Armstrong program in C using function
In this article we will learn how to find Armstrong of a number using function in C.
An Armstrong number is a three-digit number that is the sum of its separate digits' cubes.
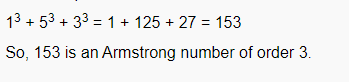
Pseudo Code:-
Take the input as number
last_dig = 0
WHILE number != 0
last_dig ← number % 10
pow ← last_dig * last_dig * last_dig
sum ← sum +pow
divide / 10
END WHILE
IF sum == number
PRINTF armstrong
ELSE
PRINTF not an armstrong
END IF
end procedure
Code: -
#include<stdio.h>
int CheckArmstrong(int num)
{
int last_dig = 0;
int pow = 0;
int sum = 0;
int n = num;
while(n!=0)
{
last_dig = n % 10;
power = last_dig *last_dig * last_dig;
sum =sum+ pow;
n /= 10;
}
if(sum == num)
return 0;
else return 1;
}
int main()
{
int num;
printf("Enter number: ");
scanf("%d",&number);
if(CheckArmstrong (num) == 0)
printf("%d is an Armstrong number.\n", num);
else
printf("%d is not an Armstrong number.", num);
return 0;
}
Output: -
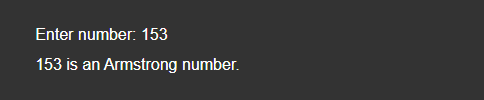
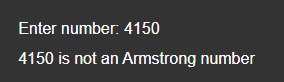
Explanation:-
- First we have the integer variables ,
- Then assign the value to the respective variables.
- After that we have to split all the digits of number of which we want to find the armstrong.
- Then find the cube of each digit of number.
- After that store the output to a variable like named sum.
- Then check if sum is equal to the function created equals to zero then print it is armstrong number
If the sum is not equal to the function then print that it is not armstrong number.