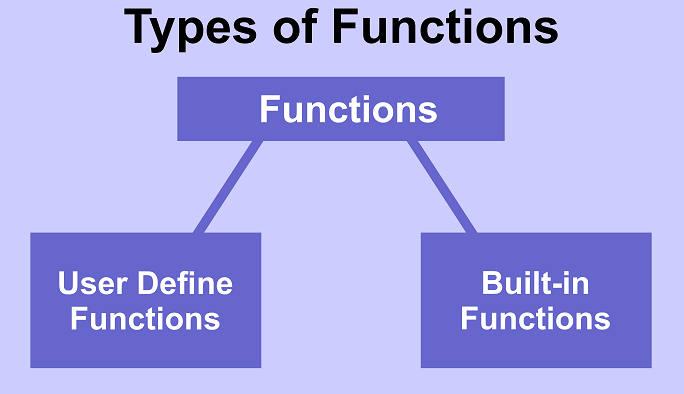
There are two types of functions in C language, that are user defined functions and library functions. Both of these functions are important in C language. User defined functions means which are defined by the users and library functions are those which are built in functions of programming language included in header files.
Creation of Functions
Functions can be defined as follows:
Return_type function_name (parameters)
{
//function’s body
}
- Function_name: As the heading defines, we have to define the function name but it should not be identical. They are known as function_name.
- Arguments: It specifies the values which are passed when the function get called. Arguments plays an important role in defining a functions.
- Return_type: Functions required return type because it always starts with the return type. Void is also used as the return type in functions. Every function required a return type that’s it is one of the most important term of function definition or declaration.
Types of Functions
Functions are divided in two categories. User defined and library functions both functions are one of the most important functions those are used in every programming language whether it is C, C++, Java, Python, C# etc.
- User defined functions: These types of functions are defined or declared by the user itself so that’s why they are known as user defined functions. They are also known as tailor made functions. These functions are updated according to the requirement of the programmer. We all know that this type is not included in the header file of the programming but 90% of the developers require building their own function which is known as user defined function for performing the specific task which is not performed by the library function or a built in function of C language.
- Library functions: These types of functions are also known as built in functions which are included in header files of the particular programming language. Compiler package that already presents contains library functions. They have advantage over user defined functions that there is no need to define the library functions because they are already built. Examples of library functions are pow(), sqrt (), strcmp (), strcpy () etc. Library functions are also as important as user defined functions. These types of function are also used by most of the developers and programmers of C language.
Advantages of User defined Functions
- As we know that they are defined by the user so, they can be redesign according to the requirement of programmer.
- The code of these functions are transformable in other programs.
- They are easy to maintain and recognize the working of these functions.
Advantages of Library Functions
- These are easy to understand and improved for efficient performance.
- These are suitable as they always work.
- It saves time that’s why they are better than user defined functions.
Function with arguments and return values
In the C language which was developed by Dennis Ritchie in the year 1972 which is most advanced type of programming language that time until C++ was discovered in 1983 by Bjarne Strostrup, function is called with the parameters or without the parameters and return values. Sometimes, they may not return values to the calling functions. They are the most important terms of function because most of them whether user defined or library functions.
Difference between Argument and Parameters
Arguments
Arguments are known as the values that are proceed with the function at the time when the functions are called. They are allocated to the variables in the definition of the function which is called. Arguments are one of the most important terms of all time included in the function of C language.
- Arguments are also known as the actual parameters.
- When we call each argument, it is always allocated to the parameter in the function definition.
- During the function, calling the values that are passed are known as arguments.
- They are used to send values from calling function to the destination or receiving function.
Parameters
Parameters are known as local variables. Variables are the type of containers which are used to store the values whether it is an integer, float or character. Which are defined when the function is created. Local variables are used to collect the arguments that are proceed at the time of function call. These type of parameters with the function sample used at the time of function execution. They are also known as formal parameters or we can say formal arguments.
- Parameters are local variables so that’s why getting the allocated value of arguments when the function is called.
- Values which are declared at the time of function sample, known as parameters.
- They are used in function header of called function to collect the values from the arguments. This is the most important difference between the parameter and the argument.