Socket Programming in C
Socket programming is a process that connects the two or more nodes on a networking communication with respective to each other. One of the sockets that determines the socket (I.e., node) that listens on a specific port in an IP address, then coming to another socket it reaches the out to other form of the connection. The socket programming server connects the several forms to the listener socket and the client consumes out of the server networking.
- socket()
- bind()
- listen()
- accept ()
For client process there are two steps involved in it.
- socket()
- connect()
There is an inter connection link between those server and client process.
- ) method connects with the bind() method with an error. From the blind() it enters into the listen() by accept() method that will not block. Again, it will process into the accept() method by accepting the block until it reaches to the connect().
- Then it will enter into the read() and write() method to folllow up the socket facilitate communication process. After that it closes the close (). This indicates the successful completion of server and client model process of the socket programming.
The stages for the server process.
1. Socket creation:
The syntax for the socket creation is:
int sockfd = socket(domain, type (communication type), protocol(IP)
- sockfd: it is a socket descriptor like an integer value(I.e. file handle function)
- Domain: it is an integer that specifies the communication domain. The AF_LOCAL that defined as the POSIX standard that communicates between the process for the same host of the server process. For the communication purpose we have the different hosts that connected by the IPV4 for the AF_INET and we use IPV6 for the AF_INET 6.
- type: it is one of the communication types. There are two types in the process:
- SOCK_STREAM
- SOCK_DGRAM
- Protocol: The protocol is the value function that determines the internet protocol address (IP) which rates 0. This is the constant value that appears on the above protocol field.
2. Setsockopt: This is the filed that helps in manipulating the options which may referred by the socket in the file descriptor in the sockfd. This thing is an optional one not a mandatory, but this can helpful in reuse the address and port. It depicts an error for the “address that already in use”.
The syntax for setsockopt is defined as:
int setsockopt(int sockfd(socket descriptor), int level, int optname(opted name), const void *optval(opted value), socklen_taddresslen)
3. Bind:
The syntax creation for Bind:
int bind(int sockfd, const struct sockaddress: address, socklen_taddresslen);
After successful creation of the socket, the bind function that binds the socket for the address and the port number of the IP that specified in the address (I.e. the custom data structure of the port).
4. Listen:
The syntax for the listen:
int listen(int sockfd, int backlog);
5. Accept:
The syntax creation for Accept:
int new_socket = accept(int sockfd, struct sockaddress *address, socklen_t *addresslen)
In this step it extracts all the possible first connections on the request of the queue of the connections that are in the pending state for listening of the socket, sockfd. It creates the new socket connection and it returns to the new file of sockfd. In this point it is established a connection between the server and the client.
Stages for the client process:
- Socket connection: It is same as the server process for the socker creation.
- Connect: The connect() is a function call that connects the server is referred to the sockfd( file descriptor) which is specified by the address.
Syntax for creating the connect():
int connect(int sockfd. Const struct sockfd *address, socklen_taddresslen);
Let's see an example program for server socket creation:
Example 1:
// socket programming
#include <netinet/in.h> // preprocessor for socket programming
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h> // preprocessor for socket programming
#include <unistd.h>
#define PORT 1000
int main(int args, char const* argc[]) // main function
{
int server_fd ,new_socket, valueread; // for descriptor
struct sockaddress_inaddress; // structure of address
int opt = 10;
int addresslen = sizeof(address); // defining size and length
char buffer[1024] = { 0 }; // assigning buffer
char* Hi = "Hi greeting from server";
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0))// socket descriptor
== 0) {
perror("socket failed"); // error for socket failure
exit(EXIT_FAILURE); // exit the failure of the socket
}
if (setsockopt(server_fd, SOL_SOCKET, // forcing to attach
SO_REUSEADDR | SO_REUSEPORT, &opt,
sizeof(opt))) // reusing the address
{
perror("setsockopt"); // error for setsockopt
exit(EXIT_FAILURE); // exiting failure
}
address.sin_family = AF_INET; // importing the address
address.sin_address.s_addr = INADDR_ANY;
address.sin_port = htons(PORT);
if (bind(server_fd, (struct sockaddr*)&address, // forcing for the socket
sizeof(address))
< 0) {
perror("bind failed"); // failure for bind
exit(EXIT_FAILURE); // exiting failure
}
if (listen(server_fd, 3) < 0)
{
perror("listen failure"); // listen error
exit(EXIT_FAILURE); // exiting failure for listen
}
if ((new_socket
= accept(server_fd, (struct sockaddr*)&address,
(socklen_t*)&addresslen)) // creating new socket for accept server process
< 0) {
perror("accept"); // accept error for server process
exit(EXIT_FAILURE); // exiting the failure
}
valread = read(new_socket, buffer, 1024); // socket for buffer
printf("%s\n", buffer);
send(new_socket, hello, strlen(hello), 0); // sending socket
printf("Hi message is sent\n");
close(new_socket); // close the connected socket
shutdown(server_fd, SHUT_RDWR); // closing listened socket
return 0;
}
The output will conflict after the client process.
Let’s see an example program for client server process
Example 2:
// socket programming for client process
#include <arpa/inet.h> // preprocessor for client process
#include <stdio.h>
#include <string.h>
#include <sys/socket.h> // preprocessor for socket programming
#include <unistd.h>
#define PORT 1020
int main(int args, char const* argc[]) // main function for client
{
int sock = 10, valread, client_fd; //client depositor for value read
struct sockaddress_inserv_address; // server address for structure
char* hello = "Hi message from the client process";
char buffer[1024] = { 0}; // buffer for IP address
if ((sock = socket(AF_INET, SOCK_STREAM, 0)) < 0) // socket stream
{
printf("\n The Socket creation error in client \n"); // socket error creation for client process
return -1; // returning the value
}
serv_address.sin_family = AF_INET; // server address for creation
serv_address.sin_port = htons(PORT); // address port
if (inet_pton(AF_INET, "171.0.0.1", &serv_address.sin_address)
<= 0) // converting IPv4 and IPv6 addresses to the client server
{
printf(
"\n The Address is not supported for creation \n");
return -1; // returning the value
}
if ((client_fdc= connect(sock, (struct sockaddress*)&serv_address,
sizeof(serv_address)))
< 0) // connecting the server address to the IP
{
printf("\nThe Connection is Failed \n");
return -1;
}
send(sock, hi, strlen(hi), 0); // sending the server
printf("Hi message is sent\n");
valread = read(sock, buffer, 1024); // reading the sock
printf("%s\n", buffer);
close(client_fd); // closing the connection
return 0;
}
Output:
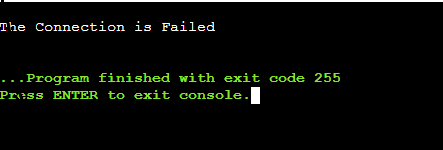