How to return a Pointer from a Function in C?
In the C programming language, pointers are variables that hold the address memory location of another variable. We could also input pointers to a function and return pointers from a function. However, returning the location of the local variable outside of the function is not recommended since it will be out of scope once the method returns.
Syntax for a function declaration that returns a pointer:
returnType *func_name(parameter);
Where, “returnType” denotes the type of pointer returned by the function “func_name” and here "parameter" is a list of the function's optional arguments.
For example, We declare a function called "Max_numbers" that accepts two integer pointer variables as parameters and returns an integer pointer.
int *Max_numbers(int * , int *);
Example – 1:
For example, the Max numbers( ) function returns an integer pointer, which is the location of a variable with the bigger value.
#include <stdio.h>
/* Function Declaration */
int *Max_numbers(int *, int *);
int main(void){
/* Define integer data-type variables */
int a = 180;
int b = 360;
/* Define pointer variable*/
int *maxi = NULL;
/* Get the variable address with the highest value by supplying the addresses of a and b to the function Max_functions( ) */
maxi = Max_numbers(&a, &b);
/* print the greater value using printf function */
printf(“Maximum Value:%d\n”,*maxi);
return 0;
}
/* Define Function */
int *Max_numbers(int *x, int *y) {
/* Return the address contained in the pointer variable ‘a’ if the value given by pointer ‘x’ is bigger than ‘y’ */
if (*x > *y){
return x;
}
/* else return the address stored in the pointer variable ‘y’ */
else {
return y;
}
}
Output:
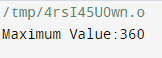
We can represent these two variables ‘a’ and ‘b’ in the memory location as follows
int x = 180;
Assume the address location of the variable ‘a’ which has value 180 is 6550.
int y = 360;
Assume the address location of the variable ‘b’ which has value 360 is 7550.
Then we make an integer pointer variable “maxi” that has the address of the variable with the higher value. At this time, the pointer variable “maxi” is stored at address location 8550 with the value NULL.
Following that, we use the Max_numbers() method, supplying the addresses of variables ‘a’ and ‘b.’ In the Max_numbers() function, parameter ‘x’ receives the address of variable ‘a’ and parameter ‘y’ gets the address of variable ‘b.’
As a result, integer pointer variable x retains the address of variable a, i.e., 6550, and is assigned memory location 10850 (assume).
Similarly, the integer pointer variable y is assigned memory position 10852 and stores the address of variable b, which is 7550.
Now we're comparing to see whether *x > *y, which means that the value indicated by the pointer variable x is bigger than the value indicated by the pointer variable y.
The value shown by the pointer variable x is 180, while the value indicated by the pointer variable y is 360. As a result, the if block is disregarded, and the else block is run, returning the address held in the pointer variable y, 7550, which is the address of the variable b.
As a result, the address of variable b is returned by the Max_numbers( ) method and placed in the integer pointer variable maxi. So, the maxi variable now has the address 7550.
Finally, we display the value saved at position 7550 using the pointer variable maxi. This gives us the bigger value, i.e., 360.
Example -2:
#include <stdio.h>
/* Function returning the pointer variable */
int *function( ) {
int K = 100;
return (&K);
}
/* Main block code */
int main( ) {
/* Pointer Declaration */
int *q;
/* Calling the function */
q = function( );
printf("%p\n", q);
printf("%d\n", *q);
return 0;
}
Output:
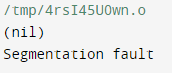
Because "K" was local to the function, the above program code will generate a segmentation fault.
The fundamental cause of this situation is that the compiler always creates a "stack" when calling a function. When a function quits, the function stack is likewise erased, and the local variables of the function are no longer in scope.
Static Variables
Static variables have the property of holding their value even when they are removed from their scope. To implement the idea of returning a pointer from a function in the C language, the local variable must be declared as a static variable.