Evaluation of Arithmetic Expression in C
We can use the "eval" function in C to evaluate an arithmetic expression stored as a string. However, using "eval" is generally considered bad practice and can lead to security vulnerabilities if the input is not properly sanitized.
A better approach would be to use a shunting yard algorithm or write a recursive descent parser that can evaluate the arithmetic expression.
Another option could be to use the C math library, with functions like sin, cos, exp, etc., to evaluate trigonometric and exponential expressions.
We can also use the C++ math library 'cmath' to accomplish the same task with more functionalities.
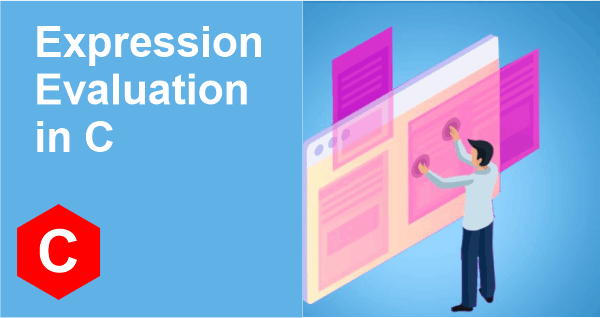
Evaluating arithmetic expressions in C is a common task in computer programming. However, the method of evaluation can vary depending on the complexity of the expression and the desired level of security. In this article, we will discuss different methods for evaluating arithmetic expressions in C, including the use of the "eval" function, the shunting yard algorithm, and the recursive descent parser.
The "eval" function is a built-in function in C that evaluates a string as an arithmetic expression. The function takes the expression as a string and returns the result as a double. The syntax for using the "eval" function is as follows:
double result = eval(expression);
While the "eval" function is a convenient method for evaluating arithmetic expressions, it is generally considered bad practice due to security vulnerabilities. The input to the function is not sanitized, which means that a malicious user could potentially enter an expression that would cause a buffer overflow or other security vulnerability.
An alternative method for evaluating arithmetic expressions in C is the shunting yard algorithm. The algorithm was developed by Edsger Dijkstra and is used to convert an infix arithmetic expression to postfix notation. The postfix notation is then used to evaluate the expression. The shunting yard algorithm is a more complex method than the "eval" function, but it is more secure and can handle more complex expressions.
Another alternative method for evaluating arithmetic expressions in C is the recursive descent parser. This method involves writing a program that parses the expression and evaluates it. The program uses a set of rules to determine the order of operations and the precedence of operators. The recursive descent parser is a more complex method than the "eval" function and the shunting yard algorithm, but it is also more secure and can handle more complex expressions.
The C math library also provides a number of mathematical functions that can be used to evaluate trigonometric and exponential expressions. These functions include sin, cos, exp, and log. These functions can be used to evaluate expressions that involve trigonometric and exponential functions, but they cannot be used to evaluate more complex expressions.
C++ also provides a math library 'cmath' that can be used to evaluate arithmetic expressions. The library provides mathematical functions such as sin, cos, exp, log, etc. as well as additional functionalities like hyperbolic trigonometric functions and complex numbers. This library can be used to evaluate more complex expressions.
In conclusion, evaluating arithmetic expressions in C is a common task in computer programming. While the "eval" function is a convenient method for evaluating expressions, it is generally considered bad practice due to security vulnerabilities. Alternatives such as the shunting yard algorithm, the recursive descent parser, and the C/C++ math library can be used to evaluate more complex expressions and provide a higher level of security. As a developer, it is important to choose the appropriate method for evaluating arithmetic expressions based on the complexity of the expression and the desired level of security.
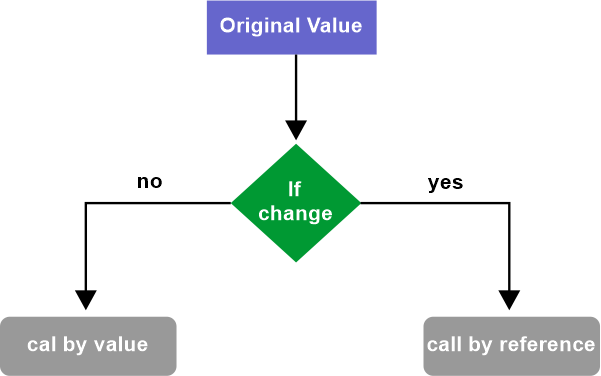
Types of Arithmetic Expression Evaluation
There are several types of arithmetic expression evaluation, including:
- Infix notation: Expressions are written in the form of "operand operator operand", for example, 2 + 3. This is the most common notation used in mathematics and programming.
- Postfix notation (Reverse Polish notation): Expressions are written in the form of "operand operator", for example, 2 3 +. This notation is used in stack-based computing and is considered to be more efficient than infix notation.
- Prefix notation (Polish notation): Expressions are written in the form of "operator operand operand" for, for example: + 2 3. This notation is used in some programming languages and is considered to be more efficient than infix notation.
- Functional notation: Expressions are written in the form of functions, for example, f(x) = 2x + 3. This notation is used in mathematics and computer science.
- Reverse-Polish functional notation: Expressions are written in the form of functions with postfix notation, for example, x 2 * 3 +
- Reverse-Polish Notation with Functional notation: Expressions are written in the form of functions with postfix notation, for example, sin(x) + cos(x)
Each type of notation has its own set of rules and algorithms for evaluating the expression, and it is important to choose the appropriate method based on the complexity of the expression and the desired level of security.