How to move a text in C
A text can be moved to the desired location in the output based on the coordinates provided in the gotoxy() function.
What is gotoxy() function?
In C language, gotoxy() is a pre-defined function that helps in moving a text by placing the cursor at any desired location on the output screen. Using the gotoxy() function, one can quickly move or change the cursor position anywhere. The cursor position is also known as the cursor location. The gotoxy() function prints the required text wherever the cursor moves. The cursor is driven based on the x and y coordinates mentioned in the gotoxy() function.
Syntax
gotoxy(int x, int y);
Here (x,y) is where the user wants to print the text on the output screen.
C program to print the “king” message on the output screen without using the gotoxy() function :
// C program without using gotoxy() function
#include <stdio.h>
void main()
{
printf("king"); //printing the text “king”
}
Output:
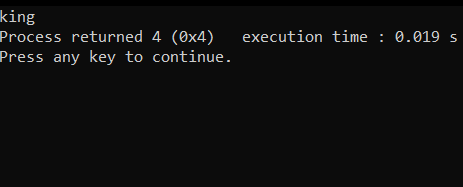
This is the output for printing the text "king" without using the gotoxy() function.
The text "king" is displayed on the coordinates (0,0) of the output screen. It shows the text on the top left side, which is at coordinates (0,0) by default. The top left side is the default position for a text to be displayed.
gotoxy() in Code::Blocks
In Code::Blocks, the gotoxy() pre-defined function is not provided. Hence, a function “SetConsoleCursorPosition()” is used to replace the gotoxy() function. SetConsoleCursorPosition() is used for the same procedure as gotoxy().
A header file named “#include<windows.h>” is added to use the SetConsoleCursorPosition() function.
The function SetConsoleCursorPosition() has some arguments such as :
- Handle
- COORD
Handle: The handle is used to get the values. A pre-defined function "GetStdHandle(STD_OUTPUT_HANDLE)” is called.
COORD: The X and Y coordinates are available by using the pre-defined function COORD.
Note: A screen usually has 80 columns and 25 lines.
C program to print the “king” message on the output screen using the SetConsoleCursorPosition() function instead of using gotoxy() function:
#include <stdio.h>
#include <windows.h>
void main()
{
COORD c;
c.X = 50; //x coordinate as 50
c.Y = 12; // y coordinate as 12
// using the SetConsoleCursorPosition() function
SetConsoleCursorPosition(
GetStdHandle(STD_OUTPUT_HANDLE), c);
printf("king"); //printing the text “king”
getch();
}
Output:
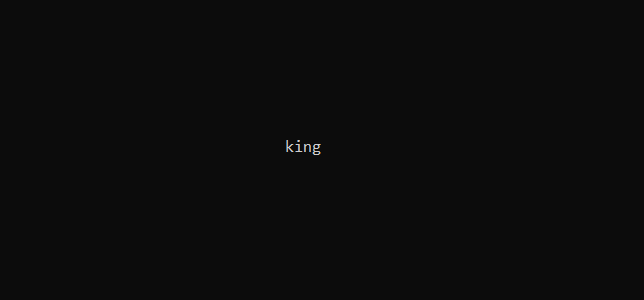
This is how the output looks at the position x=50 and y=12 using the COORD. The text “king” can be displayed anywhere on the output screen by changing the coordinates x and y, where x is the horizontal axis, and y is the vertical axis.
C program to print the “king” message on the output screen using the gotoxy() function by defining it :
#include<windows.h>
void gotoxy(int x,int y)
{
COORD c;
c.X=x;
c.Y=y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE),c);
}
main()
{
int x=50,y=12;
char ch;
gotoxy(x,y); //function call
printf("king");
while(1){
ch=getch();
switch(ch)
{
case 'a':
x--; //horizontal decrement in position
break;
case 'd':
x++; //horizontal increment in position
break;
case 'w':
y--; //vertical increment in position
break;
case 's':
y++; //vertical decrement in position
break;
case 'p':
exit(0);
}
system("cls"); //acts like a clrscr() in turbo c/c++
gotoxy(x,y);
printf("king");
}
}
Output 1:
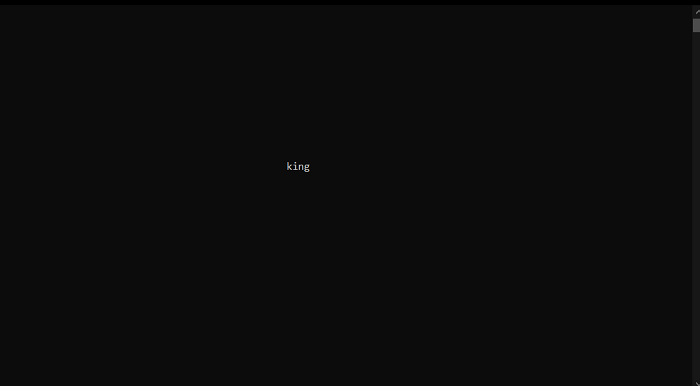
This is the output displayed without using any keys.
Output 2:
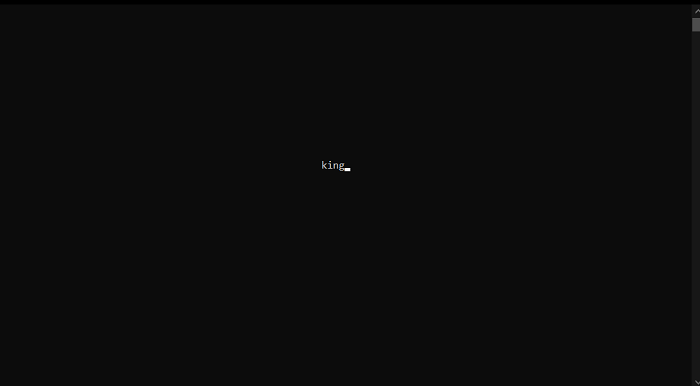
This is the output displayed when the key 'd' is used five times. This moves the text 5 coordinates to the right from x=50. “_” is the cursor whose position can be changed by pressing keys.
Note: COORD holds the coordinates x and y. The COORD is named after coordinates. The GetStdHandle function returns a handle as input, output, or error. Windows kernel object can be accessed to a handle that is an index in a system table. SetControlCursorPosition() function sets the cursor location.
In the gotoxy() function, the x and y coordinates are row and column numbers.
In Turbo C/C++, the gotoxy() function is available. This function moves a text on the output screen using the coordinates ( parameters passed to the function). The top compilers, GCC and Visual Studio, do not have a gotoxy() function. The text alignment can be maintained using the gotoxy() function.
Program using gotoxy() function (turbo c/c++):
The following program is compatible only with the turbo c/c++ compiler.
#include<stdio.h>
#include<conio.h>
int main()
{
int x, y;
x = 10;
y = 10;
gotoxy(x, y);
printf("king”); // printing the text “king”
getch();
return 0;
}
Output:
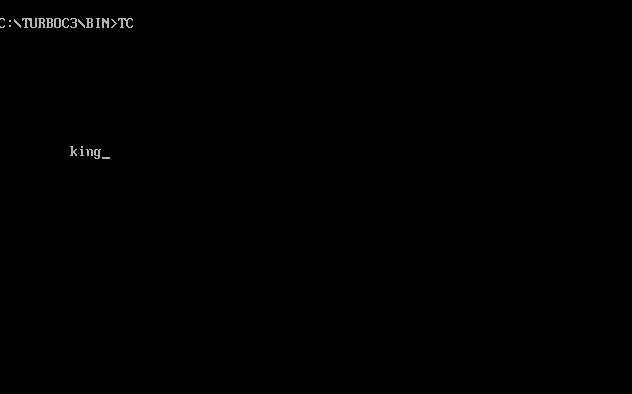