How to delete a file in C
A file is a group of data kept on a secondary device, such as a hard disc. It is typically utilized as a real-world application with a lot of data.
These applications have two following problems:
- Handling such a massive volume of data requires a lot of time and effort.
- The entire data is lost if the software is closed or the computer is turned off when the I/O terminal is in use. Consequently, it is essential to save the data on a durable medium.
There are two categories of files
- Stream-oriented - They are either high-level or standard files. Compared to system-oriented data files, they are more common and simpler to utilize.
- System-oriented - These files are of a low level.
The library functions to manage the files are given below:
- High-level file I/O functions - They have command over their buffers.
- Low-level file I/O functions - Programmers are responsible for managing buffers.
File operations in C Language
- Opening a file (fopen())
- Closing a file (fclose())
- Reading a file (fscanf())
- Writing a file (fputs())
Opening a file: Create a file pointer first before a user can access any file.
Syntax:
FILE *ptr;
Closing a file: A file is closed using the fclose() method. The file pointer is separated from a file when this function is utilized.
Syntax:
int fclose(FILE *fp);
Reading a file: Using the method fopen(), open a file and save its reference in a FILE pointer. Use one of these functions, fgetc(), fgets(), fscanf(), or fread(), to read the file's contents (). Close the file using the function fclose ().
There are numerous techniques to read a text file. Several of them are.
- Completely read a file's contents.
- Read every word in a text file.
- Read each character in the text file.
Writing a file: Using fprintf() and fscanf, we can read from and write to text files (). They are all file versions of the printf() and scanf() functions. The main distinction is that fscanf() and fprintf() require a pointer to the FILE structure.
Syntax:
int print(FILE *stream, const char *format);
To add a line to a file, use the fputs() function.
Syntax:
Int fputs(const char *str,FILE *stream);
To the stream pointed to by stream, the fputs() function writes the string pointed to by str. It returns 0 upon successful completion and end of file otherwise.
Delete File by using C program
Use the remove () method of stdio. h to remove a file in the C programming language. The remove() function deletes a file by taking a file name (or path if it is not in the same directory) as an input. Remove () returns 0 if the file is successfully deleted. Else it produces a non-zero value. We'll delete a file using stdio.h's remove() function.
What is remove() in C?
Remove is a C library function (). The C library's int remove(const char *filename) function deletes and renders unavailable the provided filename.
Syntax:
remove (“file_name”);
What is the remove function?
The REMOVE() function returns a fixed-length string after removing unnecessary characters from character data.
Delete a file using the C language
This C application deletes the user-specified file. The file to be removed must exist in the directory where this program's executable file is located.
The file is deleted using a remove macro, and the user must additionally specify the file extension. If a problem removes the file, a message will be printed using the error function.
/* This C program shows how to delete user-specified files from the directory */
#include<stdio.h>
#include<conio.h>
int main()
{
int status;
char file_name[25]; //declaration of file of character type
printf("Enter the name of file you wish to delete\n");
gets(file_name); //File input
status = remove(file_name); //deletion or removal of file
if( status == 0 ){
printf("%s file deleted successfully.\n",file_name);
}
else
{
printf("Unable to delete the file\n");
perror("Error");
}
return 0;
}//main block ends
Output
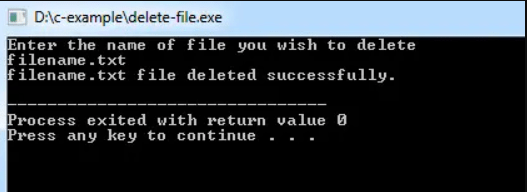
The deleted file does not go to the trash or recycle bin, so the user might not be able to get it back. Delete files can be restored using specialized recovery software if they aren't overwritten on the storage medium.