Derived Data Types in C
A variable in a program occupies some space in the computer's memory where some value is stored. Each variable in C has an associated data type. A value to be stored may be an integer, a float, a string, etc.
There are various data types provided by the ANSI C. The Data types are divided into three classes:
- Basic or Primary data types
- Derived data types
- User defined data types
Data Types
Basic Data Types
|
Derived Data Types
|
User Defined Data Types
|
* Each data type mentioned requires a different amount of memory and they vary depending on the type.
Derived Data Type
Derived Data types can be defined as the type of data types that are derived from fundamental data types.
They are derived from the primitive data types by inclusion of some elements of the basic or primary data types. They are used in order to represent single/multiple values.
The derived data types have advanced properties and are used to perform complex tasks in C programming. The derived data types don’t create new data types, they only add some functionality to existing data types.
Types of Derived Data types
The C language supports three fundamental derived data types. They are as follows:
- Arrays
- Pointers
- Functions
Arrays
An array is a collection of items or logically related variables of identical data type which are stored in contiguous memory locations. Once the size of array is declared, it cannot be changed.
The items or elements in the array are stored in contiguous memory locations, i.e., one after another.
Program 1: Printing the elements of an arrays
#include<stdio.h>
int main()
{
int arr[10]= {1,7,10,21,3,9,125,729,800,369} ; //declaring the elements of array
int i; //declaring the local variable
printf("The Elements of array are \n");
for(i=0; i<10 ;i++)
{
printf("%d\n",arr[i]);
}
return 0;
}
Output of the program:
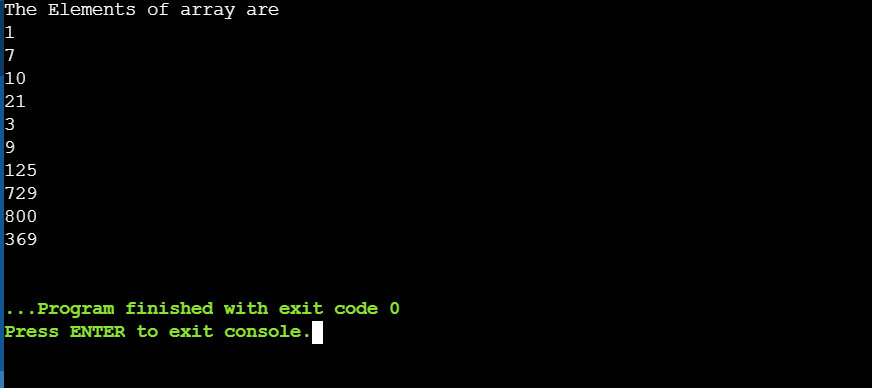
Pointers
A pointer is a variable and this variable contains the address of another variable, i.e., it’s a variable which has the address of another variable as its value. The utilization of pointers is to access the memory and also, to govern the address (manipulation of address).
For eg, If a is a variable of type int having value \0 stored at address 3000 and address of a is assigned to some other variable b, then b is a pointer variable pointing to memory address stored in it. In simple words, b is a pointer variable containing the address of a (i.e., 3000).
Program 2: Retrieving the value stored and address of variable using pointers
#include <stdio.h>
#include <conio.h>
int main ()
{
int count = 5; //declaring the local variables
int *ptr = &count; //pointer variable
printf("The value of count variable is %d\n", count);
printf("Address of count variable: %x\n", ptr);
printf("Value retrieved through pointer : %d\n", *ptr);
getch();
return 0;
}
Output of the program:
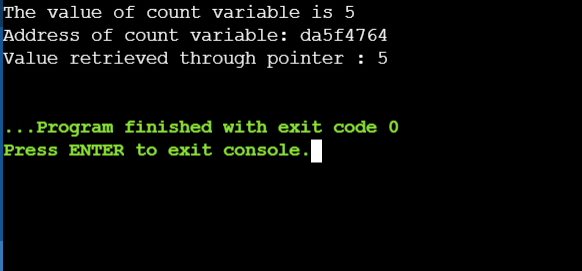
Functions
A function is a self-contained block of statements that performs a specific task. A function wraps a number of statements which are repeated in a program into a single unit or block. This wrapping of statements(unit) is identified by a name known as a function name.
Every C program consists of at least one function, which is the main() function.
Program 3: Multiplication of two numbers using functions
#include<stdio.h>
int multiplication(); // function declaration
int main()
{
int output; //local variable definition
output = multiplication();
printf("The product of the two numbers is: %d\n",output);
return 0;
}
int multiplication()
{
int num1 = 5, num2 = 15;
return num1*num2;
}
Output of the program:
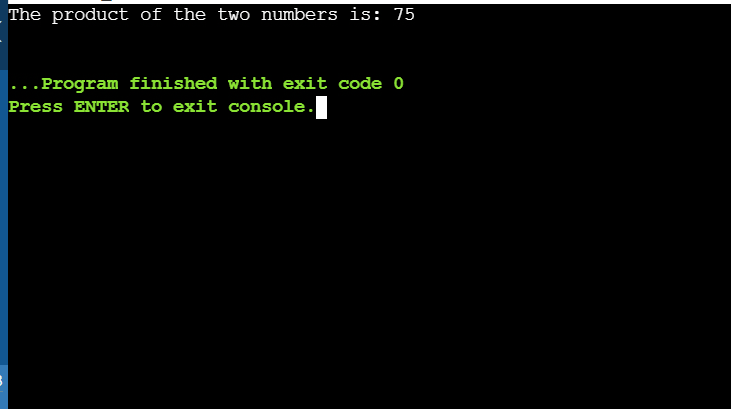
Program 4: Calculator program in C using functions
#include <stdio.h>
float addition(float x, float y);
float subtraction(float x, float y);
float multiplication(float x, float y);
float division(float x, float y);
int main()
{
char operator;
float num1, num2, result = 0;
printf("\n Please Enter a valid Operator (+, -, *, /) : ");
scanf("%c", &operator);
printf("\n Enter the values for operands: num1 and num2 : ");
scanf("%f%f", &num1, &num2);
switch(operator)
{
case '+':
printf("\n Operator entered : Addition");
result = addition(num1, num2);
break;
case '-':
printf("\n Operator entered : Subtraction");
result = subtraction(num1, num2);
break;
case '*':
printf("\n Operator entered : Multiplication");
result = multiplication(num1, num2);
break;
case '/':
printf("\n Operator entered : Division");
result = division(num1, num2);
break;
default:
printf("\n You have entered an Invalid Operator "); }
printf("\n The result of %.2f %c %.2f = %.2f", num1, operator, num2, result);
return 0;
}
float addition(float x, float y)
{
return x + y;
}
float subtraction(float x, float y)
{
return x - y;
}
float multiplication(float x, float y)
{
return x * y;
}
float division(float x, float y)
{
return x / y;
}
Output of the program:
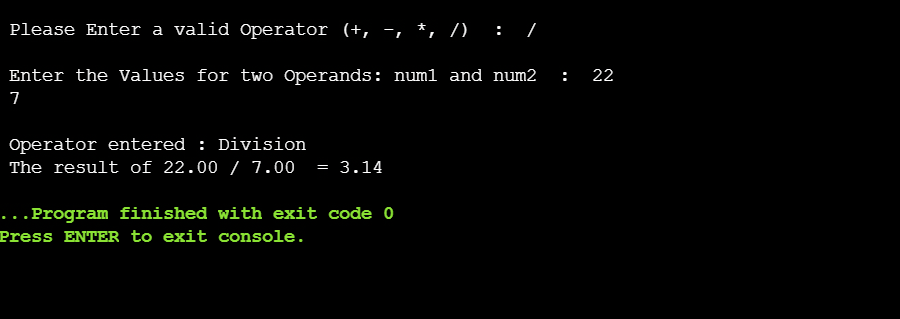