Nested loop in C
Definition of Nested Loop
A nested loop is generally used when we want to run a loop statement inside another loop statement. This kind of loop is also known as a “loop inside the loop”.
Diagrammatical presentation of Nested loop Code with the help of flow chart
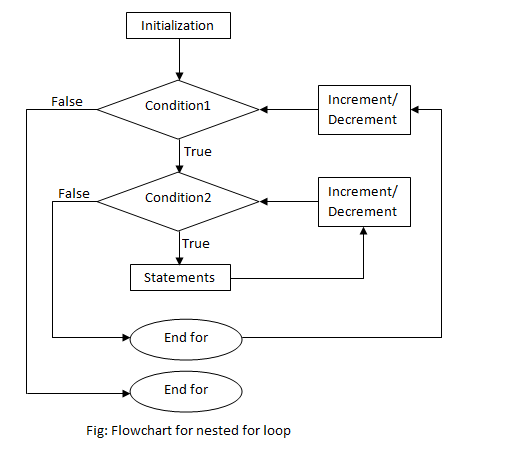
Explanation of the above Flow chart:
- As you can see, firstly, we provide an initialization statement.
- Then, the control evaluates the value of the first condition and checks whether the termination condition is true or false.
- If the value is false, the control will terminate the entire loop and only perform the basic command. But, if the value is true, it will continue to check the second condition.
- If it finds that the second condition is false, it will terminate the code of the second condition, and the control will move to the primary command and perform the loop. And if the value of the second condition is true, it will execute the code and check the termination condition, and it will continue to do so until the value becomes false.
- Along with this, some increment or decrement statements are also implemented after every statement execution.
- When the second condition terminates, the control goes back to the first condition, checks again, and repeats the entire execution process.
- The control will check and execute the termination condition multiple times.
- And it performs the condition given at the end and produces the output for displaying on the screen.
Now, let’s see the syntax of the nested loop in all loops of C.
Syntax Used For Initiating “Nested For Loop”:
for (initialization; termination condition; increment/decrement statement) {
// program line of the first loop
}
// program line of the second loop
}
Syntax Used for Initiating “Nested While Loop”:
while(condition)
{
// condition must be provided
while(condition)
{
// program line of the first loop
}
// program line of the second loop
}
Syntax Used for Initiating “Nested Do-While Loop”:
do
{
do
{
// program line of the first loop
}
while(condition); //condition is given here
// program line of the second loop
}
while(condition);
Syntax Used for Initiating “Nested Mixed (Do-While, While, For) Loop”:
do
{ while(condition) // condition should be provided
{
for (initialization; termination condition; increment/decrement condition)
{ // program line of the first loop
}
// program line of the first while loop
}
// program line of second do-while loop
}
while(condition);
Example 01: Program of Nested for loop:
#include <stdio.h>
#include <stdlib.h> /* it involves memory
allocation, process control, conversions */
#define ROW 3 // rows declaration
#define COL 3 // columns declaration
// Driver program code
int main ()
{
int i, j;
// Declaration of the matrix
int matrix [ROW][COL] =
{
{11, 12, 13},
{14, 15, 16},
{17, 18, 19}
};
printf("the provided matrix is \n");
// loops implementation
for (i = 0; i < ROW; i++) // increment statement
{
for (j = 0; j < COL; j++) // increment statement
printf("%d ", matrix[i][j]);
printf("\n");
}
return 0;
}
Output:
the provided matrix is
11 12 13
14 15 16
17 18 19
Example 02: Program of nested while with for loop:
/* program in c to find out every prime factor
of a specific number with the help of nested loop */
#include <math.h> // library to include mathematical operations
#include <stdio.h>
// program line to display every possible prime factor of a provided number n
void prime factors (int n)
{
// divisibility check
while (n % 2 == 0)
{
printf("%d ", 2);
n = n / 2;
}
/* n must be odd So that one element can be we can be skipped
(Note: i = i +2) */
for (int i = 3; i <= sqrt(n); i = i + 2)
{
// when n is divisible by i then display i and divide n
while (n % i == 0) {
printf("%d ", i);
n = n / i;
}
}
/* This test expression is defined to deal with the cases like
n is a prime number greater than 2 */
if (n > 2)
printf("%d ", n);
}
int main ()
{
int n = 1578;
primeFactors(n);
return 0;
}
Output:
2 3 263