How to measure time taken by a function in C?
Measuring the time taken by a function is quite a complex task, because numerous methods are frequently not transferable to other platforms, measuring the execution time of a C programs or of certain parts of it can sometimes be more difficult than it should be. The best way to use will mostly depends on your operating system, compiler version, and what you mean by "time."
Two important things to be noted while measuring the time are:
Simply said, wall time - also referred to as clock time or wall-clock time - is the entire amount of time that passed during the measurement. Given that you can start and stop the stopwatch accurately at the desired execution moments, it is the amount of time you can measure with one.
Inversely, CPU Time describes the period of time when the CPU was working to process the program's instructions. The CPU time does not account for the time spent waiting for other processes, such as I/O activities, to finish.
Importance of measuring time: If you’re going to evaluate performance you have to measure something, you can tell if you have improved. If a program can do the same thing in less execution time, then it can be considered more efficient, doing the same with less resources.
In simple words, measuring time of a function or a program is a measure of how long an algorithm takes to run in relation to the size of the input.
It calculates how long it takes for each algorithm's code statement to run. In other words, it is efficient, or how long a program function takes to process a given input.
Therefore, it’s highly important to know measuring time of a function or a program which ultimately results in improving the efficiency of thast particular function or program.
There are many methods available in calculating the time taken by a function. One of the methods is to use clock() function.
Clock() function is available in time.h header file.
Syntax:
clock_t begin = clock();
/* here, do your time-consuming job */
clock_t end = clock();
double time_spent = (double)(end - begin) / CLOCKS_PER_SEC;
Let’s see some programs to understand better:
Example 1:
#include <stdio.h>
#include <time.h>
int main(){
clock_t start = clock();
clock_t stop = clock();
double elapsed = (double)(stop - start) * 1000.0 / CLOCKS_PER_SEC;
printf("Time elapsed in ms: %f", elapsed);
}
Output:

The output won’t be constant and charges from time to time.
Example 2:
#include <stdio.h>
#include <time.h> // for clock_t, clock(), CLOCKS_PER_SEC
#include <unistd.h> // for sleep()
int main()
{
double time_spent = 0.0;
clock_t begin = clock();
sleep(3);
clock_t end = clock();
time_spent += (double)(end - begin) / CLOCKS_PER_SEC;
printf("The elapsed time is %f seconds", time_spent);
return 0;
}
Output:

The sleep() function enables users to wait for a running thread for a specified amount of time. While the sleep() function will put the current executable to sleep for the amount of time specified by the thread, other CPU operations will continue to run smoothly.
Example 3:
#include <stdio.h>
#include <time.h>
void fun()
{
printf("fun() starts \n");
printf("Press enter any key to stop function \n");
while(1)
{
if (getchar())
break;
}
printf("fun() ends \n");
}
int main()
{
clock_t ti;
ti = clock();
fun();
ti = clock() - ti;
double time = ((double)ti)/CLOCKS_PER_SEC;
printf("fun() took %f seconds to execute \n", time);
return 0;
}
Output:
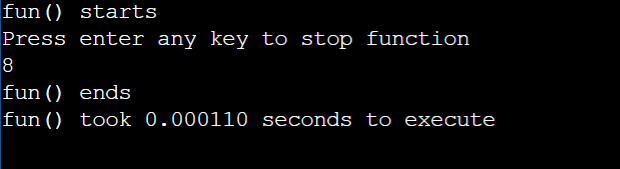
Also, it’s important to know various types of times which have to be considered, while measuring the time if a program/function.
Computer science words such as "compile," "load," and "execution times" all refer to the various phases of running software programs.
Build Time
The process of converting computer programs and code into a form that the CPU can understand, or machine-readable code, is known as compile-time. A compiler usually accomplishes this. Code in the source language is converted to a particular target language at build time.
Syntax and semantic analysis are a couple common tasks carried out at compile time. The assignment of specific computer program instructions to certain physical memory locations is another feature of compile time.
Load Period
The term "load time" describes the stage at which all the programs are loaded into memory with the aid of a loader and is typically followed by.
The software is also ready for the execution phase at load time. Reading the program's instructions and making sure any resources required for execution are ready are a few tasks carried out during this period.
Process Time
It’s a specific stage at which the commands in computer programs or code are carried out is referred to as execution time. Run-time libraries are utilized during execution. Reading programs instructions to perform tasks or complete activities is one of the fundamental operations that take place at execution time.
How measuring time of a function or a program helps in real life:
To measure efficiency of a computer program:
Dozens of companies have come up with “metrics” to measure performance - and none of them truly encapsulate the “value” of a programmer to the company.
For example:
You could count the number of lines of code they write per month. But terrible programmers need more lines of code to do the job that a good programmer can code more succinctly - more efficiently.
You could try to break up a project into “difficulty points” and count the number of “difficulty points” each programmer deals with per month. But it’s notoriously hard to align the actual difficulty of a problem from one team to another - so you really don’t get any kind of absolute count that works.
Counting the number of bugs fixed is an interesting approach - but it’s hard to cope with an occasional bug that turns out to be horribly hard to fix compared to some that turn out to be unexpectedly trivial.
In the end, what companies that I’ve worked for do is to combine three documents in an “annual review” process:
1. The lead engineer’s report on the programmer.
2. A “peer review” written by a co-worker who is picked at random.
3. A “self-review” where the programmer writes their own review.
Each person lists the big successes and the big difficulties for the person being reviewed.
The team manager collects those three reports - tries to generate a coherent picture from it - which turns into a combined review. The programmer and the manager read through it together - and perhaps tweak the wording and sign the resulting document as a “true record” of the year. These are used to decide for each person whether they performed better than expected, worse than expected or about right.
Those who met expectations get an inflation-linked and experience-based pay rise.
Conclusion:
Therefore, measuring the time of any program or a function gives us an idea of word time, execution time, process time etc. It really makes a huge difference understanding the concepts of those time. Time is one of the most valuable resources known to humans. Its limited availability is what makes it precious. Time management is crucial because it enables you to accomplish more in less time hence increasing your efficiency.